URL In Java
URL
URL stands for “Uniform Resource Locator”. URL class shows an URL that point to a resource on the World Wide Web in Java.
For example
"http://www.google.com/images"
A URL holds many types of information’s, such as:
- Protocol
- Server name or IP Address
- Port Number
- File Name or directory name
Methods of URL class
The java.net.URL class provides many methods.
public String getProtocol()
This method is used to return the protocol of the URL.
public String getHost()
This method is used to return the host name of the URL.
public String getPort()
This method is used to return the port number of the URL.
public String getFile()
This method is used to return the file name of the URL.
public URLConnection openConnection()
This method is used to return the instance of URLConnection i.e. an associated with this URL.
Let’s see an example of URL class, given below.
Code
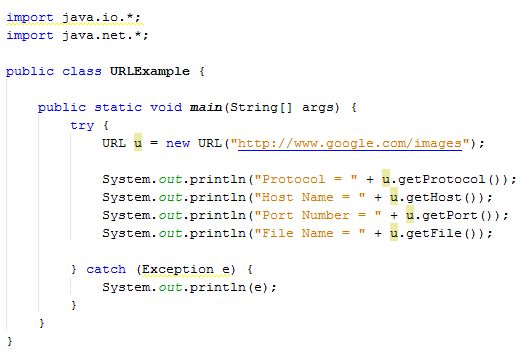
- import java.io.*;
- import java.net.*;
- public class URLExample {
- public static void main(String[] args) {
- try {
- URL u = new URL("http://www.google.com/images");
- System.out.println("Protocol = " + u.getProtocol());
- System.out.println("Host Name = " + u.getHost());
- System.out.println("Port Number = " + u.getPort());
- System.out.println("File Name = " + u.getFile());
- } catch (Exception e) {
- System.out.println(e);
- }
- }
- }
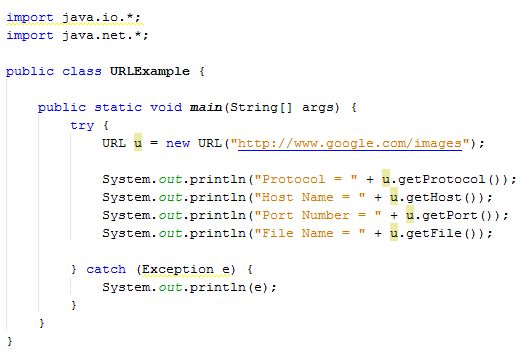
Output
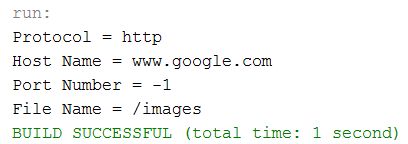
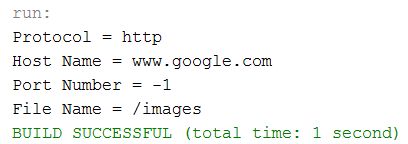
Summary
Thus, we learnt that Java URL stands for “Uniform Resource Locator”. URL class shows a URL that points to a resource on the World Wide Web in Java and also learnt its important methods in Java.