Optional Class In Java
Optional Class
Optional class is used to contain not-null objects. Its object is used to show null with absent value. This class has many methods to make easy code to handle values as ‘available’ or ‘not available’ in its place of checking null values. It is introduced in Java 8 and is like to whatOptional is in Guava.
This Optional class extends methods from the java.lang.Object class.
Class Declaration
public final class Optional<T>
extends Object
Class Methods
static <T> Optional<T> empty()
This method is used to return an empty Optional object.
boolean equals(Object obj)
This method is used to Indicate whether some other object is "equal to" the Optional.
Optional<T> filter(Predicate<? super <T> predicate)
If a value is present and the value matches a given predicate, then this method is used to return an Optional describing the value otherwise returns an empty Optional.
<U> Optional<U> flatMap(Function<? super T,Optional<U>> mapper)
If a value is present, this method applies the provided Optional-bearing mapping function to it and returns the result, otherwise returns an empty Optional.
T get()
If a value is present in the Optional and returns the value otherwise throws NoSuchElementException.
int hashCode()
This method is used to return the hash code value of the present value, if any or 0 if no value is present.
void ifPresent(Consumer<? super T> consumer)
If a value is present, this method invokes the particular consumer with the value.
boolean isPresent()
This method is used to return true if there is a value present otherwise false.
<U>Optional<U> map(Function<? super T,? extends U> mapper)
If a value is present, applies the provided mapping function to it and if the result is non-null, returns an Optional describing the result.
static <T> Optional<T> of(T value)
This method is used to return an Optional with the particular present non-null value.
static <T> Optional<T> ofNullable(T value)
This method is used to return an Optional describing the particular value, if non-null otherwise returns an empty Optional.
T orElse(T other)
This method is used to return the value if present otherwise returns other.
T orElseGet(Supplier<? extends T> other)
This method is used to return the value if present otherwise calls other and returns the result of that invocation.
<X extends Throwable> T orElseThrow(Supplier<? extends X> exceptionSupplier)
This method is used to return the contained value otherwise throws an exception to be created by the provided supplier.
String toString()
This method is used to return a non-empty string representation of the Optional suitable for debugging.
Let’s see an example of Optional class.
Code
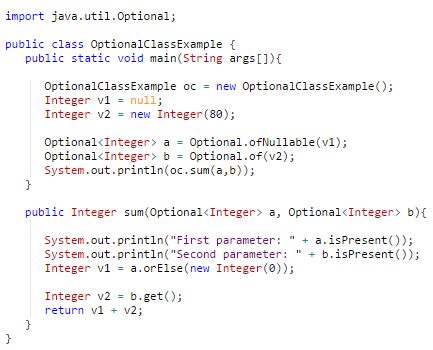
- import java.util.Optional;
- public class OptionalClassExample {
- public static void main(String args[]) {
- OptionalClassExample oc = new OptionalClassExample();
- Integer v1 = null;
- Integer v2 = new Integer(80);
- //Optional.ofNullable - allows passed parameter to be null.
- Optional < Integer > a = Optional.ofNullable(v1);
- //Optional.of - throws NullPointerException if passed parameter is null
- Optional < Integer > b = Optional.of(v2);
- System.out.println(oc.sum(a, b));
- }
- public Integer sum(Optional < Integer > a, Optional < Integer > b) {
- //Optional.isPresent - checks the value is present or not
- System.out.println("First parameter: " + a.isPresent());
- System.out.println("Second parameter: " + b.isPresent());
- //Optional.orElse - returns the value if present otherwise returns
- //the default value passed.
- Integer v1 = a.orElse(new Integer(0));
- //Optional.get - gets the value, value should be present
- Integer v2 = b.get();
- return v1 + v2;
- }
- }
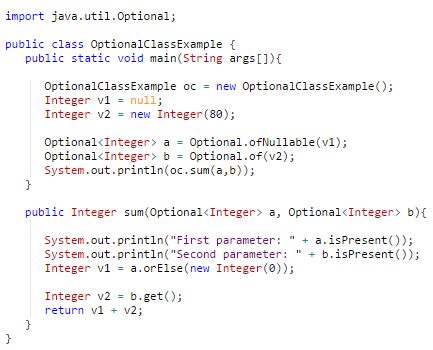
Output
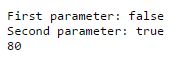
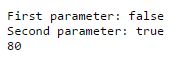
Summary
Thus, we learned that Optional class is used to contain not-null objects. Its object is used to show null with absent value and also learns how to use it in Java.