Objective:
-
How to create REST service for JSON request and response format.
-
How to create SilverLight Client, which will consume a REST service on JSON data format.
-
How to POST data in JSON format from SilverLight client.
Pre Requisite
Reader should have basic knowledge of REST services. For more on REST read my other articles.
Working explanation
Here, I am trying to insert data in static list and fetch it back at SilverLight client.
For Solution follow below steps:
Step 1:
Create new WCF Service Application
File -> New -> Project -> Web -> WCF Service Application
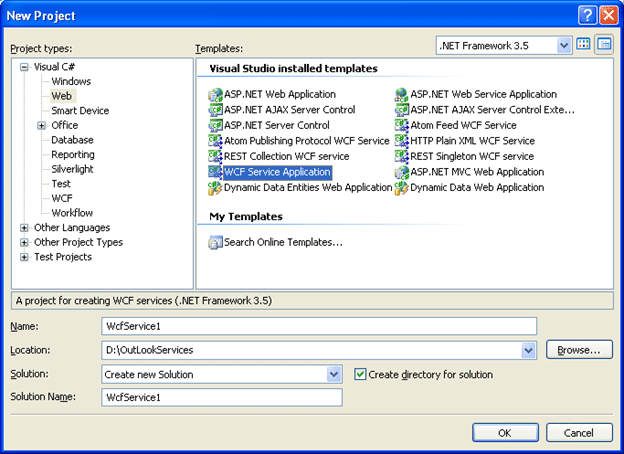
Solution explorer would like more or less,
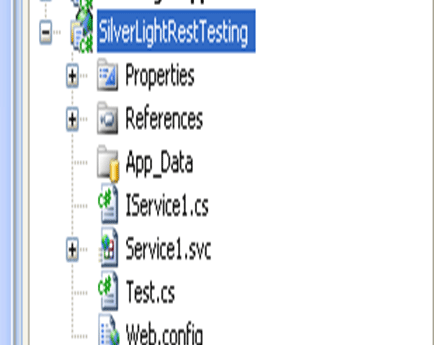
Step 2:
Remove all the default code in service1.svc and IService1.cs.
Paste the below code there in IService1.cs .
Note :
-
If you have changed the name of service interface then take care of that. If you have changed IService1 to ITest then update code in ITest.cs. if you have changed name of Service1.cs then update code in changed class.
-
In this sample, I am adding object of Test class in a static list and fetching it back. If you want to perform pure Data base operation (CRUD). Just replace Insert code and get Code with your database operation code.
-
Add reference of System.ServiceModel.Web to IService1.cs.
-
Now what is Test class here? And where it should be declared and created. So just right click on service project and add a class. Give name of the class Test and paste below code over there.
Test.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace SilverLightRestTesting
{
public class Test
{
public int Marks { get; set; }
public String Name { get; set; }
}
}
IService1.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
using System.ServiceModel.Web;
namespace SilverLightRestTesting
{
// NOTE: If you change the interface name "IService1" here, you must also update the reference to "IService1" in Web.config.
[ServiceContract]
public interface IService1
{
[OperationContract] [WebGet(UriTemplate="/Data",BodyStyle=WebMessageBodyStyle.Bare,RequestFormat=WebMessageFormat.Json,ResponseFormat=WebMessageFormat.Json)]
List<Test> GetTest();
[OperationContract]
[WebInvoke(UriTemplate = "/Data", BodyStyle = WebMessageBodyStyle.Bare, RequestFormat = WebMessageFormat.Json, ResponseFormat = WebMessageFormat.Json)]
void InsertTest(Test t);
}
}
Service1.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.Text;
namespace SilverLightRestTesting
{
// NOTE: If you change the class name "Service1" here, you must also update the reference to "Service1" in Web.config and in the associated .svc file.
public class Service1 : IService1
{
static List<Test> testList = new List<Test>();
public List<Test> GetTest()
{
return testList;
}
public void InsertTest(Test t)
{
testList.Add(t);
}
}
}
Explanation of code:
[OperationContract]
[WebGet(UriTemplate="/Data",BodyStyle=WebMessageBodyStyle.Bare,RequestFormat=WebMessageFormat.Json,ResponseFormat=WebMessageFormat.Json
]
List<Test> GetTest();
Here, Response format, Request Format all are attributed with message format as JOSN. This service GetTest() will return List<Test> .
[OperationContract]
[WebInvoke(UriTemplate = "/Data", BodyStyle = WebMessageBodyStyle.Bare, RequestFormat = WebMessageFormat.Json, ResponseFormat = WebMessageFormat.Json)]
void InsertTest(Test t);
Here, Response format, Request Format all are attributed with message format as JOSN. This service InsertTest(Test t) will take Test object as input parameter and insert this into a static list.
Step 3:
Open Web.Config file and delete below highlighted code. In other words delete all default bindings and endpoints for existing service model. Delete existing <system.serviceModel>
Step 4:
Right click on Service1.cs and open View markup.
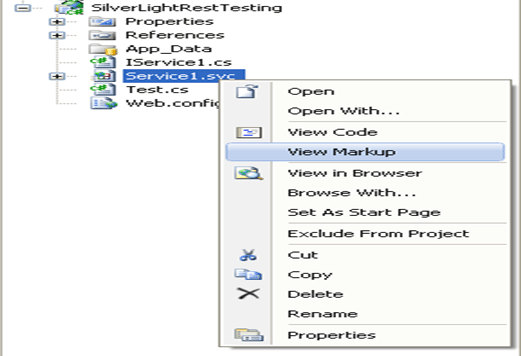
In Markup of RestService.cs , add below code there
Factory="System.ServiceModel.Activation.WebServiceHostFactory"
So after adding code the markup would look like
Markup of RestService.cs
<%@ ServiceHost Language="C#" Debug="true" Service="SilverLightRestTesting.Service1" CodeBehind="Service1.svc.cs" Factory="System.ServiceModel.Activation.WebServiceHostFactory" %>
Step 5:
Hosting the service in IIS.
Right click on Service Project and click on Publish.
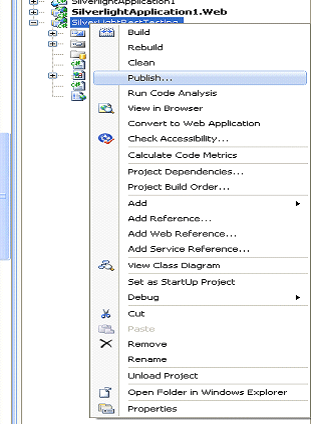
Click on browse button.
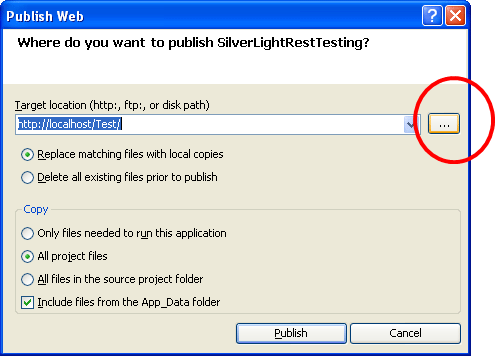
Click on Create New Virtual Directory to create a virtual directory.
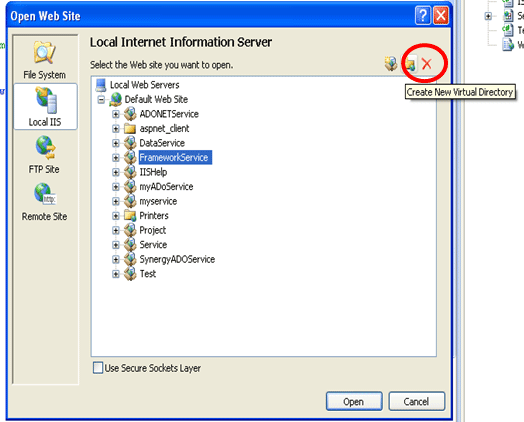
Give a Alias name and browse to the folder where you have created the service.
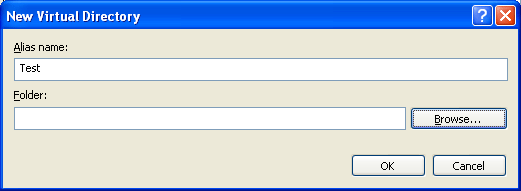
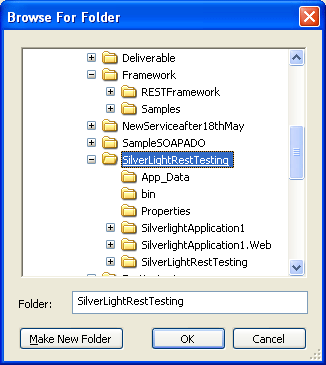
Click on OK
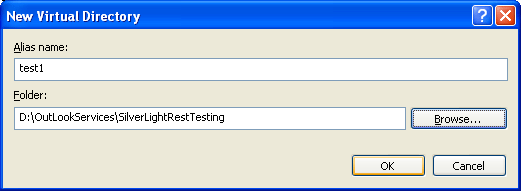
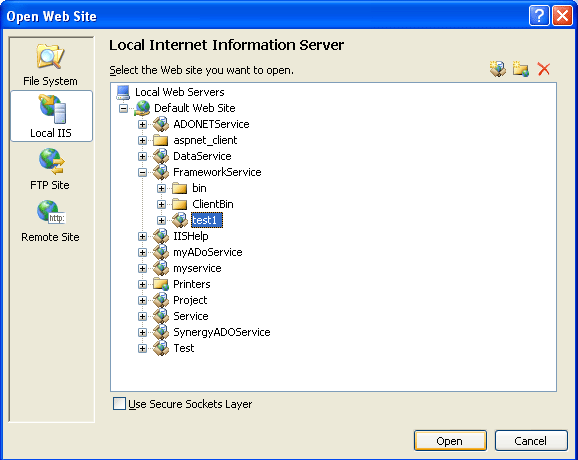
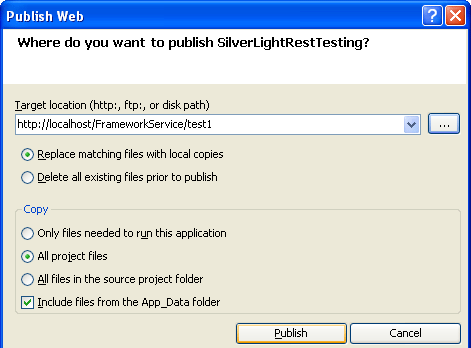
Click ok and publish the REST service in IIS. At bottom of visual studio Publish succeeded message should come.
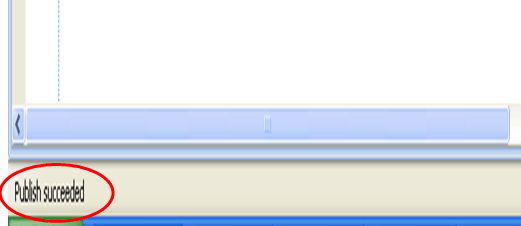
Upto this step REST service has been published in IIS.
Click on Start->Run and type Inetmgr then click ok.
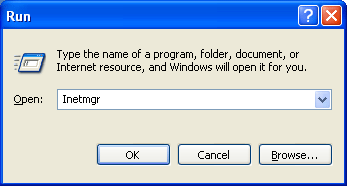
Click on Test website.
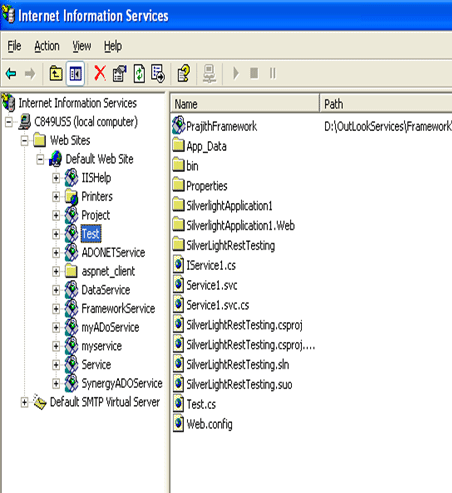
Right click and select Properties.
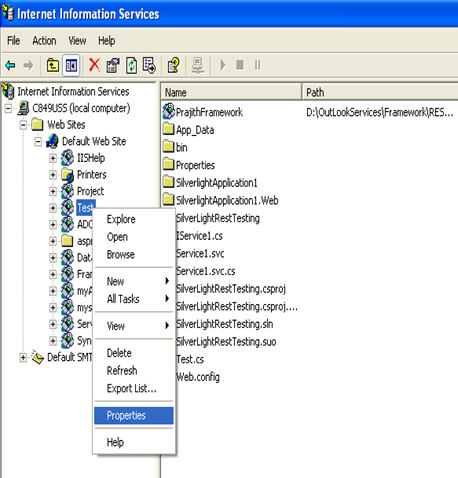
Check Write checkbox and from drop down list select Scripts and Executable
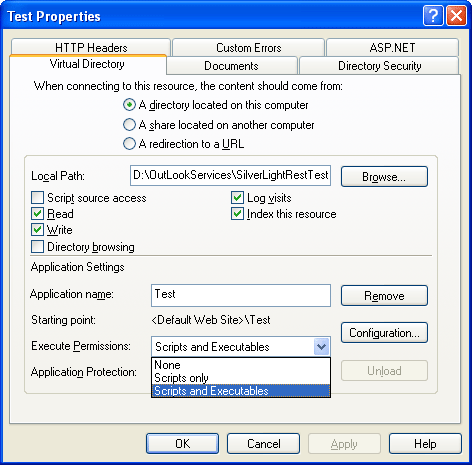
Select Yes for warning message.

Click on Directory Security. Then select Edit
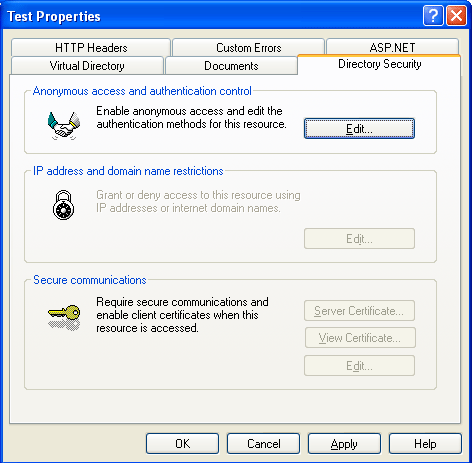
Now select Integrated Windows Authentication. Uncheck Anonymous authentication.
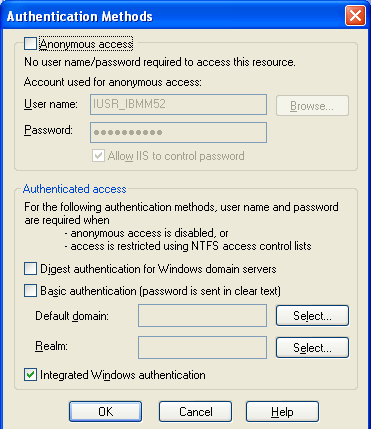
Click on Test then Service1.svc and browse.
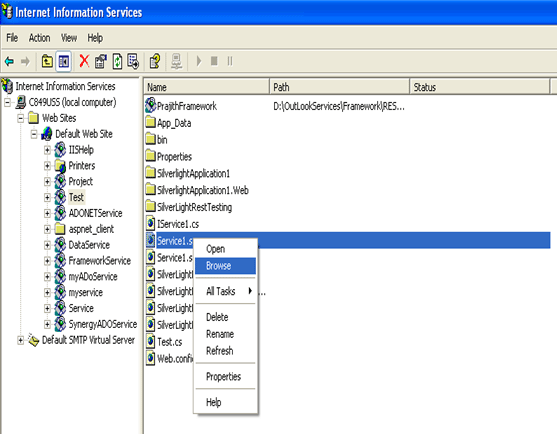
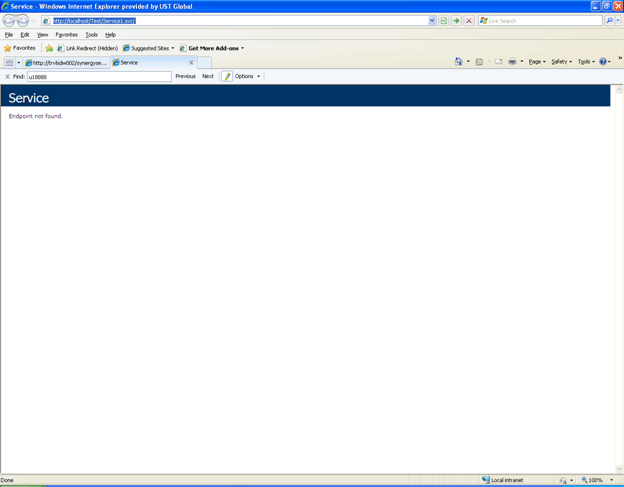
Step 6:
Create a SilverLight application.
-
Create two buttons on SilverLight markup. One for getting the records from the service and other for Insert into the service.
-
Right click on SilverLight application and add the class Test. Because in REST services DataContract are not exposed to the client.
Test.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace SilverLightRestTesting
{
public class Test
{
public int Marks { get; set; }
public String Name { get; set; }
}
}
-
Let us suppose button name is Insert for Insert into Static list of service. Write below code on click event of Insert button.
private void Insert_Click(object sender, RoutedEventArgs e)
{
Test t1 = new Test() { Name = "Civics", Marks = 100 };
DataContractJsonSerializer jsondata = new DataContractJsonSerializer(typeof(Test));
MemoryStream mem = new MemoryStream();
jsondata.WriteObject(mem,t1);
string josnserdata = Encoding.UTF8.GetString(mem.ToArray(), 0, (int)mem.Length);
WebClient cnt = new WebClient();
cnt.UploadStringCompleted += new UploadStringCompletedEventHandler(cnt_UploadStringCompleted);
cnt.Headers["Content-type"] = "application/json";
cnt.Encoding = Encoding.UTF8;
cnt.UploadStringAsync(new Uri(uri), "POST", josnserdata);
}
void cnt_UploadStringCompleted(object sender, UploadStringCompletedEventArgs e)
{
var x = e;
}
4. To get all the records in form of JSON from REST service , let us suppose button name is Display. Then write below code on click event of Display button.
private void display_Click(object sender, RoutedEventArgs e)
{
WebClient cnt = new WebClient();
cnt.DownloadStringCompleted += new DownloadStringCompletedEventHandler(cnt_DownloadStringCompleted);
cnt.DownloadStringAsync(new Uri(uri));
}
void cnt_DownloadStringCompleted(object sender, DownloadStringCompletedEventArgs e)
{
//throw new NotImplementedException();
string str = e.Result;
JsonArray json;
if (JsonArray.Parse(str) as JsonArray == null)
json = new JsonArray { JsonObject.Parse(str) as JsonObject };
else
json = JsonArray.Parse(str) as JsonArray;
var q = from t in json
select new Test
{
Marks = (int)t["Marks"],
Name = (String)t["Name"]
};
List<Test> tr = q.ToList() as List<Test>;
}
Summary:
In this article, I explained about REST service on JSON data format and HTTP POST from SilverLight application. Download Zip file and run the service. You would have better understanding of , what I am trying to in above sample.
Happy Coding..