By default, a CRUD operation can be performed on the retrieved data from a LINQ query. We can modify the data fetched by a LINQ query.
IF we do not want to modify the data then we can increase the performance by making the data READ ONLY.

If we set ObjectTrackingEnabled false for DataContext then the framework will not track the changes done on the DataContext.
Program.cs
using System;
using System.Linq;
using System.Data.Linq;
namespace ConsoleApplication5
{
class Program
{
static void Main(string[] args)
{
DataClasses1DataContext context = new DataClasses1DataContext();
context.ObjectTrackingEnabled = false;
var result = from r in context.Persons orderby r.FirstName select r;
foreach (var r in result)
{
Console.WriteLine(r.FirstName + " " + r.LastName);
}
Console.ReadKey(true);
}
}
}
Output
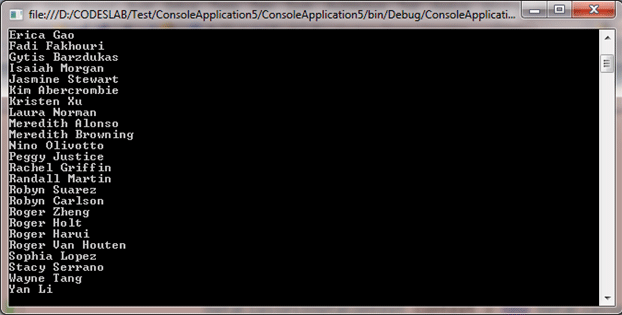
There are two scenarios while ObjectTrackingEnabled as false can throw an exception.
-
If we execute the query and after that make the ObjectTrackingEnabled false.
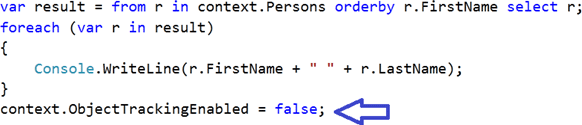
Then we will get the following exception:
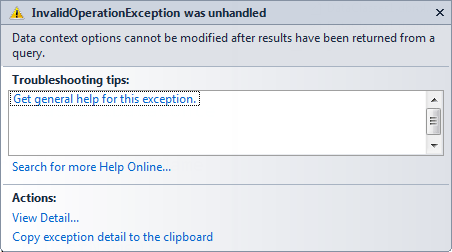
-
If we try to perform submitchanges() operation because DataContext is readonly.