Introduction
This article is intended to illustrate how to implement the master-detail presentation pattern using the ObjectDataSource components and DropDownList Web control. Master-detail presentation pattern is one of the techniques most used in enterprise applications intended to visualize one-to-many relationships, for example, let's suppose the following a common business scenario where we want to display a list of subcategories of products, and allow the user select a particular subcategory in order to display the list of associated products.
Developing the solution
In order to illustrate this data presentation technique, we're going to use the tables Production.Product (representing business entity Product) and Production.ProductSubcategory (representing the subcategories associated to products) in the database AdventureWorks shipped with SQL Server 2005. In our solution, we're going to display a list of master data (data from the Production.ProductSubcategory) in a DropDownList Web control and the details of the selected category in a GridView.
The first step is to create a Web application by opening Visual Studio.NET 2005 and select File | New | Web Site as the front-end project. Let's create a Class Library project for the data access layer components. This separation of objects (concerning their responsibilities) follows the best practices of enterprise applications development.
Let's add a strongly typed DataSet item to the Class Library project (see Figure 1).
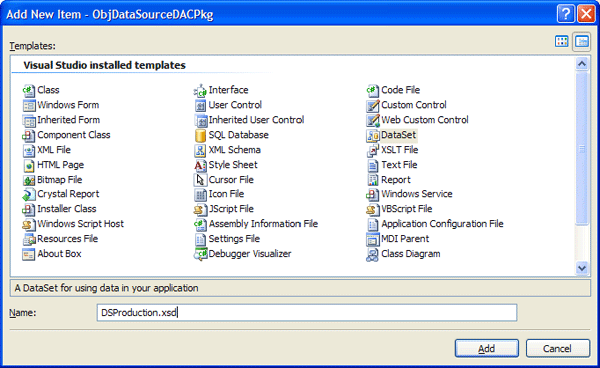
Figure 1
Let's define a TableAdapter item representing the logic to access the Production.Product table in the database AdventureWorks by using a SQL statement (see Figure 2).
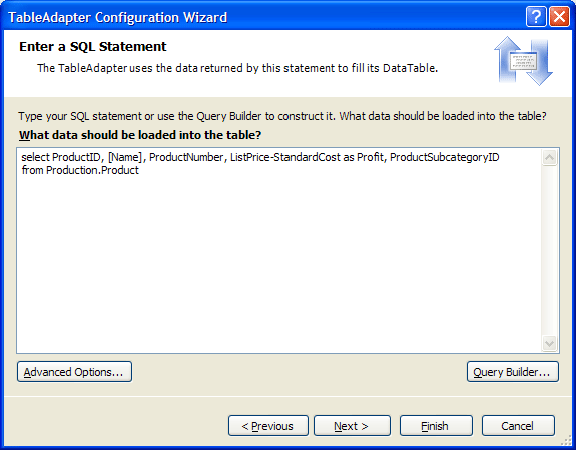
Figure 2
As well as let's define a TableAdapter item representing the logic to access the Production.ProductSubcategory table in the database AdventureWorks by using a SQL statement (see Figure 3).
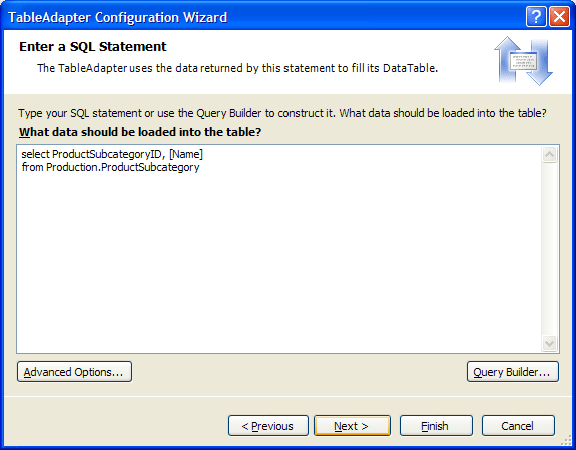
Figure 3
We need to add another query to get products by its underlying category (see Figure 4).
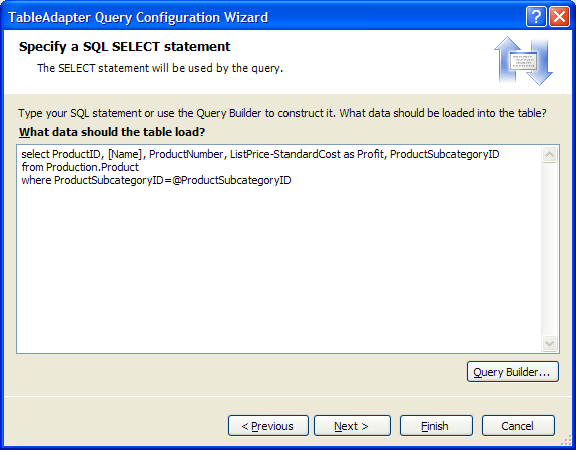
Figure 4
The result schema for the data access objects is shown in Figure 5.
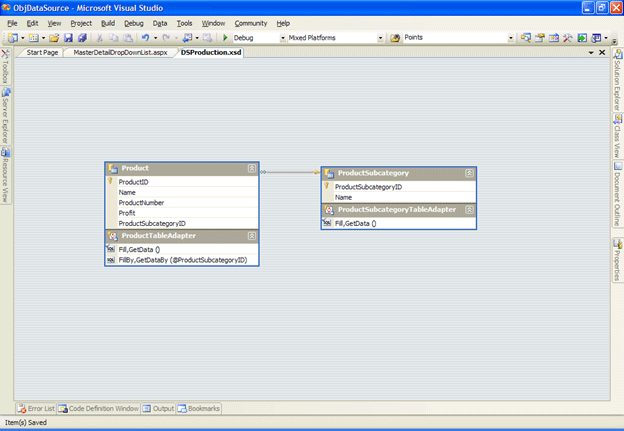
Figure 5
Now let's go to the Web front-end project and add a reference to the data access project (the Class Library project created before) and then add Web form to the project named MasterDetailDropDownList.aspx.
Now let's drag and drop a DropDownList control from the Toolbox onto the Web form, and set the ID property to ddlSubcategories.
Next drag and drop an ObjectDataSource (for the ProductSubcategory data source) from the Toolbox onto the Web form, set the ID property to odtSubcategories,click on the smart tag and select the Configure Data Source option in order to launch the data source configuration wizard. In the first page (Choose a Business Object) you must select the ProductSubcategoryTableAdapter (see Figure 6).
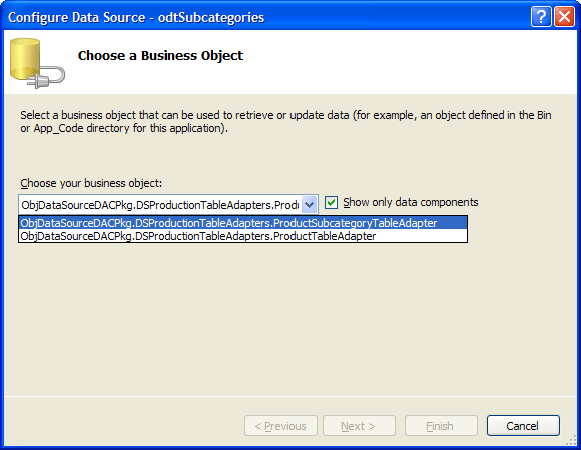
Figure 6
Click on the Next button and in the Define Data Methods page; we select the GetData method (see Figure 7).
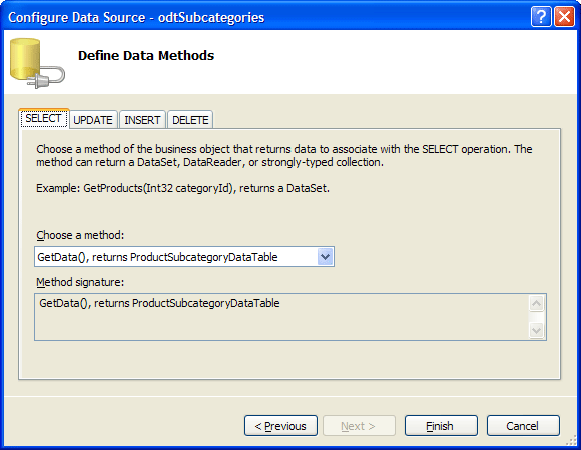
Figure 7
Click on the Finish button to finish the wizard. Now let's bind the ddlSubcategories DropDownList control to the odsSubcategories ObjectDataSource by clicking on the smart tag on the ddlSubcategories DropDownList control and selecting the Choose Data Source option and setting the fields in the wizard as shown (see Figure 8).
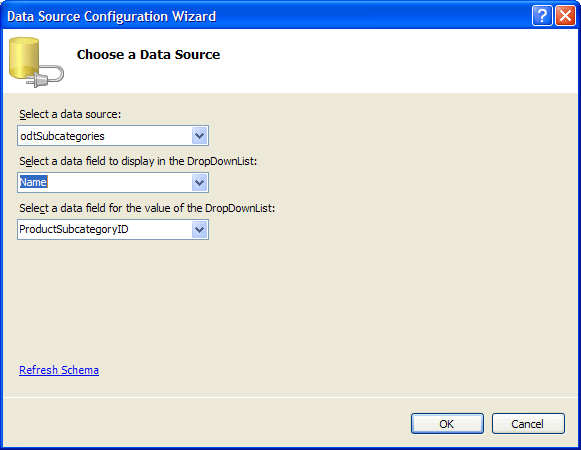
Figure 8
Now, we're going to define the data presentation control and data source for the Production.Product table. Drag and drop a GridView control from the Toolbox onto the Web form. Set the ID property to gvProducts. Next drag and drop an ObjectDataSource from the Toolbox onto the Web form, set the ID property to odtProducts, click on the smart tag and select the Configure Data Source option in order to launch the data source configuration wizard. In the first page (Choose a Business Object) you must select the ProductTableAdapter (see Figure 9).
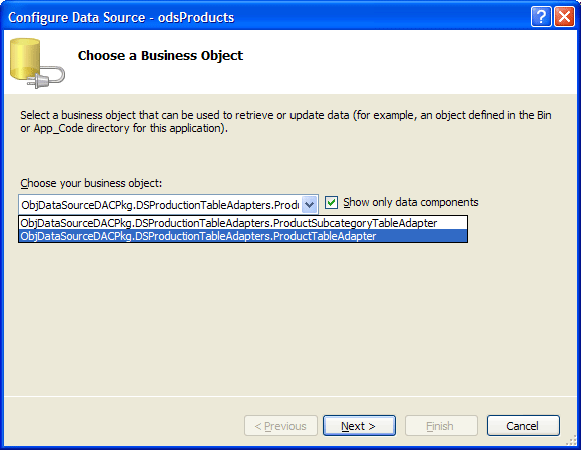
Figure 9
Click on the Next button and in the Define Data Methods page; we select the GetData method (see Figure 10).
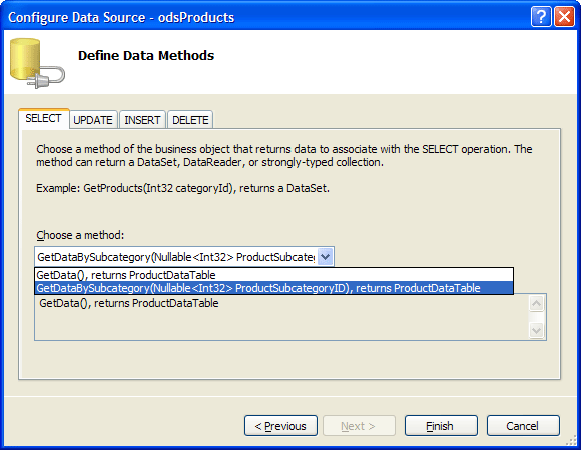
Figure 10
Click on the Next button, and the Define Parameters page appears. Select Control from the Parameter Source drop-down list and select ddlSubcategories from the ControlID drop-down list (see Figure 11).
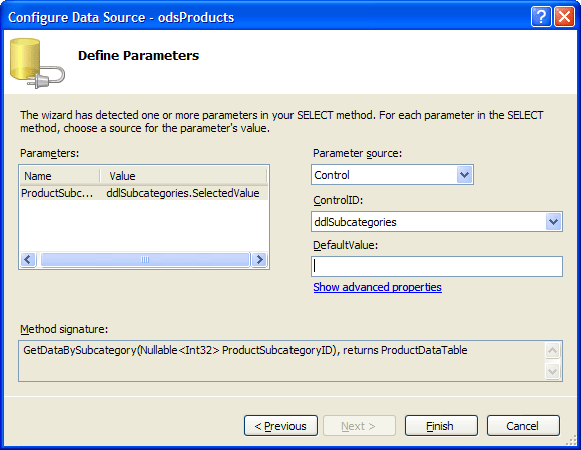
Figure 11
Click on the Finish button to finish with the wizard. Next step is to bind the odsProducts ObjectDataSource and the gvProducts GridView control by clicking on the smart tag on the GridView control and selecting Choose Data Source option (see Figure 12).
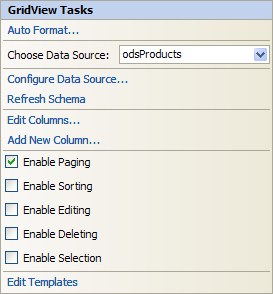
Figure 12
In order to update the changes in the DropDownList control onto the GridView control through the ObjectDataSource items, we need a postback to the server for executing the underlying logic. We can achieve this postback by setting the AutoPostBack property to True in the ddlSubcategories DropDownList control. This will trigger a postback whenever changes, such as selecting another item in the list, occur in this control (see Figure 13).
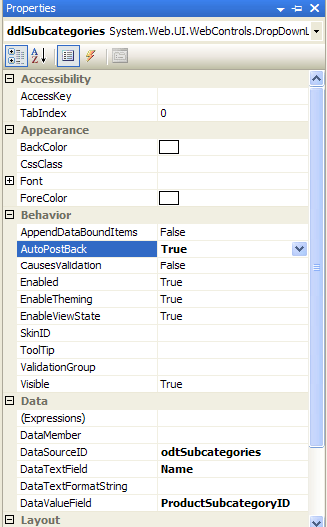
Figure 13
If you want to display a common message "Select a Subcategory" in the DropDownList ddlSubcategories, you must click on the smart tag on the DropDownList ddlSubcategories and select Edit Items option. The ListItem Collection Editor appears, and then we have to add a new item (see Figure 14).
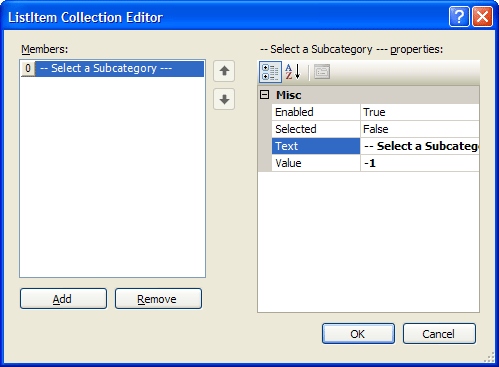
Figure 14
You must set the AppendDataBoundItems property to True in order not to override the manually entered items with the items from the data source (see Figure 15).
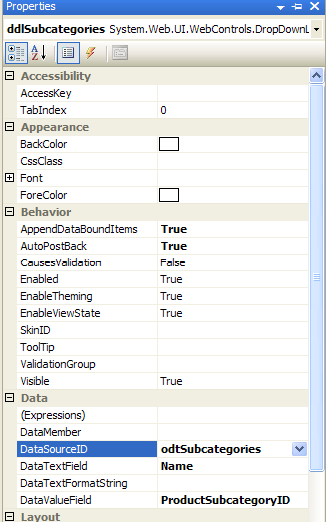
Figure 15
Conclusion
In this article, I've discussed the main techniques to visualize data using the master detail approach using ObjectDataSource items in ASP.NET 2.0.