Html Helper:
-
To add content to VIEW of Asp.net MVC application, Html Helper is being used.
-
This is nothing but a method which returns string.
-
Html Helper could we used to generate standard HTML elements like Text Box, Label etc.
-
All Html helper are called on the Html property of view. For example to render a Text Box we call Html.TextBox() method.
-
Using Html helper method is optional. Its purpose is to reduce scripting code.
The ASP.NET MVC framework includes the following set of standard HTML Helpers (this is not a complete list):
-
Html.ActionLink()
-
Html.BeginForm()
-
Html.CheckBox()
-
Html.DropDownList()
-
Html.EndForm()
-
Html.Hidden()
-
Html.ListBox()
-
Html.Password()
-
Html.RadioButton()
-
Html.TextArea()
-
Html.TextBox()
Creating Html Helper method
Custom Html Helper method could we created in two ways
-
Using static method.
-
By extension method.
Using static method:
The easiest way to create custom Html helper method is create static method and return a string. Here we are going to create a Html helper method, which will render a label element of Html.
Step 1:
Add a new folder called Helpers in Asp.Net MVC application.
Step 2:
Add a class in newly created Helpers folder. Right click at folder and then add class named LableHelper.cs
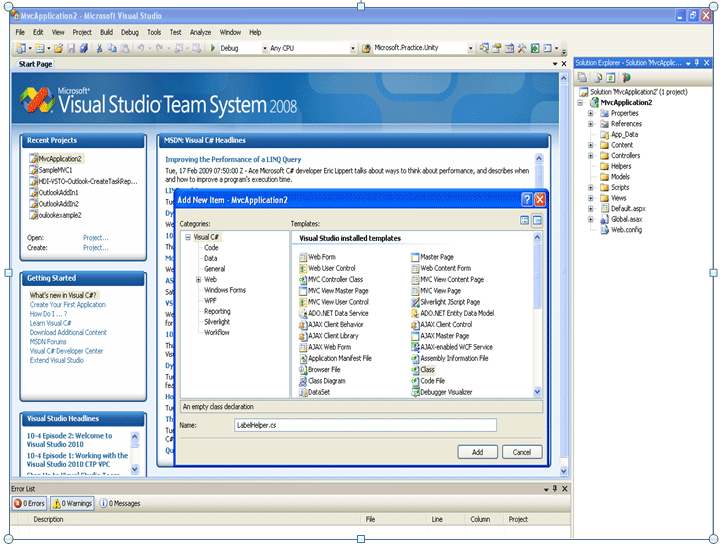
Step 3:
Now add static method in this class called Label. This method will return string and will take two string parameters named target and text.
Code will be like below
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace MvcApplication2.Helpers
{
public class LabelHelper
{
public static string Label(string target, string text)
{
return String.Format("<label for= '{0}'>{1}</label>",target,text);
}
}
}
Step 4:
Now, we are going to use Label Html helper in view or aspx page.
View\Home\Index.aspx
<%@ Page Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<%@ Import Namespace="MvcApplication2.Helpers" %>
<asp:Content ID="indexHead" ContentPlaceHolderID="head" runat="server">
<title>Home Page</title>
</asp:Content>
<asp:Content ID="indexContent" ContentPlaceHolderID="MainContent" runat="server">
<h2><%= Html.Encode(ViewData["Message"]) %></h2>
<p>
To learn more about ASP.NET MVC visit <a href="http://asp.net/mvc" title="ASP.NET MVC Website">http://asp.net/mvc</a>.
</p>
<div>
<%using(Html.BeginForm())
{ %>
<%=LabelHelper.Label("firstName","Firstname") %>
<% } %>
</div>
</asp:Content>
In above code couples of things are worth noticing.
-
We have included Namespace for Html Handler method of Label.
-
Html.BeginForm is inside using , such that after rendering form will get closed.
-
LabelHelper.Label is preceded by <%=.
As Extension method:
public static class LabelExtensions
{
public static string Label(this HtmlHelper helper, string target, string text)
{
return String.Format("<label for='{0}'>{1}</label>", target, text);
}
}
In above code fact noticing are
-
First parameter to Html Helper method is this . In case of extension method always first parameter is class on which we are extending the method.
-
Extended method enables us to add new method in existing class.
-
Here class is static class. We must define Extension method with static class.
-
Extension method will be read be Intellisense like other method of the class.