Shutdown Hook In Java
Shutdown Hook
Shutdown Hook is used to perform cleanup resource or save the state, when JVM shuts down normally. Performing clean resource such as closing log file, sending some alerts etc. Thus, if we want to execute some code before JVM shuts down, we need to use Shutdown Hook.
The JVM shuts down when the conditions, given below, exists.
- Press Ctrl+C on the command prompt
- System.exit(int) method is called
- User logoff
- User shutdown
- Completion of the thread executions
The addShutdownHook(Thread hook) method
public void addShutdownHook(Thread hook): This method of Runtime class is used to register the thread with the Virtual Machine.
Runtime class object can be obtained by calling the static factory method getRuntime().
Example
Runtime r = Runtime.getRuntime();
(Factory method is used to returns the instance of a class.)
Let’s see an example, given below.
Code
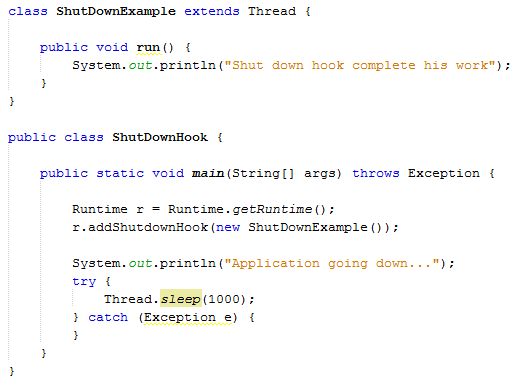
- class ShutDownExample extends Thread {
- public void run() {
- System.out.println("Shut down hook complete his work");
- }
- }
- public class ShutDownHook {
- public static void main(String[] args) throws Exception {
- Runtime r = Runtime.getRuntime();
- r.addShutdownHook(new ShutDownExample());
- System.out.println("Application going down...");
- try {
- Thread.sleep(1000);
- } catch (Exception e) {}
- }
- }
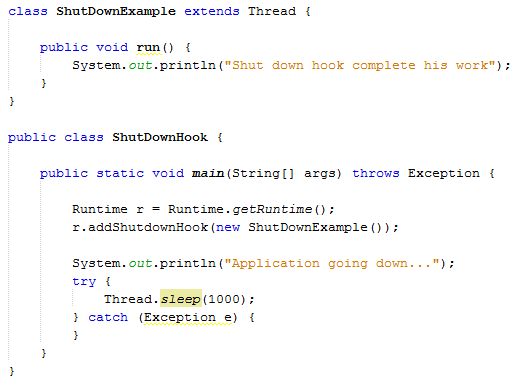
Output
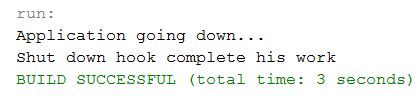
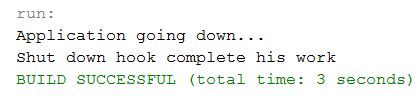
In the example, mentioned above, we create an object of runtime class, using the getRuntime() method, followed by the method, which returns the runtime object connected with the current Java Application. Now, we can attach ShutDownThread to Shutdown Hook, using addShutdownHook() method.
The Shutdownhook can stop by calling the halt(int) method of Runtime class.
Now, let’s see another example- Shutdownhook with annonymous class, given below.
Code
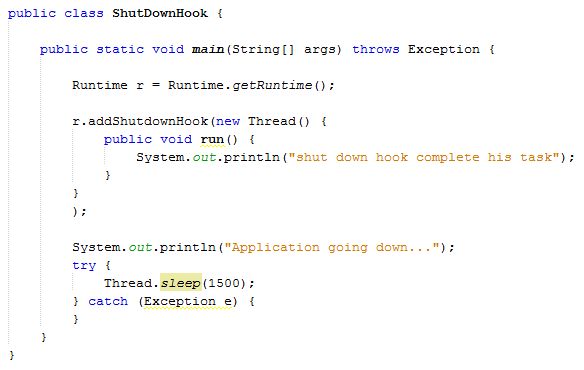
- public class ShutDownHook {
- public static void main(String[] args) throws Exception {
- Runtime r = Runtime.getRuntime();
- r.addShutdownHook(new Thread() {
- public void run() {
- System.out.println("shut down hook complete his task");
- }
- });
- System.out.println("Application going down...");
- try {
- Thread.sleep(1500);
- } catch (Exception e) {}
- }
- }
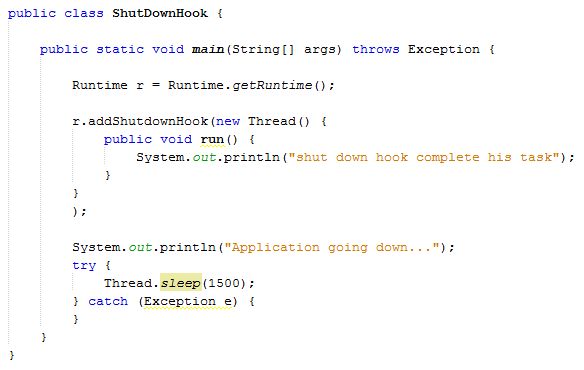
Output
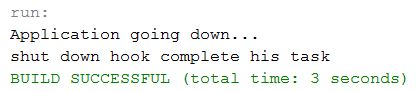
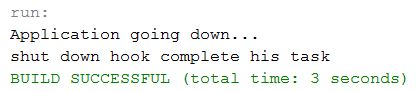
Summary
Thus, we learnt, Shutdown Hook is used to perform the cleanup resource or save the state, when JVM shuts down and also learnt how to create it in Java.