Introduction
This article is intended to illustrate how to implement the master-detail presentation pattern using the ObjectDataSource components and two Web pages in ASP.NET 2.0. Master-detail presentation pattern is one of the techniques most used in enterprise applications intended to visualize one-to-many relationships, for example, let's suppose the following a common business scenario where we want to display a list of subcategories of products, and allow the user select a particular subcategory in order to display the list of associated products.
Developing the solution
In order to illustrate this data presentation technique, we're going to use the tables Production.Product (representing business entity Product) and Production.ProductSubcategory (representing the subcategories associated to products) in the database AdventureWorks shipped with SQL Server 2005. In our solution, we're going to display a list of master data (data from the Production.ProductSubcategory) in one Web page and through a reference; we're going to navigate to the details Web page to display the underlying products.
The first step is to create a Web application by opening Visual Studio.NET 2005 and select File | New | Web Site as the front-end project. Let's create a Class Library project for the data access layer components. This separation of objects (concerning their responsibilities) follows the best practices of enterprise applications development.
Let's add a strongly typed DataSet item to the Class Library project (see Figure 1).
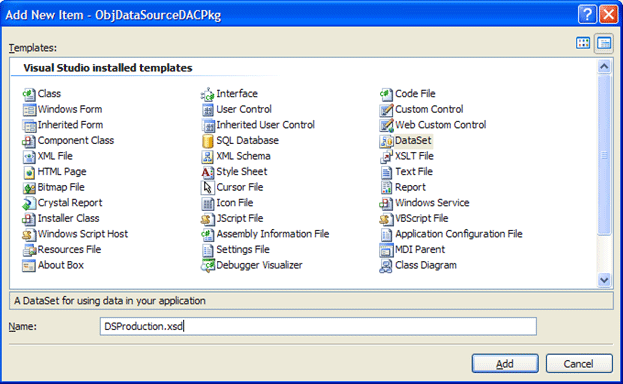
Figure 1
Let's define a TableAdapter item representing the logic to access the Production.Product table in the database AdventureWorks by using a SQL statement (see Figure 2).
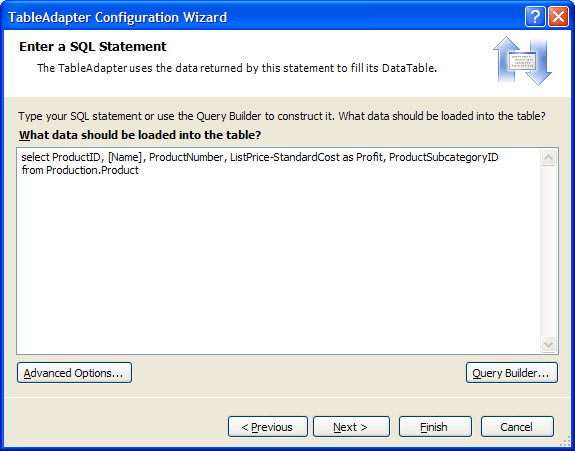
Figure 2
As well as let's define a TableAdapter item representing the logic to access the Production.ProductSubcategory table in the database AdventureWorks by using a SQL statement (see Figure 3).
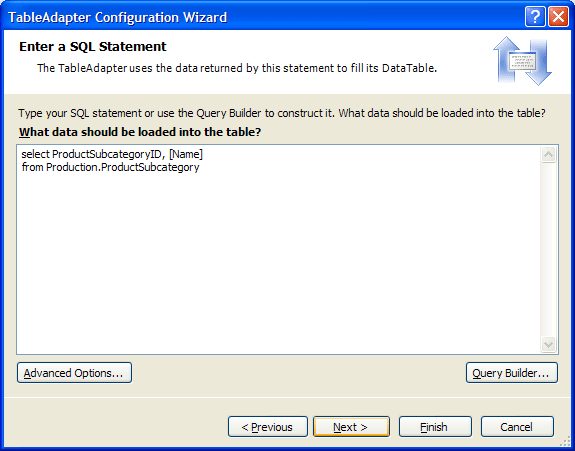
Figure 3
We need to add another query to get products by its underlying category (see Figure 4).
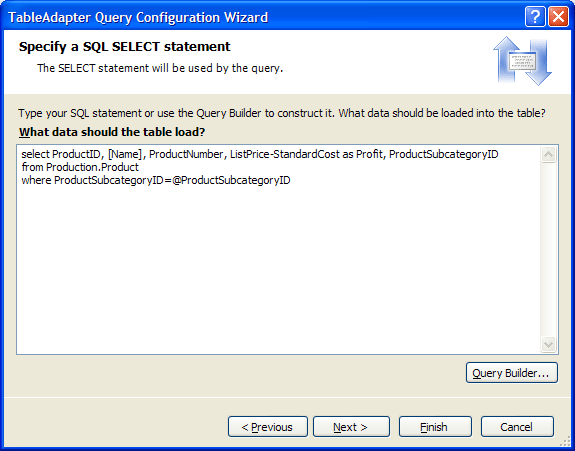
Figure 4
The result schema for the data access objects is shown in Figure 5.
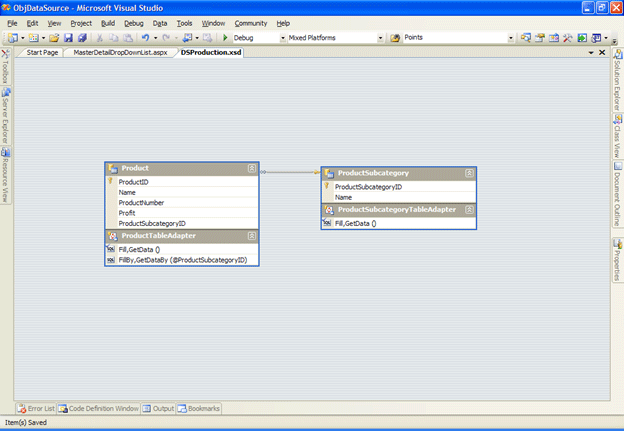
Figure 5
Now let's go to the Web front-end project and add a reference to the data access project (the Class Library project created before) and then add two Web form to the project. The first one is named ProductSubcategory.aspx to display a list of subcategories and second one is named ProductBySubcategory.aspx to display a list of products for the selected subcategory.
Now let's go to the ProductSubcategory.aspx page, drag and drop a GridView control from the Toolbox onto the Web form, and set the ID property to gvSubcategories.
Next drag and drop an ObjectDataSource (for the ProductSubcategory data source) from the Toolbox onto the Web form, set the ID property to odsSubcategories, click on the smart tag and select the Configure Data Source option in order to launch the data source configuration wizard. In the first page (Choose a Business Object) you must select the ProductSubcategoryTableAdapter (see Figure 6).
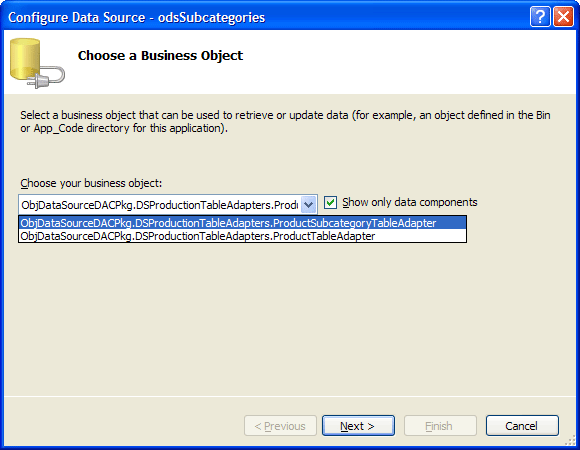
Figure 6
Click on the Next button and in the Define Data Methods page; we select the GetData method (see Figure 7).
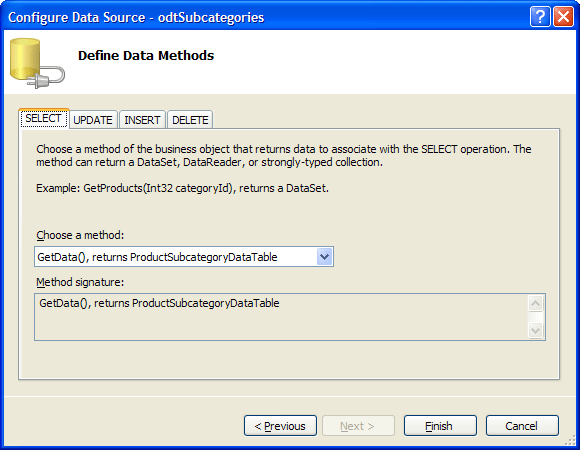
Figure 7
Click on the Finish button to finish the wizard. Now let's bind the gvSubcategories GridView control to the odsSubcategories ObjectDataSource by clicking on the smart tag on the gvSubcategories GridView control and selecting the Choose Data Source option and setting the fields in the windows as shown (see Figure 8).
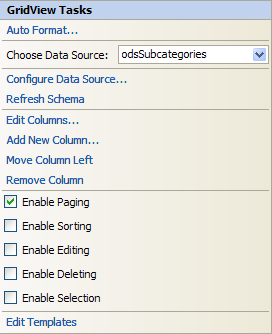
Figure 8
Because we need a link on the GridView that takes the user to the ProductBySubcategory.aspx page passing the ProductSubcategoryID value through a querystring to the page. Now, we have open the windows from the smart tag on the gvSubcategories GridView (see Figure 8) and click on the Edit Columns link, and select the HyperLinkField from the Available fields listbox, and then click on the Add button to add it to the Selected fields listbox (see Figure 9).
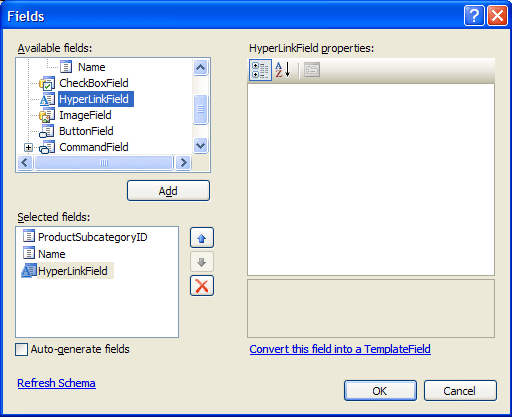
Figure 9
Now click on the HyperLinkField on the Selected fields listbox and now you can set the properties in the Property viewer on the right. Set the Text property to View Products. In order to build the URL with the querystrings necessary to be passed to the ProductBySubcategory.aspx, we have to use the DataNavigateUrlFields which represents a set of comma-delimited list of data fields whose values are passed to the URL to be formed. This URL is built using the DataNavigateUrlFormatString property which represents the static part of the URL plus a set of placeholders for the querystring values. If the URL is going to set multiple fields, we have to specify using {0} for the first field value inserted, {1} for the second one and so on. These values are supplied by the list of fields specified on the DataNavigateUrlFields property.
Now, applying these concepts to our solution, the DataNavigateUrlFields property is to ProductSubcategoryID and DataNavigateUrlFormatString property is set to ProductBySubcategory.aspx?ProductSubcategoryID={0} (see Figure 10).
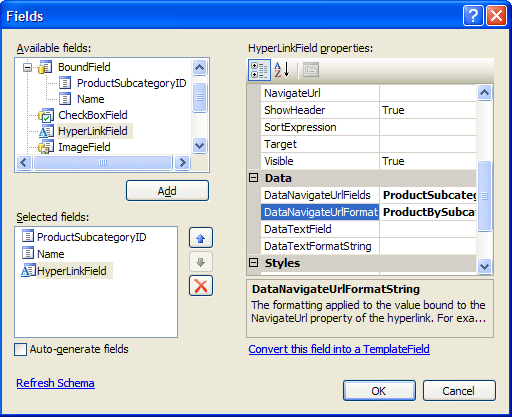
Figure 10
Now, let's go to the ProductBySubcategory.aspx page in order to define the data presentation for the list of products given the subcategory which is passed in the ProductSubcategoryID querystring.
Drag and drop a GridView control from the Toolbox onto the Web form. Set the ID property to gvProductsBySubcategory. Next drag and drop an ObjectDataSource from the Toolbox onto the Web form, set the ID property to odsProductsBySubcategory, click on the smart tag and select the Configure Data Source option in order to launch the data source configuration wizard. In the first page (Choose a Business Object) you must select the ProductTableAdapter (see Figure 11).
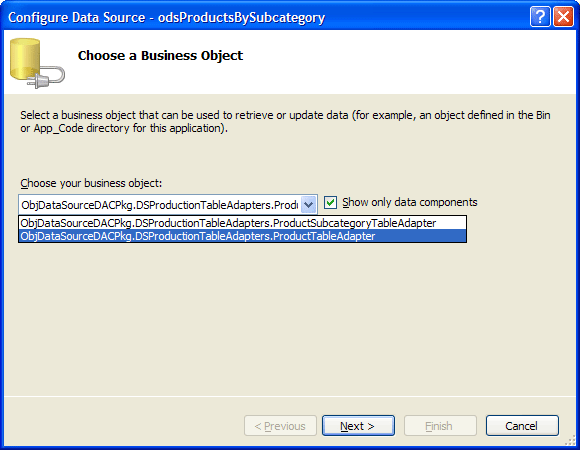
Figure 11
Click on the Next button and in the Define Data Methods page; we select the GetData method (see Figure 12).
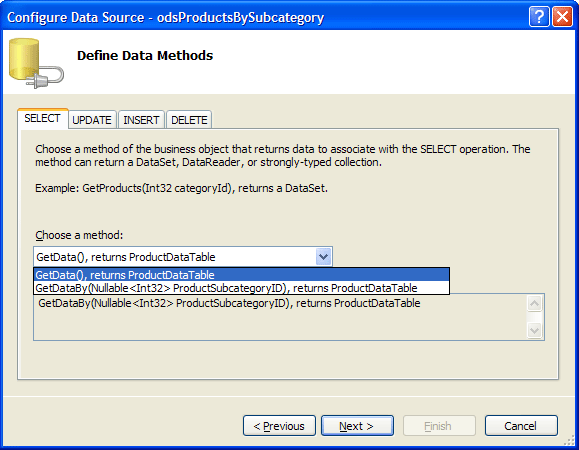
Figure 12
Click on the Next button, and the Define Parameters page appears. Select QueryString option from the Parameter Source drop-down list and set ProductSubcategoryID value to the QueryStringField field (see Figure 13).
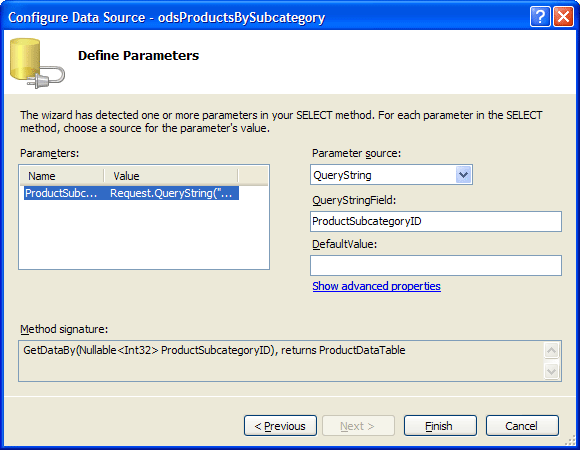
Figure 13
Click on the Finish button to finish with the wizard. Next step is to bind the odsProductsBySubcategory ObjectDataSource and the gvProductsBySubcategory GridView control by clicking on the smart tag on the GridView control and selecting Choose Data Source option (see Figure 14).
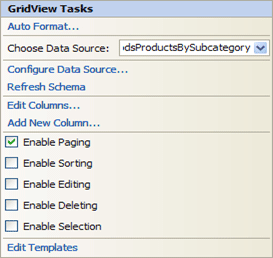
Figure 14
Conclusion
In this article, I've discussed the main techniques to visualize data using the master detail approach using two Web pages in ASP.NET 2.0; one for the master data and another for the detail data.