Objective:
Core Objective of this article is to give step by step explnation of , How to update or refresh a DataGrid dynamically in SilverLight ?
a. List which is to be bind must be ObservableCollection
b. Item of list of business class must implement INotifyPropertyChanged interface.
This article is explaning how DataGrid would get refresh dynamically whenever value in list would be changed.
Step 1 :
Create a SilverLight Application.
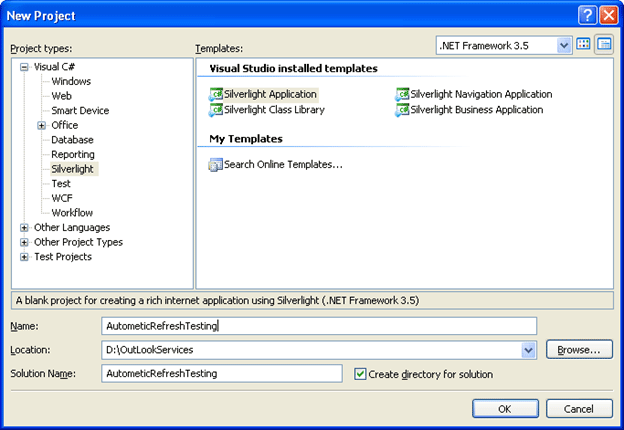
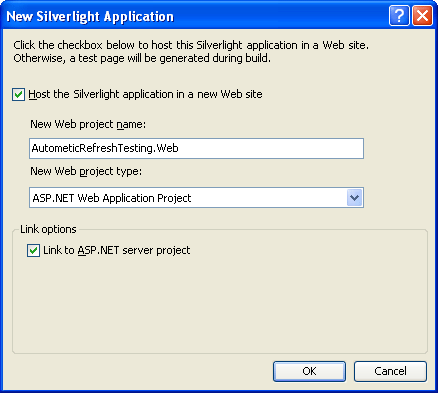
Step 2 :
a. Add one more new class. Give it any name. Name of that is EventBase. Purpose of this class is to act as a base class for which will implement INotifyPropertyChanged
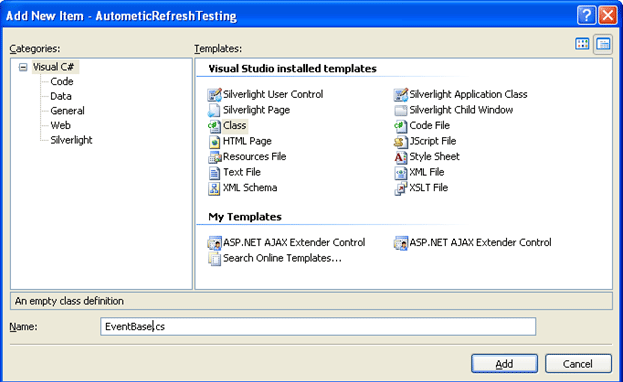
b. Add namepsace
using System.ComponentModel;
c. Implement interface INotifyPropertyChanged
d. Make class as abstarct class.
e. Declare an public event of type PropertyChangedEventHandler
The entire code will look like ,
EventBase.cs
using System.ComponentModel;
namespace AutometicRefreshTesting
{
public abstract class EventBase : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected void PropertyChangedHandler(string propertyName)
{
var handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
Step 2 :
Add a new class in Silver Light application.
Name it as Player.cs. This is Business class. This class will extend EventBase class. In property, we are calling PropertyChangedHandler("") method of EventBase class to create a notify whenever value in property being changed.
set
{
playerId = value;
PropertyChangedHandler("PlayerID");
}
Player.cs
using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace AutometicRefreshTesting
{
public class Player : EventBase
{
private int playerId;
public int PlayerID
{
get
{
return playerId;
}
set
{
playerId = value;
PropertyChangedHandler("PlayerID");
}
}
private String playerName;
public String PlayerName
{
get
{
return playerName;
}
set
{
playerName = value;
PropertyChangedHandler("playerName");
}
}
private String countryName;
public String CountryName
{
get
{
return countryName;
}
set
{
countryName = value;
PropertyChangedHandler("CountryName");
}
}
}
}
Step 3:
Add a Button and DataGrid on XAML of page.
-
DataGrid could be dragged and drop from ToolBox for SilverLight.
-
Here Grid is divided into two columns. One column is containing Button and other is containing DataGrid.
MainPage.Xaml
<UserControl xmlns:data="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data"
x:Class="AutometicRefreshTesting.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto"/>
<ColumnDefinition Width="Auto" MinWidth="337"/>
</Grid.ColumnDefinitions>
<Grid.RowDefinitions>
</Grid.RowDefinitions>
<Button x:Name="btnAdd" Content="Add Player to Static List" Width="150" Height="50" Grid.Row="0" Grid.Column="0" Click="btnAdd_Click" />
<data:DataGrid x:Name="dataList" Grid.Row="0" Grid.Column="1">
</data:DataGrid>
</Grid>
</UserControl>
Step 4:
For Dynamic updating or refresh of Data Grid, the List which is to be bind with Data Grid must be a ObservableCollection list. What I am doing here is that adds record on button click event to the list and dynamically refresh the DataGrid.
MainPage.Xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Collections.ObjectModel;
namespace AutometicRefreshTesting
{
public partial class MainPage : UserControl
{
ObservableCollection<Player> playerList;
static int i = 2;
public MainPage()
{
InitializeComponent();
playerList = new ObservableCollection<Player>();
playerList.Add(new Player(){PlayerID=1,PlayerName="MS Dhoni",CountryName="India"});
this.Loaded += new RoutedEventHandler(MainPage_Loaded);
}
void MainPage_Loaded(object sender, RoutedEventArgs e)
{
dataList.ItemsSource = playerList;
}
private void btnAdd_Click(object sender, RoutedEventArgs e)
{
Player obj = new Player() { PlayerID = i, PlayerName = "Sachin Tendulkar ", CountryName = "India" };
playerList.Add(obj);
dataList.ItemsSource = playerList;
i= i+1 ;
}
}
}
Step 5:
Output :
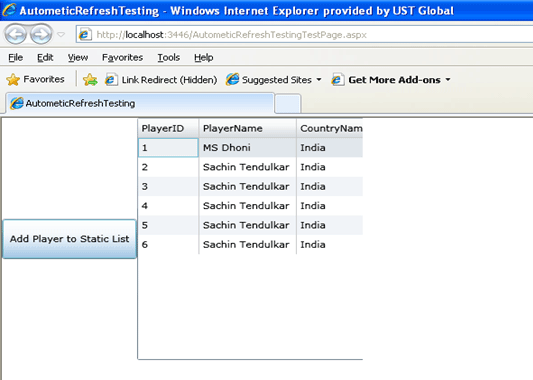
Conclusion
Here I mainly showed how to automatically update the DataGrid when Item source is changing. Please download the zip file and go through the source code for better understanding.
Happy Coding :