In my previous post, I talked about Default End Point in WCF 4. If we closely look at the output generated by default, WCF maps protocol to binding as below.
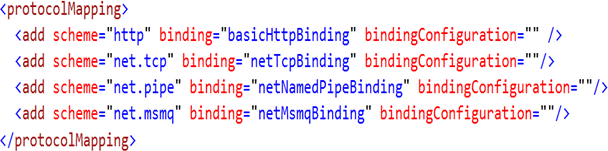
So if we are not configuring any EndPoint, then for http type base address in default EndPoint created by WCF will have basicHttpBinding.
If we see the above mapping, by default http type is mapped to basicHttpBinding, net.tcp type is mapped to netTcpBinding and so on.
If we want to change this, we can change this default mapping

Now when we do the above change in the Web.Config file then when we do not define any EndPoint then for HTTP type base address, WCF will create the EndPoint with wsHttpBinding.
So to understand more, what I am talking about
1. Create a WCF Service application
2. Create a Service contract as below
[ServiceContract]
public interface IService1
{
[OperationContract]
string GetData();
}
3. Implement the Service as below
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.Text;
namespace WcfService1
{
public class Service1 : IService1
{
public string GetData()
{
return "Hello From Iservice1 ";
}
}
}
4. Now we will host this service in a console application. Create a new console application project. Add a reference to a WCF service application project and also add reference to a System.serviceModel in the console application project.
5. Right click on the console project and add app.config file to console project. Write the configuration below in App.Config.
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.serviceModel>
<protocolMapping >
<add scheme ="http" binding ="wsHttpBinding" bindingConfiguration ="" />
</protocolMapping>
</system.serviceModel>
</configuration>
6. Now write the code below to fetch the default Endpoint created by WCF
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ServiceModel;
using System.ServiceModel.Description;
using WcfService1;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
ServiceHost host = new ServiceHost(typeof(WcfService1.Service1),
new Uri("http://localhost:8080/service"));
host.Open();
foreach (ServiceEndpoint se in host.Description.Endpoints)
{
Console.WriteLine("Address: " + se.Address.ToString() +
" Binding: " + se.Binding.Name +
" Contract :"+ se.Contract.Name);
}
Console.ReadKey(true);
}
}
}
And output we would get is
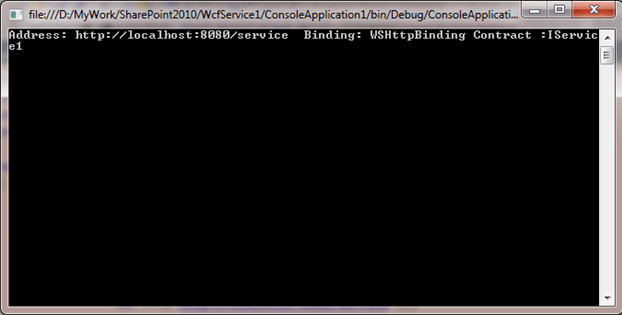
So we can see how we modified the default mapping using Protocol mapping in System.ServiceModel