I have already posted about Deferred Execution here
In this article we will see the internals of Deferred or Lazy Execution of LINQ.
Deferred Execution executes the query only when a loop starts. What I mean here is that, we iterate through the query variable to get the result.
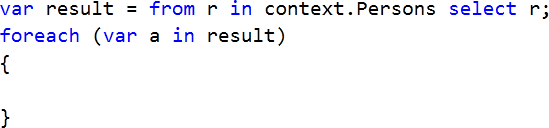
Here the result variable does not contain the actual result. To get the result, we may iterate through the result (query) variable in a loop as many times as we want. We do deferred execution mainly when multiple values are being returned from the query.
Deferred execution can be avoided
- By returning single item from the query
- By converting the query using ToList().
Lazy evolution of deferred execution is executed using the yield operator in C# 3.0
In C# 3.0 it is implemented as below.
public static class Sequence
{
public static IEnumerable<T> Where<T>(this IEnumerable<T> source,
Func<T, bool> predicate)
{
foreach (T element in source)
if (predicate(element))
yield return element;
}
}
The main advantage of lazy evolution is if query is returning large result it will be cached for better optimization. So here execution is delayed until final query is ready to execute.