Objective
In this article, I am going to show, how we could apply LINQ to query non-IEnumerable<T> Collections.
I have created a class for my explanation purpose. Student class is having details of students.
Student.cs
namespace LINQtoOBJECT1
{
public class Student
{
public int RollNumber { get; set; }
public string Name { get; set; }
public int Section { get; set; }
public int HostelNumber { get; set; }
}
}
To create ArrayList of Student, I am creating a static function. This static function will return ArraList of student.
Function to return ArrayList of students.
public static ArrayList GetStudentAsArrayList()
{
ArrayList students = new ArrayList
{
new Student() { RollNumber = 1,Name ="Alex " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 2,Name ="Jonty " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 3,Name ="Samba " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 4,Name ="Donald " , Section = 3 ,HostelNumber=2 },
new Student() { RollNumber = 5,Name ="Kristen " , Section = 2 ,HostelNumber=1 },
new Student() { RollNumber = 6,Name ="Mark " , Section = 1 ,HostelNumber=2},
new Student() { RollNumber = 7,Name ="Gibbs " , Section = 1 ,HostelNumber=1 },
new Student() { RollNumber = 8,Name ="Peterson " , Section = 2 ,HostelNumber=2 },
new Student() { RollNumber = 9,Name ="collingwood " , Section = 3 ,HostelNumber=1 },
new Student() { RollNumber = 10,Name ="Brian " , Section = 3 ,HostelNumber=2 }
};
return students;
}
Now when I tried to query the returned ArrayList using LINQ, I got compile time error. Because ArrayList does not implement interface IQueryable and it is not IEnumerable<T> collection.
Below code gave a compile time error
class Program
{
static void Main(string[] args)
{
ArrayList lstStudents = GetStudentAsArrayList();
var res = from r in lstStudents select r;
Console.ReadKey();
}
}
Error is as shown below,

Above error cause because LINQ by default only query against IEnumerable<T> collections.
So , how to query an ArrayList? Here Range variable comes into action. If I modify the above query as below , I would be able to query LINQ against a non IEnumerable<T> collection.
class Program
{
static void Main(string[] args)
{
ArrayList lstStudents = GetStudentAsArrayList();
var res = from Student r in lstStudents select r;
foreach (Student s in res)
{
Console.WriteLine(s.Name);
}
Console.ReadKey();
}
}
I am using Student as range variable. And we will get output as below .In above code I am using Student the class in ArrayList as range variable.
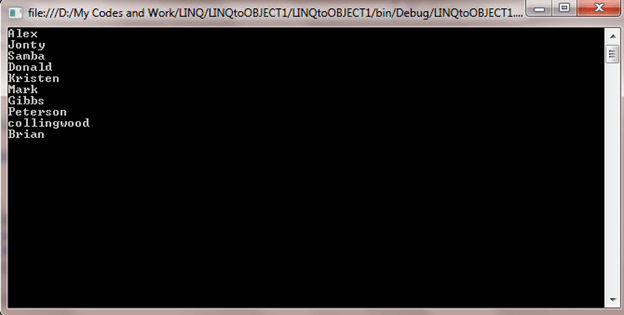
Conclusion
In this article, I discussed how to query against non IEnumerable<T> collection. Thanks for reading.