Objective
Objective of this aricle is to show, how to work with Images in ASP.Net MVC application. This article will explain, how to display images from Xml file and how to add images in Xml resourece.
The Final Output would be something like below.
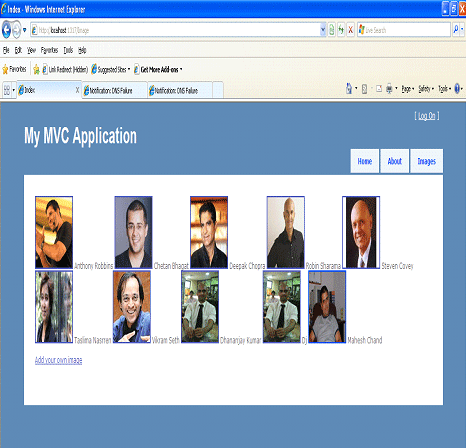
Creating the Resource folder for Image
-
Create a ASP.Net MVC application, by New->Project->Web->ASP.Net MVC Application.
-
Now add a folder in application and give it name as
Images.
-
Add few images in Images folder. For that right click on Image folder and
add existing items.
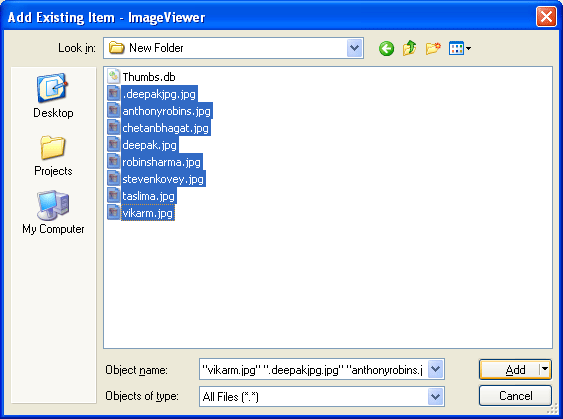
-
After adding Images in Images folder. Folder will look like below.
-
Add an
XML file in Image directory as source of the images. Give any name, here name is
ImageMetaData.xml. To add XML file in Images directory, right click on directory and add XML File as shown below.
-
Create XML elements for images. Here filename and description are xml elements. Give value according to name of your added images. For my Images the xml file is as below.
<?xml version="1.0" encoding="utf-8" ?>
<images>
<image>
<filename>anthonyrobins.jpg</filename>
<description>Anthony Robbins</description>
</image>
<image>
<filename>chetanbhagat.jpg</filename>
<description>Chetan Bhagat</description>
</image>
<image>
<filename>deepak.jpg</filename>
<description>Deepak Chopra</description>
</image>
<image>
<filename>robinsharma.jpg</filename>
<description>Robin Sharama</description>
</image>
<image>
<filename>stevenkovey.jpg</filename>
<description>Steven Covey</description>
</image>
<image>
<filename>taslima.jpg</filename>
<description>Taslima Nasrren</description>
</image>
<image>
<filename>vikarm.jpg</filename>
<description>Vikram Seth</description>
</image>
</images>
Displaying Images
Creating the Model
Right click on Model folder and add new class. Give name of the class as Image.
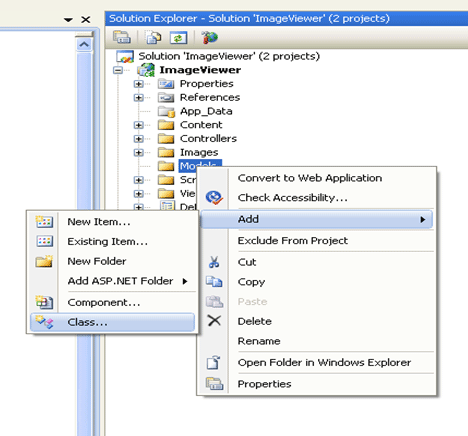
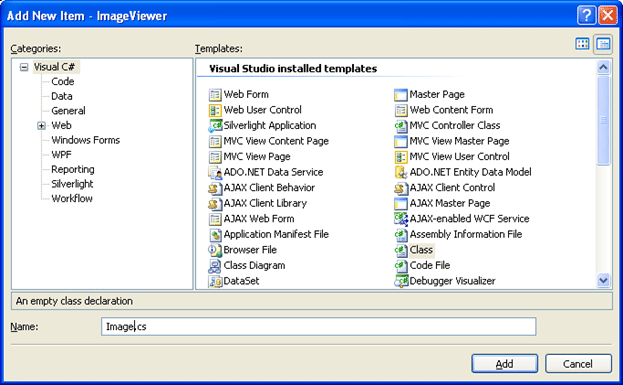
Type the below code inside the Image class. This class is acting as a business class. This class got two properties . One for Path of the image and other for Description of the image.
Image.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace ImageViewer.Models
{
public class Image
{
public Image(string path, string desc)
{
Path = path;
Description = desc;
}
public string Path { get; set; }
public string Description { get; set;}
}
}
We need to fetch data from the disk. For this, we will add one more model class and in constructor of that class, we will fetch all the images from xml file. So add one class in Model folder as shown in step above. Give name ImageModel.
ImageModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Xml.Linq;
namespace ImageViewer.Models
{
public class ImageModel:List<Image>
{
public ImageModel()
{
string directoryOfImage = HttpContext.Current.Server.MapPath("~/Images/");
XDocument imageData = XDocument.Load(directoryOfImage + @"/ImageMetaData.xml");
var images = from image in imageData.Descendants("image") select new Image(image.Element("filename").Value, image.Element("description").Value);
this.AddRange(images.ToList<Image>());
}
}
}
Creating the Controller
Right Click on Controller and add a controller called ImageController.
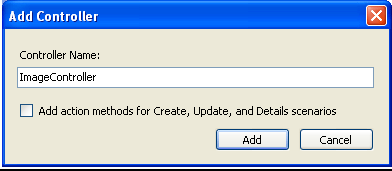
Type or paste below code inside ImageController class. This class got an action, which is returning View with instance of ImageModel in View dictionary.
Controller\ImageController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Xml.Linq;
namespace ImageViewer.Models
{
public class ImageModel:List<Image>
{
public ImageModel()
{
string directoryOfImage = HttpContext.Current.Server.MapPath("~/Images/");
XDocument imageData = XDocument.Load(directoryOfImage + @"/ImageMetaData.xml");
var images = from image in imageData.Descendants("image") select new Image(image.Element("filename").Value, image.Element("description").Value);
this.AddRange(images.ToList<Image>());
}
}
}
Creating the View
Right click on View folder and add new View. Name of the View must be Index because controller name is here Index.
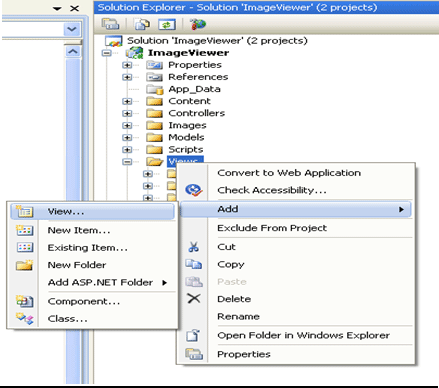
-
Again right click on Image Folder in View and add a class. Give name of the class as Index.cs.
-
Make this class as a partial class.
-
Add reference
using ImageViewer.Models;
-
Inherit ViewPage<> in this partial class.
-
Pass model class as parameter of ViewPage<ImageModel>
View/Image/Index.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ImageViewer.Models;
namespace ImageViewer.Views.Image|
{
public partial class Index : ViewPage<ImageModel>
{
}
}
-
Open index.aspx
-
Set code behind file as
CodeBehind="~/Views/Image/Index.cs"
-
Here we will fetch all the images from ViewData dictionary.
View/Image/Index.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="ImageViewer.Views.Image.Index" CodeBehind="~/Views/Image/Index.cs" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Index
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<% foreach (var image in ViewData.Model) { %>
<span class="image">
<a href="images/<%= image.Path %>">
<img src="images/<%= image.Path %>" height="100" width="100" /></a>
<span class="description"><%= image.Description %></span>
</span>
<% }%>
</asp:Content>
Adding Link to navigate Image from Home page
-
Click on View/Shared/site.Master
-
Add below Action Link
<li><%=Html.ActionLink("Images","Index","Image") %></li>
So, now three action links would be there in master page
<ul id="menu">
<li><%= Html.ActionLink("Home", "Index", "Home")%></li>
<li><%= Html.ActionLink("About", "About", "Home")%></li>
<li><%=Html.ActionLink("Images","Index","Image") %></li>
</ul>
Press F5 to run with debugging
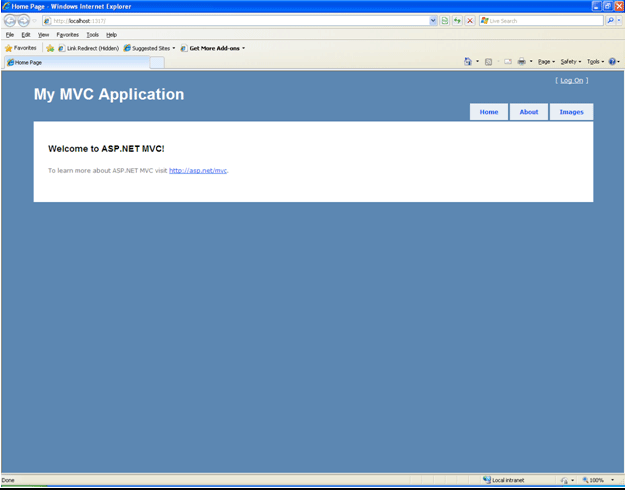
Click on Image tab to get Images.
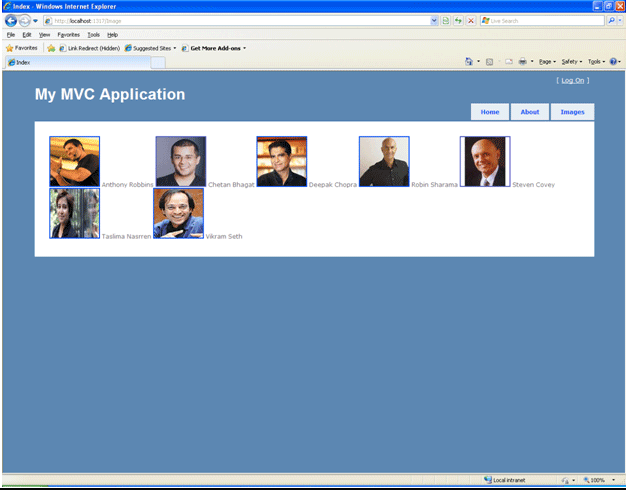
Adding Image
Amending the Model
Go to ImageModel class and add below method, which will add image in Images folder and its entry in XML file.
public void Add(Image image, HttpPostedFileBase filebase)
{
string imagerep = HttpContext.Current.Server.MapPath("~/Images/");
filebase.SaveAs(imagerep + image.Path);
this.Add(image);
XElement xml = new XElement("images", from i in this
orderby image.Path
select new XElement("image",
new XElement("filename", i.Path),
new XElement("description", i.Description))
);
XDocument doc = new XDocument(xml);
doc.Save(imagerep + "/ImageMetaData.xml");
}
So, now the ImageModel class is been modified to
Models\ImageModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Xml.Linq;
namespace ImageViewer.Models
{
public class ImageModel:List<Image>
{
public ImageModel()
{
string directoryOfImage = HttpContext.Current.Server.MapPath("~/Images/");
XDocument imageData = XDocument.Load(directoryOfImage + @"/ImageMetaData.xml");
var images = from image in imageData.Descendants("image") select new Image(image.Element("filename").Value, image.Element("description").Value);
this.AddRange(images.ToList<Image>());
}
public void Add(Image image, HttpPostedFileBase filebase)
{
string imagerep = HttpContext.Current.Server.MapPath("~/Images/");
filebase.SaveAs(imagerep + image.Path);
this.Add(image);
XElement xml = new XElement("images", from i in this
orderby image.Path
select new XElement("image",
new XElement("filename", i.Path),
new XElement("description", i.Description))
);
XDocument doc = new XDocument(xml);
doc.Save(imagerep + "/ImageMetaData.xml");
}
}
}
Amending the Controller
In ImageController add actions which will perform task of adding the image into the resource. For that add below actions called Add and Save in Image Controller.
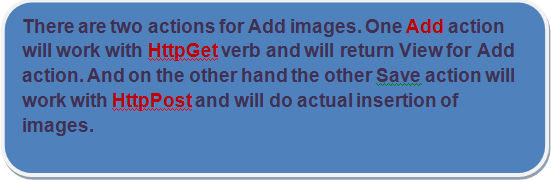
So, now Image Controller would look like below. Both Add and Save actions are highlighted in different colors.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using System.Web.Mvc.Ajax;
using ImageViewer.Models;
namespace ImageViewer.Controllers
{
public class ImageController : Controller
{
//
// GET: /Image/
public ActionResult Index()
{
return View(new ImageModel());
}
public ActionResult Add()
{
return View();
}
[AcceptVerbs(HttpVerbs.Post)]
public ActionResult Save()
{
foreach (string name in Request.Files)
{
var file = Request.Files[name];
string fileName = System.IO.Path.GetFileName(file.FileName);
Image image = new Image(fileName, Request["description"]);
ImageModel model = new ImageModel();
model.Add(image, file);
}
return RedirectToAction("index");
}
}
}
Creating the View
Right click on View folder and add new View. Name of the View must be Index because controller name is here Add.
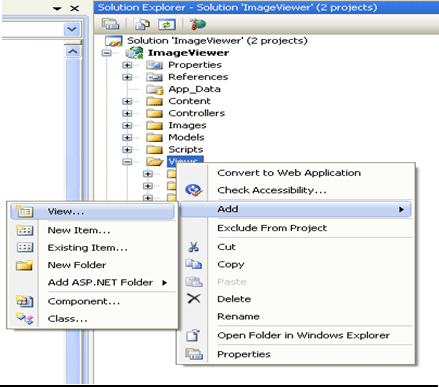
-
Again right click on Image Folder in View and add a class. Give name of the class as Add.cs.
-
Make this class as a partial class.
-
Add reference
using ImageViewer.Models;
-
Inherit ViewPage<> in this partial class.
-
Pass model class as parameter of ViewPage<ImageModel>
View/Image/Add.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ImageViewer.Models;
namespace ImageViewer.Views.Image
{
public partial class Add: ViewPage<ImageModel>
{
}
}
-
Open Add.aspx
-
Set code behind file as
CodeBehind="~/Views/Image/Add.cs"
-
Type the below code in Add.aspx
View/Image/Add.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" CodeBehind="~/Views/Image/Add.cs" %>
<asp:Content ID="Content1" ContentPlaceHolderID="MainContent" runat="server">
Add
<form method="post" action="<%=Url.Action("Save") %>" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="submit" value="submit" /> <br />
<input type="text" name="description" />
</form>
</asp:Content>
Amending Views\Index.aspx
Add below ActionLink in index.aspx.
<p><%= Html.ActionLink("Add your own image", "Add", "Image")%></p>
So, Now modified Index.aspx will look like below,
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="ImageViewer.Views.Image.Index"
CodeBehind="~/Views/Image/Index.cs" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Index
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<% foreach (var image in ViewData.Model) { %>
<span class="image">
<a href="images/<%= image.Path %>">
<img src="images/<%= image.Path %>" height="100" width="100" /></a>
<span class="description"><%= image.Description %></span>
</span>
<% }%>
<p><%= Html.ActionLink("Add your own image", "Add", "Image")%></p>
</asp:Content>
Press F5 to run the application
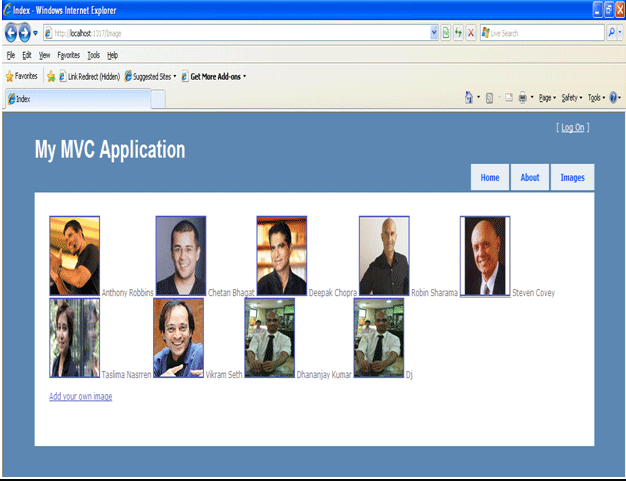
Click on Add your own image to add new Image. Browse the Image, give a description and click on submit button.
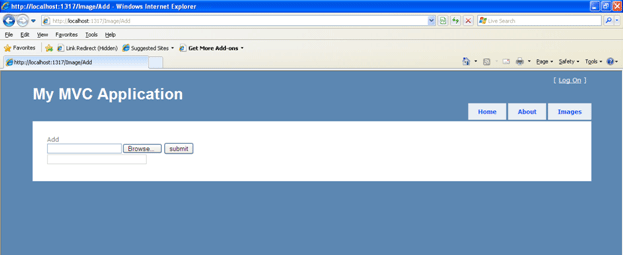
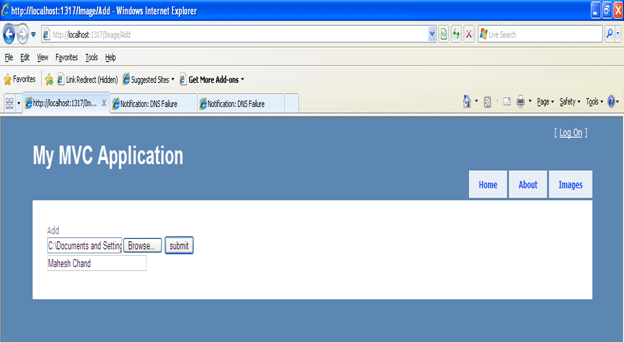
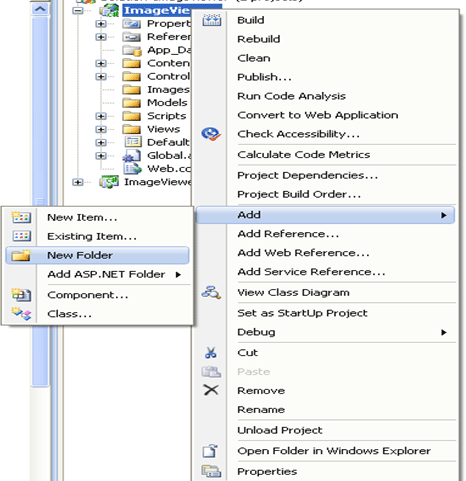
Future Scope:
-
To add functionality for Delete and update Description for a particular image.
-
Modify the data source from an xml file to database.
How to use Zip file:
Just download Zip file and run it.
Happy Coding.