newInstance() Method In Java
newInstance() method
In Java, newInstance() method of Class “class” and Constructor class creates a new instance of the class. The newInstance() method of Class “class” can call zero-argument constructor but newInstance() method of Constructor class can call any number of arguments. Thus, Constructor class is preferred over Class “class”.
Syntax
public T newInstance()throws InstantiationException,IllegalAccessException
In this, T is the generic version. We can think it like Object class.
Let's see an example of newInstance() method.
Code
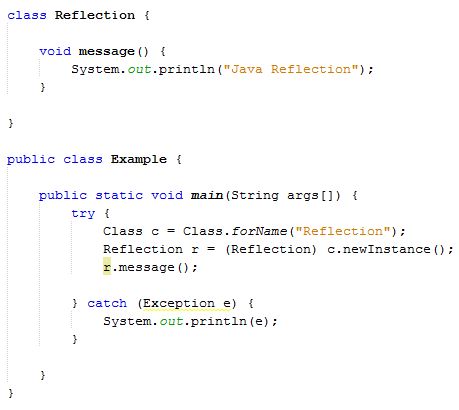
- class Reflection {
- void message() {
- System.out.println("Java Reflection");
- }
- }
- public class Example {
- public static void main(String args[]) {
- try {
- Class c = Class.forName("Reflection");
- Reflection r = (Reflection) c.newInstance();
- r.message();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
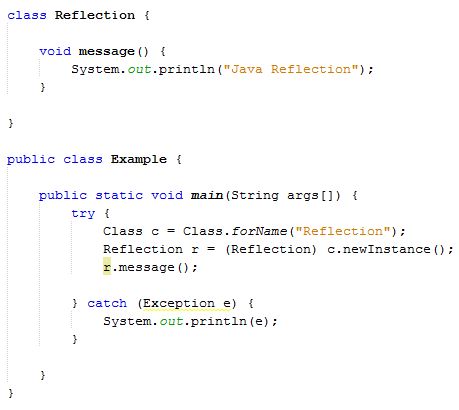
Summary
Thus, we learnt that Java newInstance() method of Class “class” and Constructor class creates a new instance of the class and also learnt how we can use it in Java.