Introduction
Language Integrated Query (LINQ) is considered one of the most important features to all developers nowadays; the powerfull feature of LINQ to execute complex queries from any datasourse with simple, clean and concise code.
Linq has different styles; LINQ to SQL, LINQ to Dataset, LINQ to XML and LINQ to object. LINQ to object gives us the capability to write declarative code to retrieve data from collections which implements IEnumerable or IEnumerable<T> interfaces.
The following project prepares us for use of LINQ to object to extract and show data in DataGridView. First we create the CandidatesForJob class containing several properties for later retrieval. Secondly, we decalre BindingList<> object of type CandidatesForJob class, hence, when the user fills every textbox with its apropriate property, all of CandidatesForJob properties will be added to every object in BindingList<> collection. At last, executing simple LINQ query will deliver data to dataGridView1 to show the result.
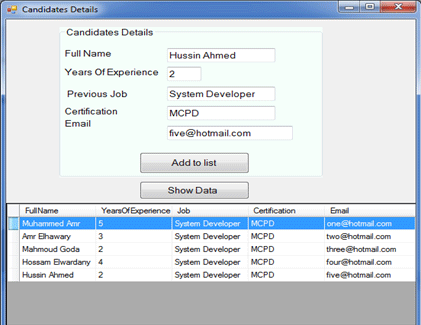
using System;
using System.Linq;
using System.Windows.Forms;
using System.ComponentModel;
namespace LinqToObjectWithDataGridView
{
public partial class Form1 : Form
{
// decalre BindingList<> object of type CandidatesDetails class
BindingList<CandidatesForJob> CandidatesDetails = new BindingList<CandidatesForJob>();
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button2_Click(object sender, EventArgs e)
{
// Add CandidatesForJob object to CandidatesDetails list
CandidatesDetails.Add(new CandidatesForJob
{
FullName=textBox1.Text,
YearsOfExperience = int.Parse(textBox2.Text),
Job =textBox3.Text,
Certification = textBox4.Text,
Email = textBox5.Text
});
}
private void button1_Click(object sender, EventArgs e)
{
// Execute Linq query over collection of CandidatesDetails
var q = from w in CandidatesDetails select w;
dataGridView1.DataSource = q.ToList();
}
}
public class CandidatesForJob
{
public CandidatesForJob()
{
}
// Automatic properties of CandidatesForJob
public string FullName { get; set; }
public int YearsOfExperience { get; set; }
public string Job { get; set; }
public string Certification { get; set; }
public string Email { get; set; }
}
}