Throw Exception In Java
Throw Exception
In Java, throw keyword is used to throw an exception explicitly.
In Java, we can throw checked or unchecked exceptions by throw keyword. The throw keyword is used to throw user defined or custom exception.
Syntax
throw exception;
Let’s see an example, given below.
Code
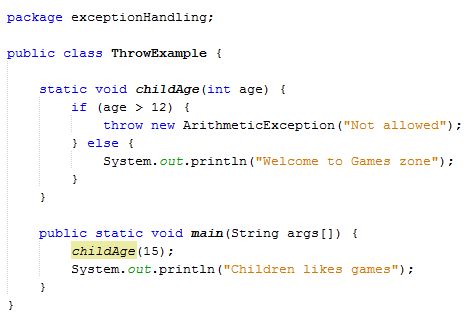
- package exceptionHandling;
- public class ThrowExample {
- static void childAge(int age) {
- if (age > 12) {
- throw new ArithmeticException("Not allowed");
- } else {
- System.out.println("Welcome to Games zone");
- }
- }
- public static void main(String args[]) {
- childAge(15);
- System.out.println("Children likes games");
- }
- }
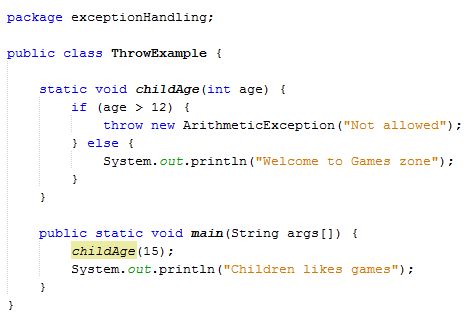
Output


In the example, shown above, the childAge method takes an integer value as a parameter. If the age is greater than 12, it throws the exception otherwise print a message welcome to games zone and children likes games.
Summary
Thus, we learnt throw keyword is used to throw an exception explicitly in Java and also learnt how we can throw the exception.