StringBuffer Class In Java
StringBuffer Class
In Java, we know that the string is immutable. It cannot be changed but a new instance is created. Due to this, we use StringBuffer and StringBuilder class for mutable class.
StringBuffer class is used to create mutable string, which means we can modify the class through StringBuffer class in Java.
StringBuffer class is same as a String class, except it is mutable. It can be changed easily.
In other words, we can say that StringBuffer is a mutable sequence of characters. It is like a string but the contents of the StringBuffer can be modified after creation.
StringBuffer class has a capacity to hold the number of characters. It increases automatically when more contents are added to it.
In Java, StringBuffer class is thread-safe, which means that multiple threads cannot access it at the same time. Thus, it is safe and it will give the result in an order.
Three important constructors of StringBuffer class are,
StringBuffer()
This constructor creates a blank string buffer with the initial capacity of 16.
This constructor creates a blank string buffer with the initial capacity of 16.
StringBuffer(String str)
This constructor creates a string buffer with the specified string.
StringBuffer(int capacity)
This constructor creates a blank string buffer with the specified capacity as length.
This constructor creates a blank string buffer with the specified capacity as length.
There are many important methods of StringBuffer class in Java, which are,
public synchronized StringBuffer append(String s)
This method is used to append the particular string with this string. The append() method is overloaded like append(int), append(float), append(double) etc.
This method is used to append the particular string with this string. The append() method is overloaded like append(int), append(float), append(double) etc.
public synchronized StringBuffer insert(int offset, String s)
This method is used to insert the particular string with this string at the specified position. The insert() method is overloaded like insert(int, char), insert(int, int), insert(int, float) etc.
This method is used to insert the particular string with this string at the specified position. The insert() method is overloaded like insert(int, char), insert(int, int), insert(int, float) etc.
public synchronized StringBuffer replace(int startIndex, int endIndex, String str)
This method is used to replace the string from particular startIndex and endIndex.
This method is used to replace the string from particular startIndex and endIndex.
public synchronized StringBuffer delete(int startIndex, int endIndex)
This method is used to delete the string from the particular startIndex and endIndex.
This method is used to delete the string from the particular startIndex and endIndex.
public synchronized StringBuffer reverse()
This method is used to reverse the string in Java.
This method is used to reverse the string in Java.
public int capacity()
This method is used to return the current capacity of the string.
This method is used to return the current capacity of the string.
public void ensureCapacity(int minimumCapacity)
This method is used to ensure the capacity i.e. at least equal to the given minimum.
This method is used to ensure the capacity i.e. at least equal to the given minimum.
public char charAt(int index)
This method is used to return the character at the particular position.
This method is used to return the character at the particular position.
public int length()
This method is used to return the length of the string (means the total number of characters).
public String substring(int beginIndex)
This method is used to return the substring from the particular beginIndex.
This method is used to return the substring from the particular beginIndex.
public String substring(int beginIndex, int endIndex)
This method is used to return the substring from the particular beginIndex and endIndex.
This method is used to return the substring from the particular beginIndex and endIndex.
Let's see an examples of methods of StringBuffer class.
StringBuffer append() method
The append() method is used to concatenate the particular argument with this string.
Let’s see an example, given below.
Code
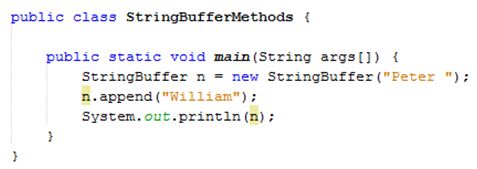
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer n = new StringBuffer("Peter ");
- n.append("William");
- System.out.println(n);
- }
- }
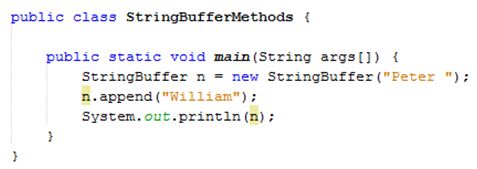
Output


In the example, mentioned above, the original string is changed and gives the output.
StringBuffer insert() method
The insert() method is used to insert the particular string with this string at the specified position.
Let’s see an example, given below.
Code
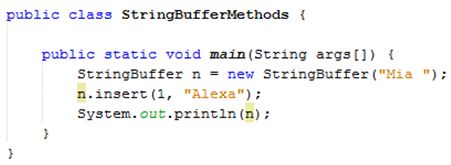
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer n = new StringBuffer("Mia ");
- n.insert(1, "Alexa");
- System.out.println(n);
- }
- }
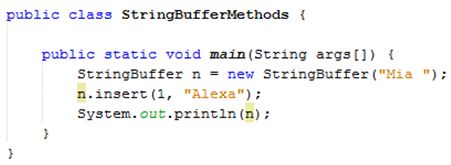
Output


In the example, shown above, the original string is changed and gives the output.
StringBuffer replace() method
The replace() method is used to replace the particular string from the specified beginIndex and endIndex.
Let’s see an example, given below.
Code
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer n = new StringBuffer("Lily ");
- StringBuffer n1 = new StringBuffer("Lily ");
- n.replace(1, 2, "Jaxon");
- n1.replace(1, 4, "Jaxon");
- System.out.println(n);
- System.out.println(n1);
- }
- }
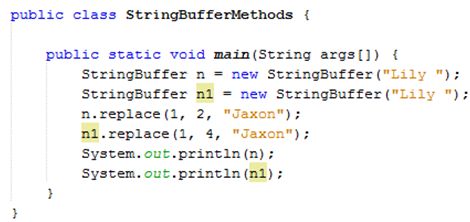
Output
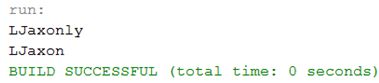
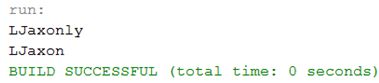
StringBuffer delete() method
The delete() method is used to delete the string from the particular beginIndex to endIndex.
Let’s see an example, given below.
Code
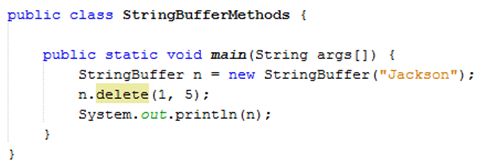
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer n = new StringBuffer("Jackson");
- n.delete(1, 5);
- System.out.println(n);
- }
- }
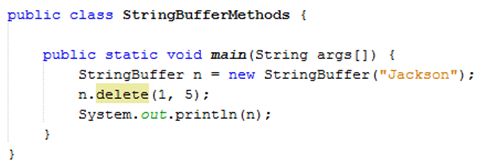
Output


StringBuffer reverse() method
The reverse() method is used to reverse the current string.
Let’s see an example, given below.
Code
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer name = new StringBuffer("David");
- name.reverse();
- System.out.println(name);
- }
- }
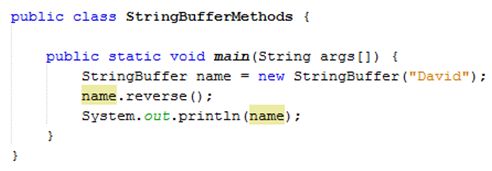
Output


StringBuffer capacity() method
The capacity() method is used to return the current capacity of the buffer.
The default capacity of the buffer is 16 and If the number of character increases from its current capacity, it increases the capacity by (old capacity*2) +2.
Let’s see an example, given below.
Code
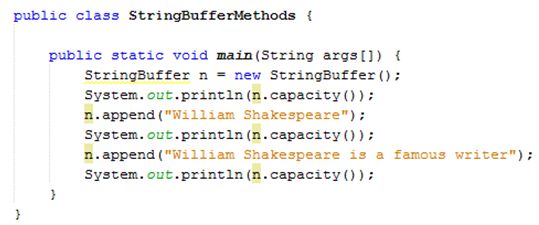
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer n = new StringBuffer();
- System.out.println(n.capacity());
- n.append("William Shakespeare");
- System.out.println(n.capacity());
- n.append("William Shakespeare is a famous writer");
- System.out.println(n.capacity());
- }
- }
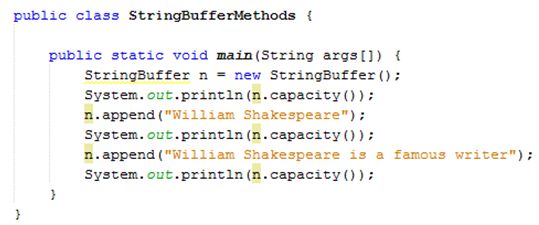
Output
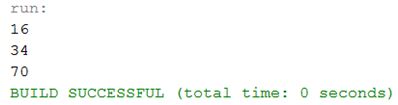
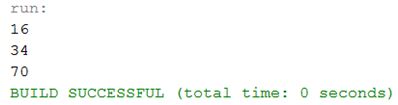
StringBuffer ensureCapacity() method
The ensureCapacity() method is used to ensure that the given capacity is the minimum to the current capacity and if it is greater than the current capacity, it increases the capacity by (old capacity*2) +2.
Let’s see an example, given below.
Code
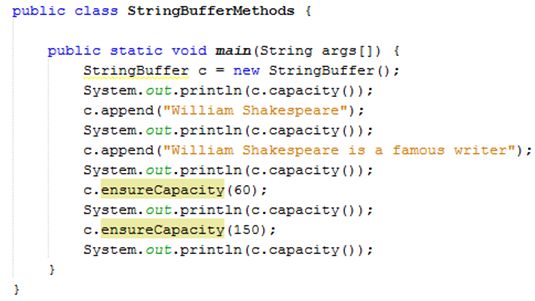
- public class StringBufferMethods {
- public static void main(String args[]) {
- StringBuffer c = new StringBuffer();
- System.out.println(c.capacity());
- c.append("William Shakespeare");
- System.out.println(c.capacity());
- c.append("William Shakespeare is a famous writer");
- System.out.println(c.capacity());
- c.ensureCapacity(60);
- System.out.println(c.capacity());
- c.ensureCapacity(150);
- System.out.println(c.capacity());
- }
- }
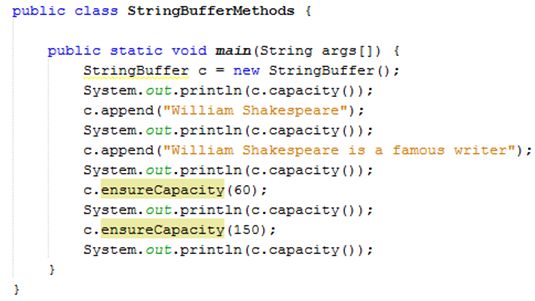
Output
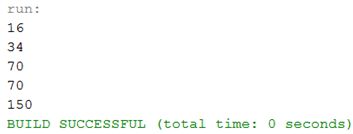
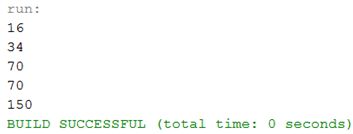
Summary
Thus, we learnt that StringBuffer class is same as String class, except it is mutable. It can be changed easily and also learnt its important methods in Java.