How To Connect To The MySQL Database
Java application connects with the MySql database.
There are some important steps to perform database connectivity.
We are using MySql as the database.
- In JDBC, the driver class for the MySql database is com.mysql.jdbc.Driver.
- In JDBC, the connection URL for the MySql database is jdbc:mysql://localhost3306/HumanResources. In it jdbc is the API, MySql is the database and localhost is the server name on which MySql is running, we also use IP address, 3306 is the port number and HumanResources is the database name. We can use any database. In such case, we need to replace the HumanResources with our database name.
- Username for the MySql database is root by default and password is given by the user at the time of installing the MySql database.
First create a table in oracle database.
create database HumanResources;
use HumanResources;
create table student(id int(20),name varchar2(50),age int(3));
Now, let’s see an example to connect Java Application with Oracle database, given below.
Code
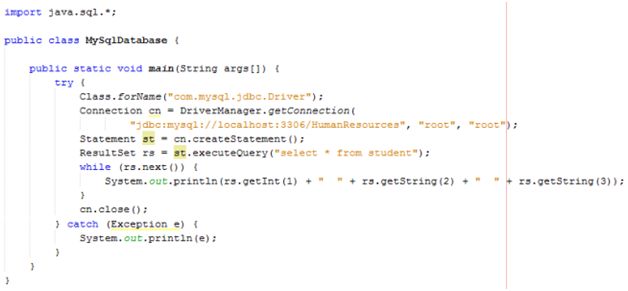
- import java.sql.*;
- public class MySqlDatabase {
- public static void main(String args[]) {
- try {
- Class.forName("com.mysql.jdbc.Driver");
- Connection cn = DriverManager.getConnection(
- "jdbc:mysql://localhost:3306/HumanResources", "root", "root");
- Statement st = cn.createStatement();
- ResultSet rs = st.executeQuery("select * from student");
- while (rs.next()) {
- System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
- }
- cn.close();
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
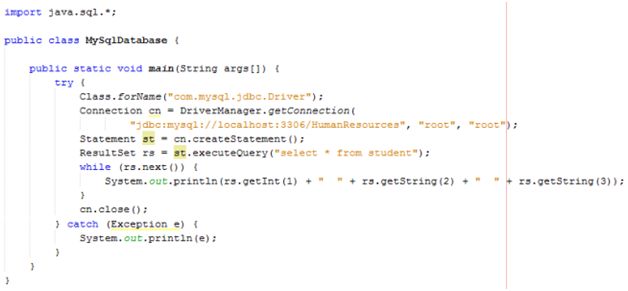
In the above example, HumanResources is the database name, root is the username and password and we are fetching all the records of student table.
Java application connects with the MySql database mysqlconnector.jar file is required to be loaded.
There are two ways to load the jar file, given below.
- Paste the mysqlconnector.jar file in jre/lib/ext folder.
- Set classpath.
- Paste the mysqlconnector.jar file in JRE/lib/ext folder, given below.
Download the mysqlconnector.jar file.
Go to jre/lib/ext folder and paste the jar file at this point. - Set classpath
There are 2 ways to set the classpath, given below.
Temporary set classpath
Permament set classpath
Temporary set classpath
- Open comman prompt.
- Write: C:>set classpath=c:\folder\mysql-connector-java-5.0.8-bin.jar;.;
Permanent set classpath
- Go to environment variable.
- Click new tab. In the variable name, write: classpath and in variable value, paste the path to the mysqlconnector.jar file by adding mysqlconnector.jar;.; as C:\folder\mysql-connector-java-5.0.8-bin.jar;.;
Summary
Thus, we learnt, some important steps to perform MySQL database connectivity and also learn how we can set a classpath in Java.