Objective
This article is going to explain; how we can read RSS feeds in Silverlight 3.0.
Expected output
-
User will enter RSS URL in text box.
-
On click of Fetch Feed button ; RSS items will get populated.
-
On Clear Search button click text box and list box will be cleared.
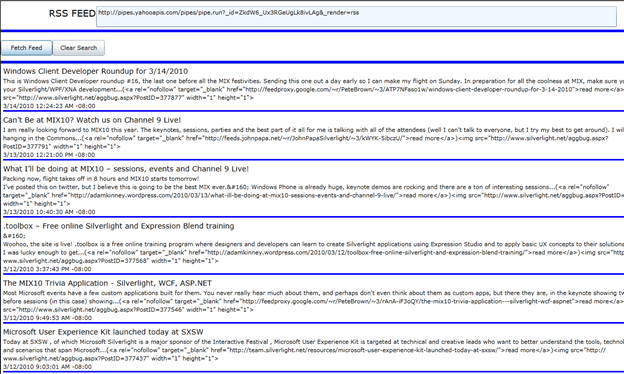
So, let us start follow the below steps
Step 1
Create a Silverlight application.
Step 2
Add an entity class. In this class RSS Feeds would be read. Right click Silverlight project and add a class. Give any name; I am giving name here MYFeed.
MyFeed.cs
namespace ReadingFeeds
{
public class MyFeed
{
public string Title { get; set; }
public string Summary { get ; set ;}
public string PublishedDate { get; set; }
public Uri Url { get; set; }
}
}
Step 3
Design the page
-
Divide grid in three rows.
-
In first and second row add stack panels.
-
Give orientation of stack panel as horizontal.
-
In first row; add one text block and one text box. User will enter RSS URL in text box provided here.
-
In second row; add two buttons. One button to fetch URL and other to clear the values.
-
In third row put a list box. Return items of RSS will be bind to this list box. List box is bind as follows.
<ListBox x:Name="myListBox" Grid.Row="2" ScrollViewer.HorizontalScrollBarVisibility="Disabled" >
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Vertical" >
<TextBlock x:Name="txtTitle" FontSize="14" Text="{Binding Title}"/>
<TextBlock x:Name="txtSummary" Text="{Binding Summary}" TextWrapping="Wrap"/>
<TextBlock x:Name="txtPublishedDate" Text="{Binding PublishedDate}" />
<TextBlock x:Name="txtUri" Text="{Binding Url}" />
<Rectangle Height="3" Fill="Blue" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
There is stack panel inside data template. There are different text blocks bind with different data.
MainPage.Xaml
<Grid x:Name="LayoutRoot">
<Grid.RowDefinitions>
<RowDefinition Height="1*" />
<RowDefinition Height="1*" />
<RowDefinition Height="12*" />
</Grid.RowDefinitions>
<StackPanel Grid.Row="0" Orientation="Horizontal" HorizontalAlignment="Center">
<TextBlock x:Name="lblFeed" Height="40" Text="RSS FEED" FontSize="18" />
<TextBox x:Name="txtRssFeed" Height="40" Width="1000" Background="AliceBlue" />
</StackPanel>
<StackPanel Grid.Row="1" Orientation="Horizontal">
<Button x:Name="btnFetchFeed" Height="30" Width="100" Content="Fetch Feed" VerticalAlignment="Center" />
<Button x:Name="btnClear" Height="30" Width="100" Content="Clear Search" VerticalAlignment="Center" />
</StackPanel>
<Rectangle Height="5" Fill="Blue" Grid.Row="0" VerticalAlignment="Bottom" />
<Rectangle Height="5" Fill="Blue" Grid.Row="1" VerticalAlignment="Bottom" />
<ListBox x:Name="myListBox" Grid.Row="2" ScrollViewer.HorizontalScrollBarVisibility="Disabled" >
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Vertical" >
<TextBlock x:Name="txtTitle" FontSize="14" Text="{Binding Title}"/>
<TextBlock x:Name="txtSummary" Text="{Binding Summary}" TextWrapping="Wrap"/>
<TextBlock x:Name="txtPublishedDate" Text="{Binding PublishedDate}" />
<TextBlock x:Name="txtUri" Text="{Binding Url}" />
<Rectangle Height="3" Fill="Blue" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
Step 4
-
To read RSS feed; first we need to create instance of WebClient class. This class helps us to make HTTP call from Silverlight.
WebClient proxy = new WebClient();
proxy.OpenReadCompleted += new OpenReadCompletedEventHandler(proxy_OpenReadCompleted);
proxy.OpenReadAsync(serviceURI);
Where serviceURI is RSS URL; we are going to fetch.
-
Create a list to read RSS feeds.
List<MyFeed> lstFeed = null;
-
Add reference of
using System.ServiceModel.Syndication;
-
Read result return from service in a Stream.
-
Create XMLReader from the stream.
-
Load SyndicationFeed from the XMLReader.
Stream stream = e.Result;
XmlReader response = XmlReader.Create(stream);
SyndicationFeed feeds = SyndicationFeed.Load(response);
-
Fetch all the SyndicationItem ina foreach loop and create MyFeed instance and add to list.
-
Bind list as datasourec of List box.
MainPage.Xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.IO;
using System.Xml;
using System.ServiceModel.Syndication;
using System.Collections;
namespace ReadingFeeds
{
public partial class MainPage : UserControl
{
List<MyFeed> lstFeed = null;
MyFeed feed = null;
public MainPage()
{
InitializeComponent();
btnFetchFeed.Click += new RoutedEventHandler(btnFetchFeed_Click);
btnClear.Click += new RoutedEventHandler(btnClear_Click);
}
void btnClear_Click(object sender, RoutedEventArgs e)
{
myListBox.SelectedIndex = -1;
myListBox.ItemsSource = null;
txtRssFeed.Text = "";
}
void btnFetchFeed_Click(object sender, RoutedEventArgs e)
{
Uri serviceURI = null;
if (string.IsNullOrEmpty(txtRssFeed.Text))
{
MessageBox.Show("RSS Field is empty ");
}
else
{
serviceURI = new Uri(txtRssFeed.Text.Trim());
WebClient proxy = new WebClient();
proxy.OpenReadCompleted += new OpenReadCompletedEventHandler(proxy_OpenReadCompleted);
proxy.OpenReadAsync(serviceURI);
}
}
void proxy_OpenReadCompleted(object sender, OpenReadCompletedEventArgs e)
{
lstFeed = new List<MyFeed>();
Stream stream = e.Result;
XmlReader response = XmlReader.Create(stream);
SyndicationFeed feeds = SyndicationFeed.Load(response);
foreach (SyndicationItem f in feeds.Items)
{
Uri imgUri = f.BaseUri;
feed = new MyFeed() { Title = f.Title.Text, Summary = f.Summary.Text, PublishedDate = f.PublishDate.ToString(), Url = imgUri };
lstFeed.Add(feed);
}
myListBox.ItemsSource = lstFeed;
}
}
}
Press F5 and run the application.
Conclusion
In this article I discussed how to read RSS in Silverlight 3.0. Thanks for reading.