In this article we will retrieve data from SharePoint list and display it Windows 7 phone application.
The expected output is as below,
Flow Diagram
Now we will follow the following steps to perform all above operations.
Step 1: Setting up the SharePoint list
We have a custom list
- Name of the list is Test_Product.
- Columns of the list is as below
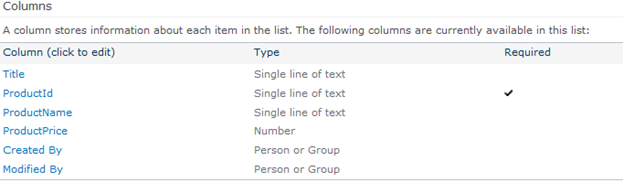
- There are two items in the list

- URL of the SharePoint site is
http://dhananjay-pc/my/personal/Test1
Now we will access the SharePoint site on above URL.
Step 2: Creating WCF Service
Now we will create a WCF Service. WCF Service will have four operation contracts for CRUD operation on list.
To create WCF service, open visual studio and from WCF tab select WCF Service application.
Now to make sure that we are able to use SPLinq or LINQ to SharePoint we need to change the target framework and Platform target
So to do those follow as below,
- Right click and click on Properties of WCF Service
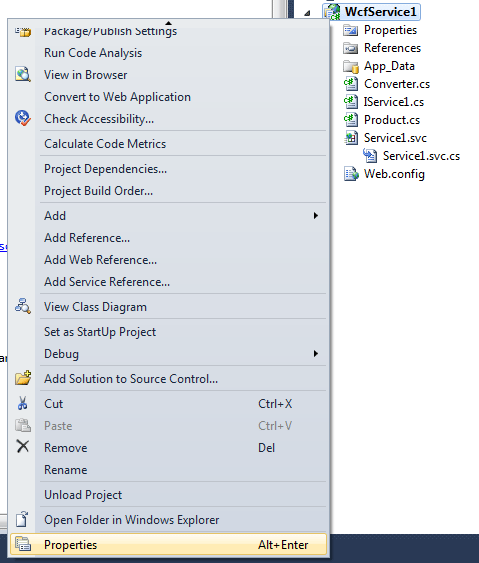
- Click on the Build tab and change Platform Target to Any CPU.
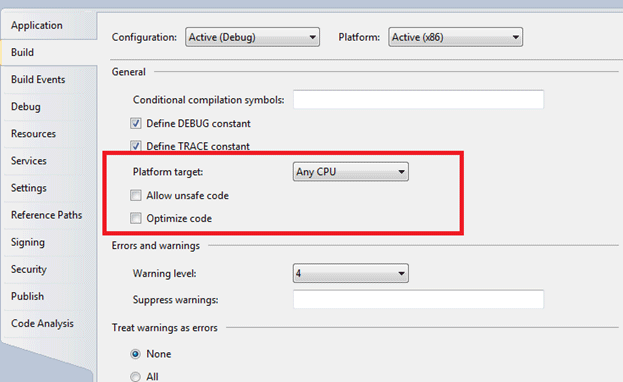
- Click on the Application tab and change the Target framework type to .Net Framework 3.5
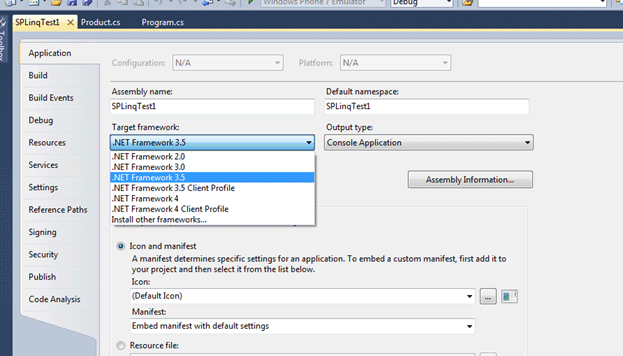
Now we need to create DataContext class of SharePoint list such that we can perform LINQ against that class to perform CRUD operation. To create context class we need to perform the following steps.
- Open the command prompt and change directory to
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\BIN
Type command CD C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\BIN
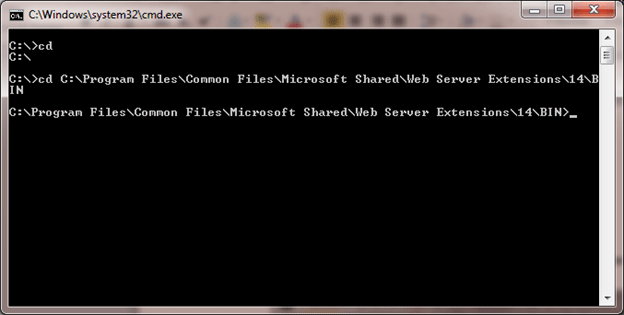
- Now we need to create the class for corresponding list definitions.
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\BIN> spme
tal.exe /web:http://dhananjay-pc/my/personal/Test1 /namespace:nwind /code:Product.cs
In the preceding command we are passing a few parameters to spmetal.exe, they are as below
a. /web:Url
Here we need to provide URL of SharePoint site
/web:http://dhananjay-pc/my/personal/Test1 /://dhananjay-pc/my/personal/Test1 / is URL of SharePoint site, I created for myself. You need to provide your SharePoint site URL here.
b. /namespace:nwind
This would be the namespace under which class of the list will get created. In my case name of the namespace would be nwind.
c. /code:Product.cs
This is the file name of the generated class. Since we are giving name Product for the file then class generated will be ProductDataContext
The classes default gets saved in the same folder with SPMetal.exe. So to see where the class got created we need to navigate to folder
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\BIN
Add created class to the WCF Service project
Now add this class to the project. To do this, right click on the WCF Service project and select Add existing item. Then browse to above path and select Product.cs
Host the WCF Service in IIS
To work with SPLInq in WCF service, we need to host the service in IIS with the same application pool SharePoint is running.
See the below link for step by step explanation on how to host WCF 4.0 Service in IIS 7.5
Only we need to make sure that,
Hosted WCF service is sharing the same application pool with SharePoint.
Select the Application pool to SharePoint-80
Create Contracts
We need to create DataContract or Data Transfer object. This class will represent the Product Data context class (We generated this class in previous step) 0
Create Data Contract,
[DataContract]
public class ProductDTO
{
[DataMember]
public string ProductName;
[DataMember]
public string ProductId;
[DataMember]
public string ProductPrice;
}
Add References to work with LINQ to SharePoint
Microsoft.SharePoint
Microsoft.SharePoint.Linq
Right click on Reference and select Add Reference. To locate Microsoft.SharePoint and Microsoft.SharePoint.Linq dll browse to C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\ISAPI. All the SharePoint dll are here in this location.
Add the namespace
Nwind is the name of the namespace of the data context class we created in previous steps. We need to make sure that we have added Product class to the WCF Service application project as Add an Existing item.
Create Service Contract
We need to create Service contract. There would be four operation contracts each for one operation on Sharepoint list.
IService1.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.ServiceModel;
using System.Runtime.Serialization;
using System.ServiceModel.Description;
namespace WcfService1
{
[ServiceContract ]
public interface IService1
{
[OperationContract]
List<ProductDTO> GetProduct();
}
Implement the Service
Now we need to implement the service to perform operations
Retrieving all the list items
public List<ProductDTO> GetProduct()
{
using (ProductDataContext context = new ProductDataContext("http://dhananjay-pc/my/personal/Test1"))
{
ProductDTO product;
List<ProductDTO> lstProducts = new List<ProductDTO>();
var result = from r in context.Test1_Product select r;
foreach (var r in result)
{
product = new ProductDTO { ProductId = r.ProductId, ProductName = r.ProductName, ProductPrice = r.ProductPrice.ToString ()
};
lstProducts.Add(product);
}
return lstProducts;
}
}
In the preceding code
- Creating instance of ProductDataContext.
- Using LINQ to SharePoint retrieving all the list items.
- Creating instance of ProductDTO class with values fetched from SharePoint list
- Adding the instance of ProductDTO class in List of ProductDTO.
- Returning list of ProductDTO
Modify the Web.Config
- We will put Binding as basicHttpBinding.
- In ServiceBehavior attribute , we will enable the servicemetadata
- In ServiceBehavior , we will make IncludeserviceException as false.
- We will configure MetadataExchnagePoint in the service
So, Now in Web.Config Service configuration will look like
<system.serviceModel>
<behaviors>
<serviceBehaviors>
<behavior name="MyBeh">
<serviceMetadata httpGetEnabled="true"/>
<serviceDebug includeExceptionDetailInFaults="false"/>
</behavior>
</serviceBehaviors>
</behaviors>
<services>
<service name="WcfService1.Service1" behaviorConfiguration="MyBeh">
<endpoint address="" binding="basicHttpBinding" contract="WcfService1.IService1"/>
<endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange"/>
<host>
<baseAddresses>
<add baseAddress="http://localhost:8181/Service1.svc/"/>
</baseAddresses>
</host>
</service>
</services>
<!--<serviceHostingEnvironment multipleSiteBindingsEnabled="true"/>-->
</system.serviceModel>
Now our service is created. We will be hosting this service in IIS as we discussed in previous step. From IIS we will browse to test whether service is up and running or not.
For reference, full source code for service implementation is as below ,
Service1.svc.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Runtime.Serialization;
using System.ServiceModel;
using System.ServiceModel.Web;
using System.Text;
using Microsoft.SharePoint.Linq;
using Microsoft.SharePoint;
using nwind;
namespace WcfService1
{
public class Service1 : IService1
{
public List<ProductDTO> GetProduct()
{
using (ProductDataContext context = new ProductDataContext("http://dhananjay-pc/my/personal/Test1"))
{
ProductDTO product;
List<ProductDTO> lstProducts = new List<ProductDTO>();
var result = from r in context.Test1_Product select r;
foreach (var r in result)
{
product = new ProductDTO { ProductId = r.ProductId, ProductName = r.ProductName, ProductPrice = r.ProductPrice.ToString () };
lstProducts.Add(product);
}
return lstProducts;
}
}
}
Creating a Windows 7 Phone application
Open visual studio and create a new Windows Phone application from Silverlight from Windows phone project tab.
Add the service reference. Right click and add the Service reference of the WCF service created in previous step to the phone application.
Create the Product Client class
ProductClient.cs
public class ProductatClient
{
public string ProductId { get; set; }
public string ProductName { get; set; }
public string ProductPrice { get; set; }
}
Divide the content grid in two rows and put button in first row and list box in second row. We will create button and on click event of this button we will fetch data from the WCF service.
Now we will create a List box to bind the SharePoint list data
So the full XAML is as below,
MainPage.xaml
<phone:PhoneApplicationPage
x:Class="WindowsPhoneApplication1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="480" d:DesignHeight="768"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
SupportedOrientations="Portrait" Orientation="Portrait"
shell:SystemTray.IsVisible="True">
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="Windows 7" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="SharePoint List" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<Grid.RowDefinitions>
<RowDefinition Height="1*"/>
<RowDefinition Height="4*"/>
</Grid.RowDefinitions>
<Button x:Name="btnGetData"
Content="Get Data from SharePoint list"
Margin="0,0,19,43" FontWeight="ExtraBold"
Foreground="#FFFCAEAE" FontStyle="Italic"
FontStretch="UltraCondensed"
Cursor="Hand"
BorderBrush="#FFB76C6C"
FontSize="18" FontFamily="Arial">
<Button.Background>
<LinearGradientBrush EndPoint="1,0.5" StartPoint="0,0.5">
<GradientStop Color="Black" Offset="0" />
<GradientStop Color="#FF902727" Offset="1" />
</LinearGradientBrush>
</Button.Background>
</Button>
<ListBox x:Name="lstBox"
Grid.Row="1"
FontSize="18"
BorderThickness="2,3,2,0"
DataContext="{Binding ElementName=lstBox}"
FontStretch="ExtraExpanded" Background="#FF8996DE">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal" >
<TextBlock x:Name="txtProductId"
Margin="15"
Text="{Binding Path=ProductId, Mode=OneWay}"
Foreground="White"
FontWeight="ExtraBold"
FontSize="24"
FontFamily="Arial" />
<TextBlock x:Name="txtProductName"
Margin=" 15"
Text="{Binding ProductName, Mode=OneWay}"
Foreground="White" FontWeight="ExtraBold"
FontSize="24"
FontFamily="Arial" />
<TextBlock x:Name="txtProductPrice"
Margin=" 15"
Text="{Binding ProductPrice, Mode=OneWay}"
Foreground="White"
FontWeight="ExtraBold"
FontSize="24" FontFamily="Arial" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Grid>
</phone:PhoneApplicationPage>
Now at the code behind, we need to fetch the data from WCF service
So the full source code is as below ,
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using Microsoft.Phone.Controls;
using WindowsPhoneApplication1.ServiceReference1;
namespace WindowsPhoneApplication1
{
public partial class MainPage : PhoneApplicationPage
{
// Constructor
public MainPage()
{
InitializeComponent();
btnGetData.Click += new RoutedEventHandler(btnGetData_Click);
}
void btnGetData_Click(object sender, RoutedEventArgs e)
{
// MessageBox.Show("Dhananjay");
Service1Client proxy = new Service1Client();
proxy.GetProductCompleted += new EventHandler<GetProductCompletedEventArgs>(proxy_GetProductCompleted);
proxy.GetProductAsync();
}
void proxy_GetProductCompleted(object sender, GetProductCompletedEventArgs e)
{
List<ProductatClient> lstResults = new List<ProductatClient>();
ProductatClient p;
List<ProductDTO> results = e.Result.ToList();
foreach (var r in results)
{
p = new ProductatClient { ProductName = r.ProductName,
ProductId = r.ProductId,
ProductPrice = r.ProductPrice };
lstResults.Add(p);
}
lstBox.ItemsSource = lstResults;
}
}
}
Now when we run the application, we will get the output as below,