Objective:
The objective of this article is to give a very basic explanation of how to catch an event on a SharePoint list and deploy them programmatically. I will achieve in this article "User will get error message; whenever any item will get deleted from a particular list ".
What is Event?
Event handling gives developer the ability to catch certain user actions in code and react programmatically.
Steps to handle List in SharePoint
-
Create a class that will extend SPListEventReceiver or SPItemEventReceiver class. These are classes of Microsoft.SharePoint.dll assembly.
-
Sign the class library with strong name.
-
Deploy the class library to GAC
-
Deploy the event receiver assembly.
Step 1: Creating the class
Create a new project of type class library. I am giving name of the project eventhandlersampleclasslibrary. You are free to give any name of your choice. I am extending the SPItemEventReceiver. The purpose of extending this class is that I am going to handle events on a particular item of a list.
Note: I am going to handle the Item Deleting event on a particular list. I will not allow any user to delete any item in that list.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.SharePoint;
namespace eventhandlersampleclasslibrary
{
public class Class1 :SPItemEventReceiver
{
public override void ItemDeleting(SPItemEventProperties properties)
{
//base.ItemDeleting(properties);
string errorMessage = "Please Do not delete ; you are not supposed too ";
properties.ErrorMessage = errorMessage;
properties.Cancel = true;
}
}
}
Step 2: Signing the class name
-
Right click on Project and then Properties.
-
Now click on signing tab.
-
Check the Sign the Assembly checkbox.
-
For selecting the Strong name key file , select New option from drop down
-
Give a strong name. I am giving the name abc here.
-
Make sure Password protection checkbox is unchecked.
-
After this; you would be able to see abc.snk in solution explorer.
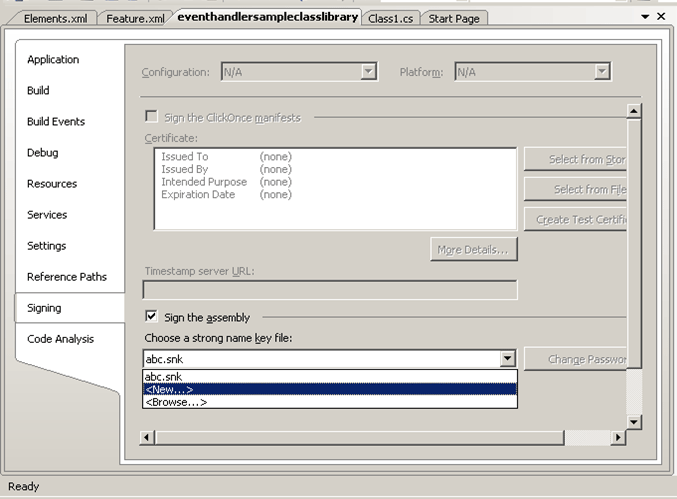
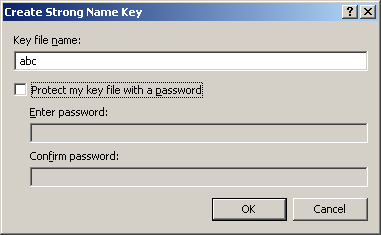
Step 3: Deploying the class library to GAC
-
Open the command prompt
-
Change directory to Program Files\Common Files\ Microsoft Shared\ web server extension\12\Bin
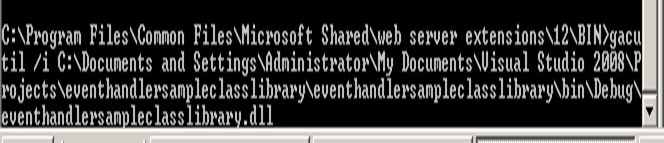
-
Type the command gacutil /I complete path of the dll of class created as eventreceiver class in step1
Gacutil /I [Complte path of dll of class]
The above command will deploy the dll in Gac assembly.
Step 4: Deploy the Event Receiver assembly
This step could be done I either in three ways
-
Through code
-
Through Stsadm command ( Features)
-
Through the content types
I am going to deploy using the program.
-
Either create another project of console type or add class to any existing project.
-
Add the reference of SharePoint dll.
-
Return the Site Collection using SPSite.
-
Return the top level site using SPWeb.
-
Return the list using SPList.
-
Add the event in the list using SPEventRecieverDefinition.
-
Dhan is the name of the list which event I am going to capture.
-
Class name is the name of the class, you created in step1.
-
Assembly is the assembly information of the dll in GAC. To get information about dll in GAC follow below steps.
a. Go to Start -> Run
b. Type Assembly
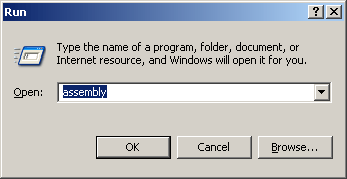
c. Window will open; seek for dll you added.
d. Right click there and go to properties for all the information.
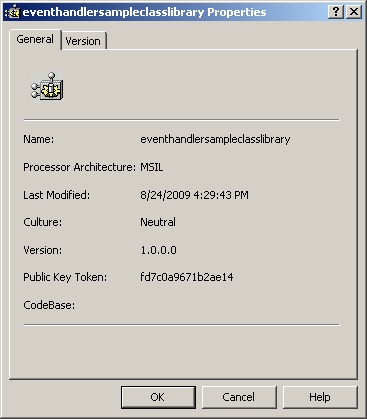
-
In Type select ItemDeleting.
-
Then call the update method.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.SharePoint;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
SPSite spsite = new SPSite("http://adfsaccount:2222/");
SPWeb mysite = spsite.OpenWeb();
SPList theList = mysite.Lists["Dhan"];
SPEventReceiverDefinition newReceiver = theList.EventReceivers.Add();
newReceiver.Class = "eventhandlersampleclasslibrary.Class1";
newReceiver.Assembly = "eventhandlersampleclasslibrary,Version=1.0.0.0,Culture=neutral,PublicKeyToken=fd7c0a9671b2ae14";
newReceiver.SequenceNumber = 5000;
newReceiver.Type = SPEventReceiverType.ItemDeleting;
newReceiver.Update();
Console.Read();
}
}
}
Now the event has been deployed.
Output
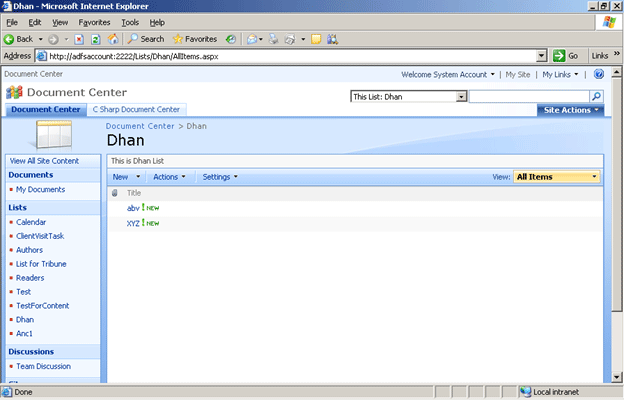
When I tried to delete the item
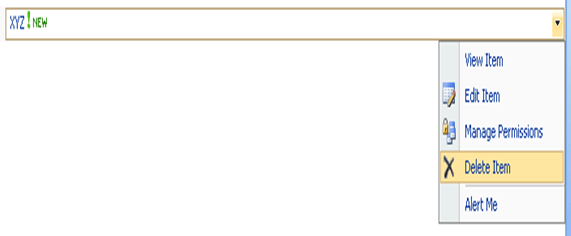
I got the below error message;
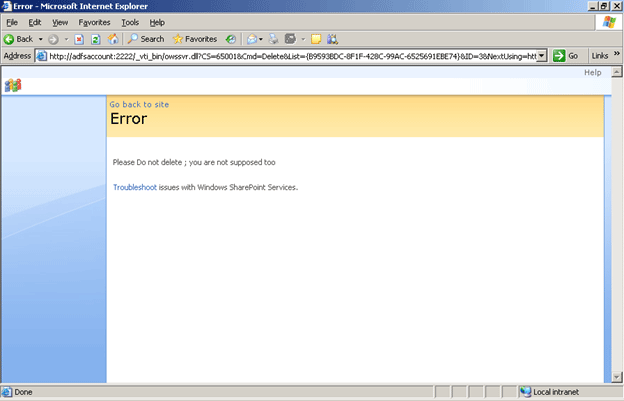
Conclusion
I have shown how to handle item deleting event. Whenever any item will get deleted user will get error message. Thanks for reading.
Happy Coding.