LinkedHashMap Class In Java
LinkedHashMap Class
In Java, LinkedHashMap class holds the values, based on the key. It implements the map interface and extends HashMap class. It holds the unique elements only, may have one null key and multiple null values. It maintains an insertion order.
Constructors of LinkedHashMap Class
LinkedHashMap( )
This constructor is used to construct a default LinkedHashMap.
LinkedHashMap(Map m)
This constructor is used to initialize the LinkedHashMap with the elements from the given Map class m.
LinkedHashMap(int capacity)
This constructor is used to initialize a LinkedHashMap with the given capacity.
LinkedHashMap(int capacity, float fillRatio)
This constructor is used to initialize both the capacity and fill ratio. The meaning of capacity and fill ratio are the same as far as HashMap is concerned.
LinkedHashMap(int capacity, float fillRatio, boolean Order)
This constructor is used to allow us to specify, whether the elements will be stored in the linked list by insertion order or not. If order is true, an access order is used. If order is false, an insertion order is used.
Methods of LinkedHashMap Class
void clear()
This method is used to remove all the mappings from the map.
boolean containsKey(Object key)
This method is used to return true, if the map maps one or more keys to the specified value.
Object get(Object key)
This method is used to return the value to which the map maps the specified key.
protected boolean removeEldestEntry(Map.Entry eldest)
This method is used to return true, if the map should remove its eldest entry.
Let’s see an example, given below.
Code
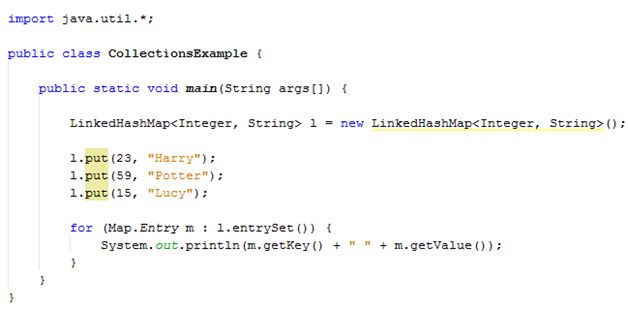
- import java.util.*;
- public class CollectionsExample {
- public static void main(String args[]) {
- LinkedHashMap < Integer, String > l = new LinkedHashMap < Integer, String > ();
- l.put(23, "Harry");
- l.put(59, "Potter");
- l.put(15, "Lucy");
- for (Map.Entry m: l.entrySet()) {
- System.out.println(m.getKey() + " " + m.getValue());
- }
- }
- }
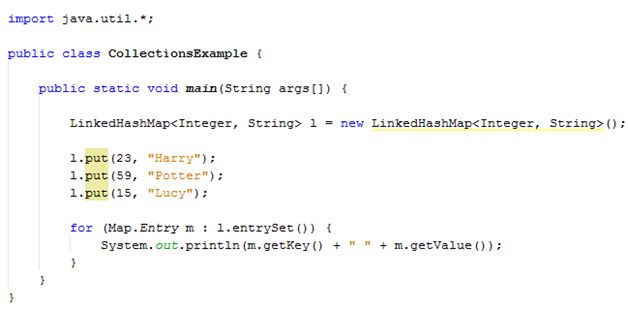
Output
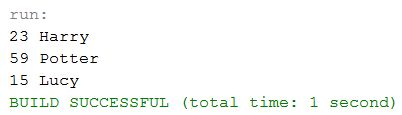
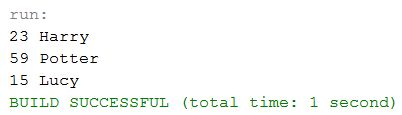
Summary
Thus, we learnt that Java LinkedHashMap class holds the values, based on the key. It implements the map interface and extends HashMap class. It holds the unique elements only and we also learnt how to create it in Java.