Objective
This is a very high level article which will explain; how to handle exception in parsing XML using LINQ to XML.
Problem
Let us say, we are parsing a below string using XElement.Parse
String strParse = "<Address><Name>Dhananjay Kumar </Name> ";
This string is not valid formed. But XElement.Parse won't be able to catch the exception. So if we will run the below code
String strParse = "<Address><Name>Dhananjay Kumar </Name> ";
XElement xmlTree = XElement.Parse(strParse);
Console.WriteLine(xmlTree);
We will get the below run time error.
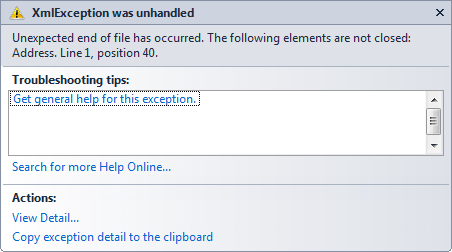
Five Facts
- LINQ to XML is implemented through XMLReader.
- Parse methods on various LINQ to XML class are unable to handle the Exception.
- At time of parsing if input is invalid XML or not formed exception occurs.
- At Exception the underlying XMLReader will throw the Exception.
- High level Exception would be System.Xml.XmlException
How to handle the Exception?
Programmer has to explicitly handle the Exception while parsing. Parsing code need to be put in try catch and Exception must be handled explicitly.
try
{
String strParse = "<Address><Name>Dhananjay Kumar </Name> ";
XElement xmlTree = XElement.Parse(strParse);
Console.WriteLine(xmlTree);
}
catch (System.Xml.XmlException e)
{
Console.WriteLine(e.Message);
}
Output
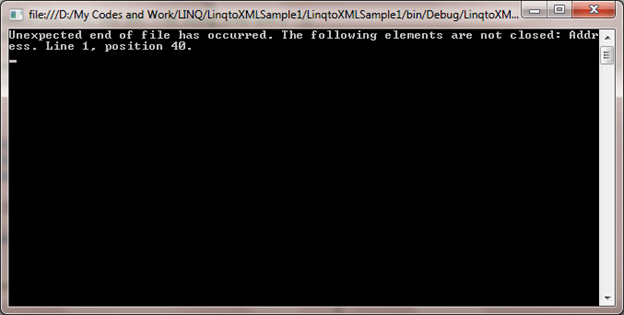
Conclusion
In this article, I showed how to handle exception in parsing XML using LINQ to XML. Thanks for reading.