Objective:
This article will discuss about, different way of consuming HTTP enabled service (like REST).This article, will explain about WebGet and HttpWebRequest. Before reading this article, I will suggest readers to read my previous articles on REST, ADO.NET Data Service and Cross Domain issue on our site.
Assumption:
- Already REST service has been created and hosted within placed client access policy file.
- There is a Silverlight Client. Which is going to consume WebGet service of REST
How to access HTTP based service in Silverlight?
- HTTP based service returned data on a HTTP request in a particular URI
- HTTP request can be send any type of URI like,
http://trvlsdw002/synergyservices/appointmentservice.svc/Appointments/
http://b068ltrv/SynergyService/AppointmentService.svc/Appointments/Mahesh
http://localhost:22947/AppointmentService.svc"/Appointments/Praveen
- HTTP request is cofigured to work with , verb GET
- Required parameter to invoke service (WebGet sercvice) should be appended with URI
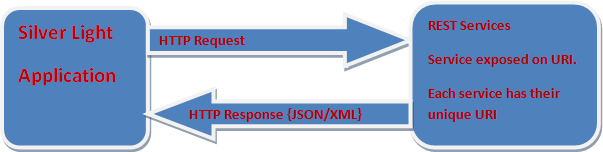
- The output service data is contained in HTTP Response.
- Format of data depends on service implementation, but it mainly in format of JOSN, XML or RSS.
Silverlight provides two methods for sending HTTP request
- WebClient
- HttpWebRequest
Difference between WebClient and HttpWebRequest
- HttpWebRequest supports large subset of HTTP Protocol , which makes it better option for advanced scenarios
- HttpWebRequest uses asynchronous programming model which uses delegates but on the other hand WebClient uses event based asynchronous programming model. So it is easier to use and it needs lessee line of code.
- Callback for WebClient is raised for HTTP Response and it is invoked on the same user interface thread. So it is easier to update property of UI element. That is why it is easy with WebClient to bind UI properties with data of Http response.
Whereas, HttpWebRequest callback run on different thread. So there is need of write extra code for UI binding.
WebClient
Namespace: System.Net
WebClient class is being used in Silverlight control which is hosted in a webpage.
The following table describes WebClient methods for uploading data to a resource.
Method |
Description |
OpenWriteAsync |
Retrieves a Stream used to send data to the resource asynchronously, without blocking the calling thread. |
UploadStringAsync |
Sends a String to the resource, without blocking the calling thread. |
The following table describes WebClient methods for downloading data from a resource.
Method |
Description |
DownloadStringAsync |
Downloads a String from a resource, without blocking the calling thread. |
OpenReadAsync |
Returns the data from a resource asynchronously, without blocking the calling thread. |
Steps to call Http based service
- Create URI , where request is to be sent
string baseuri = "http://trvlsdw002/synergyservices/appointmentservice.svc/Appointments";
- Take care of cross domain issue here.
- Create instance of WebClient. don't forget to ass namespace System.Net
WebClient wc = new WebClient();
- Now ,
wc.DownloadStringCompleted += ParseProducts_AsXml;
Here ParseProducts_AsXml is event , which will parse HTTP Response in XML format.
If response format is JSON , then
wc.DownloadStringCompleted += ParseProducts_AsJson
Note : Here , ParseProducts_AsXml and ParseProducts_AsJson are user defined events. We need to define these events later.
- Now need to call ,
Uri uri = new Uri(baseuri);
wc.DownloadStringAsync(uri);
- Parse the response either as XML or as JOSN depending on response type of Http Response.
For parsing as XML, given code might help. Here response is being passed in Product business object. In e.Result, result stream is coming to Silverlight application. Just need to desterilize that.
private void ParseProducts_AsXml(object sender, DownloadStringCompletedEventArgs e)
{
string abc = "";
string rawXmlresponse = e.Result;
XDocument xdoc = XDocument.Parse(rawXmlresponse);
var query = from product in xdoc.Descendants(abc + "Product")
select new Product
{
ProductId = product.Element("ProductId").Value.ToInt(),
ProductName = product.Element("ProductName").Value,
UnitPrice = product.Element("UnitPrice").Value.ToDecimal()
};
List<Product> products = query.ToList() as List<Product>;
lstProducts.DataContext = products;
}
For parsing as JOSN below code might help.
private void ParseProducts_AsJson(object sender, DownloadStringCompletedEventArgs e)
{
string raw = e.Result;
JsonArray json;
if (JsonArray.Parse(raw) as JsonArray == null)
json = new JsonArray { JsonObject.Parse(raw) as JsonObject };
else
json = JsonArray.Parse(raw) as JsonArray;
var query = from product in json
select new Product
{
ProductId = (int)product["ProductId"],
ProductName = (string)product["ProductName"],
UnitPrice = (decimal)product["UnitPrice"]
};
List<Product> products = query.ToList() as List<Product>;
lstProducts.DataContext = products;
}
For Desterilizing as JOSN using DataContractJsonSerliazer
private void ParseProducts_AsJson_UsingDataContractJsonSerializer(object sender, DownloadStringCompletedEventArgs e)
{
string json = e.Result;
DataContractJsonSerializer serializer =
new DataContractJsonSerializer(typeof(List<Product>));
//DataContractJsonSerializer serializer = new DataContractJsonSerializer(products.GetType());
MemoryStream stream = new MemoryStream(Encoding.UTF8.GetBytes(json));
List<Product> products = serializer.ReadObject(stream) as List<Product>;
stream.Close();
lstProducts.DataContext = products;
}
Note:
-
There is no direct way, to expose DataContract to client in RESTful service. So workaround need to be done. There is need to create Business class at Silverlight client side. In above examples business class is Product.
-
All the code written above is consuming REST service, which is returning List of appointments.
[OperationContract(Name="GetAllAppointments")]
[WebGet(UriTemplate = "/Appointments/")]
List<AppointmentDTO> GetAppointments();
Here, AppointmentDTO is business object. Which has to be created at client side too?
Happy Coding!