Introduction
There are several articles on the web on how to export a GridView or DataGrid into excel. The object of this article is to extend that functionality into a loosely implemented exporting factory. Because we don't want to repeat other material we will add exporting support for Excel, HTML, and PDF. The PDF exporter will take advantage of the iTextSharp library (Version 5.0.4.0).
Interface
Interfaces create a contract with your class, stating that they will implement a particular method or function. For our simple library the Export method will take in two parameters; HttpResponse and GridView. The interface will be important because the exporting classes will be internal and all the implementation will be hidden.
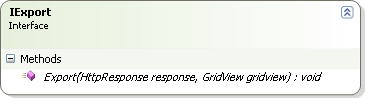
PdfExporter
The PdfExporter will have a reference to the iTextShap library and two fields to hold our Document and PdfWriter. The classes were sealed because we don't want any other class to inherit functionality and reduce the compiler overhead of traversing the class hiarchy looking for sub classes. There are two more code segments that may stand out from the class. The first is that a destructor was implemented.
~PdfExporter()
{
if (m_writer != null)
m_writer.Close();
…
}
The other option was to use the IDispose interface, but I am going to trust the GC in this case and way for my class to go out of scope to make sure all the memory was released. The second code change is the use of the Response.OutputStream for our PdfWriter.GetInstance function.
m_writer = PdfWriter.GetInstance(m_doc, response.OutputStream);
This will take care of having to actually writer a byte array or keep track of a MemoryStream.
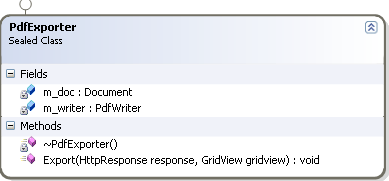
Excel/HtmlExporters
Some of the issues I had using the HtmlTextWriter for exporting were with the GridView.RenderControls expecting a form tag as the container. To resolve the container issue a wrapper was created to convert the row cells into HtmlTableCells. The entire code to process the GridView is below.
HtmlTableHelper htmlHelper = new HtmlTableHelper();
htmlHelper.AddCells(htmlHelper.GetRow(), HtmlTableHelper.GetList(gridview.HeaderRow.Cells));
htmlHelper.Table.Border = 1;
foreach (GridViewRow row in gridview.Rows)
{
htmlHelper.AddCells(htmlHelper.GetRow(), HtmlTableHelper.GetList(row.Cells));
}
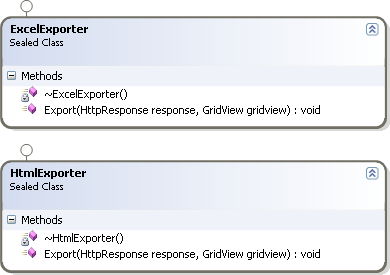
ExportFactory
The ExportFactory only contains one function called GetExporter with a return type IExport.
public static IExport GetExporter(ExportFormat Format)
{
switch (Format)
{
case ExportFormat.PDF:
return new PdfExporter();
case ExportFormat.Html:
return new HtmlExporter();
case ExportFormat.Excel:
return new ExcelExporter();
default:
throw new Exception(string.Format("Export Forma: {0} not supported.", Format.ToString()));
}
}
We are returning the interface because we don't want to expose our implementation classes so the implementing code will look like:
ExportFactory.GetExporter(ExportFormat.PDF).Export(Response, gvProviderSearchResults);
Note
I hope that the different exporters and concepts covered assist in the reader's next project. Please leave comments and suggestions as there are better or more efficient ways to perform a task in programming.