Objective
In this article, I am going to show, how we could achieve grouping in LINQ to object, Here is the Second Part.
I have created two classes for my explanation purpose. Student class is having details of students and Hostel class is having details of hostel. Both classes are having a properties HostelNumber common. I will be using this property to perform join operations in later articles. Both classes are as below.
Student.cs
namespace LINQtoOBJECT1
{
public class Student
{
public int RollNumber { get; set; }
public string Name { get; set; }
public int Section { get; set; }
public int HostelNumber { get; set; }
}
}
Hostel.cs
namespace LINQtoOBJECT1
{
public class Hostel
{
public int HostelNumber { get; set; }
public int NumberofRooms { get; set; }
}
}
To create collection of students and hostels, I am creating two static functions.
Function to return collection of students
static List<Student> GetStudents()
{
List<Student> students = new List<Student>
{
new Student() { RollNumber = 1,Name ="Alex " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 2,Name ="Jonty " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 3,Name ="Samba " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 4,Name ="Donald " , Section = 3 ,HostelNumber=2 },
new Student() { RollNumber = 5,Name ="Kristen " , Section = 2 ,HostelNumber=1 },
new Student() { RollNumber = 6,Name ="Mark " , Section = 1 ,HostelNumber=2},
new Student() { RollNumber = 7,Name ="Gibbs " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 8,Name ="Peterson " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 9,Name ="collingwood " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 10,Name ="Brian " , Section = 3 ,HostelNumber=2 }
};
return students;
}
Function to return collection of hostels
static List<Hostel> GetHostel()
{
List<Hostel> hostels = new List<Hostel>
{
new Hostel(){HostelNumber=1 ,NumberofRooms = 100},
new Hostel(){HostelNumber= 2 ,NumberofRooms = 200}
};
return hostels;
}
Now have a look on both codes below, one is using LINQ and other is using LOOP to retrieve data from the list and print.
Note: I will be using above two classes and functions for my entire sample below.
Grouping in LINQ
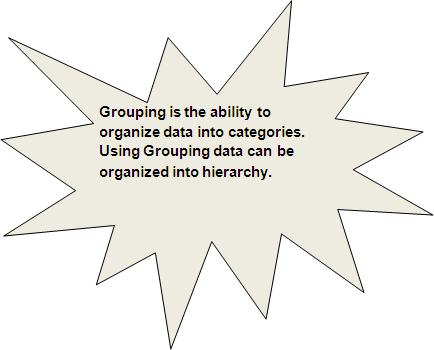
Syntax of Groupby
Var result = FROM selectVariable IN dataSourceorList GROUPBY selectVariable.variable INTO RANGEVARIABLE Select new { };
Grouping by a single property
List<Student> lstStudents = GetStudents();
var lstStudentName = from r in lstStudents
group r by r.HostelNumber into rngStudent
select new
{
HostelID = rngStudent.Key,
Students = rngStudent
};
foreach (var name in lstStudentName)
{
Console.WriteLine(name.HostelID);
foreach (Student s in name.Students)
{
Console.WriteLine(s.RollNumber + s.Name);
}
}
Console.ReadKey();
Explanation
-
I am grouping on property hostel number.
-
rngStudent is range variable in which ; I am putting the intermediate result.
-
I am selecting the result in as anonymous type.
-
While printing the result , first I am printing the key of group by and then retrieving the object in foreach.
Output
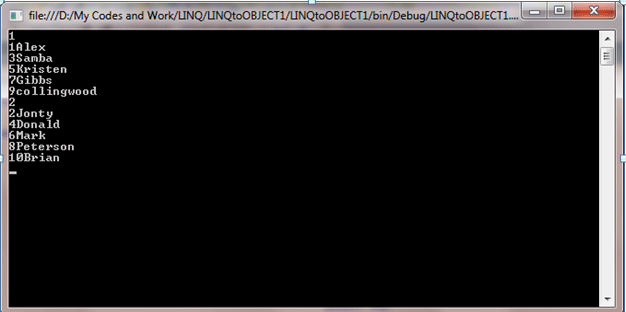
Accessing the Group object
foreach (var name in lstStudentName)
{
Console.WriteLine(name.HostelID);
foreach (Student s in name.Students)
{
Console.WriteLine(s.RollNumber + s.Name);
}
}
If you see the above loop , first I am looping the key for the group by clause and then , I am looping through IEnumerable LINQ object. This object is containing the real result.
Conclusion
In this article, I have discussed GROUPING in LINQ to OBJECT. Thanks for reading.