A CheckBox control allows users to select a single or multiple options from a list of options. In this article, I will discuss how to create a CheckBox control in Windows Forms at design-time as well as run-time. After that, I will continue discussing various properties and methods available for the CheckBox control.
Creating a CheckBox
We can create a CheckBox control using a Forms designer at design-time or using the CheckBox class in code at run-time (also known as dynamically).
To create a CheckBox control at design-time, you simply drag and drop a CheckBox control from Toolbox to a Form in Visual Studio. After you drag and drop a CheckBox on a Form, the CheckBox looks like Figure 1. Once a CheckBox is on the Form, you can move it around and resize it using mouse and set its properties and events.
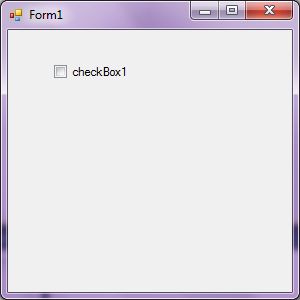
Figure 1
Creating a CheckBox control at run-time is merely a work of creating an instance of CheckBox class, set its properties and add CheckBox class to the Form controls.
First step to create a dynamic CheckBox is to create an instance of CheckBox class. The following code snippet creates a CheckBox control object.
// Create a CheckBox object
CheckBox dynamicCheckBox = new CheckBox();
In the next step, you may set properties of a CheckBox control. The following code snippet sets location, width, height, background color, foreground color, Text, Name, and Font properties of a CheckBox.
dynamicCheckBox.Left = 20;
dynamicCheckBox.Top = 20;
dynamicCheckBox.Width = 300;
dynamicCheckBox.Height = 30;
// Set background and foreground
dynamicCheckBox.BackColor = Color.Orange;
dynamicCheckBox.ForeColor = Color.Black;
dynamicCheckBox.Text = "I am a Dynamic CheckBox";
dynamicCheckBox.Name = "DynamicCheckBox";
dynamicCheckBox.Font = new Font("Georgia", 12);
Once a CheckBox control is ready with its properties, next step is to add the CheckBox control to the Form. To do so, we use Form.Controls.Add method. The following code snippet adds a CheckBox control to the current Form.
Controls.Add(dynamicCheckBox);
Setting CheckBox Properties
After you place a CheckBox control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
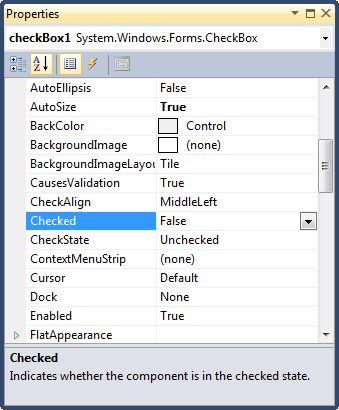
Figure 2
Location, Height, Width, and Size
The Location property takes a Point that specifies the starting position of the CheckBox on a Form. You may also use Left and Top properties to specify the location of a control from the left top corner of the Form. The Size property specifies the size of the control. We can also use Width and Height property instead of Size property. The following code snippet sets Location, Width, and Height properties of a CheckBox control.
// Set CheckBox properties
dynamicCheckBox.Location = new Point(20, 150);
dynamicCheckBox.Height = 40;
dynamicCheckBox.Width = 300;
Background, Foreground, BorderStyle
BackColor and ForeColor properties are used to set background and foreground color of a CheckBox respectively. If you click on these properties in Properties window, the Color Dialog pops up.
Alternatively, you can set background and foreground colors at run-time. The following code snippet sets BackColor and ForeColor properties.
// Set background and foreground
dynamicCheckBox.BackColor = Color.Red;
dynamicCheckBox.ForeColor = Color.Blue;
Name
Name property represents a unique name of a CheckBox control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a CheckBox control.
dynamicCheckBox.Name = "DynamicCheckBox";
string name = dynamicCheckBox.Name;
Text and TextAlign
Text property of a CheckBox represents the current text of a CheckBox control. The TextAlign property represents text alignment that can be Left, Center, or Right. The following code snippet sets the Text and TextAlign properties and gets the size of a CheckBox control.
dynamicCheckBox.Text = "I am a Dynamic CheckBox";
dynamicCheckBox.TextAlign = ContentAlignment.MiddleCenter;
Check Mark Alignment
CheckAlign property is used to align the check mark in a CheckBox. By using CheckAlign and TextAlign properties, we can place text and check mark to any position on a CheckBox we want. The following code snippet aligns check mark to middle-center and text to top-right and creates a CheckBox that looks like Figure 3.
dynamicCheckBox.CheckAlign = ContentAlignment.MiddleCenter;
dynamicCheckBox.TextAlign = ContentAlignment.TopRight;
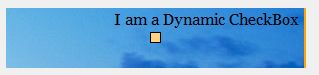
Figure 3
Font
Font property represents the font of text of a CheckBox control. If you click on the Font property in Properties window, you will see Font name, size and other font options. The following code snippet sets Font property at run-time.
dynamicCheckBox.Font = new Font("Georgia", 16);
Read CheckBox Contents
The simplest way of reading a CheckBox control contents is using the Text property. The following code snippet reads contents of a CheckBox in a string.
string CheckBoxContents = dynamicCheckBox.Text;
Appearance
Appearance property of CheckBox can be used to set the appearance of a CheckBox to a Button or a CheckBox. The following property makes a CheckBox look like a Button control.
dynamicCheckBox.Appearance = Appearance.Button;
AutoEllipsis
An ellipsis character (...) is used to give an impression that a control has more characters but it could not fit in the current width of the control. If AutoEllipsis property is true, it adds ellipsis character to a control if text in control does not fit. You may have to set AutoSize to false to see the ellipses character. The following code snippet sets the AutoEllipsis property of a CheckBox to true.
dynamicCheckBox.AutoEllipsis = true;
Image in CheckBox
The Image property of a CheckBox control is used to set the background as an image. The Image property needs an Image object. The Image class has a static method called FromFile that takes an image file name with full path and creates an Image object.
You can also align image and text. The ImageAlign and TextAlign properties of CheckBox are used for this purpose.
The following code snippet sets an image as a CheckBox background.
// Assign an image to the CheckBox.
dynamicCheckBox.Image = Image.FromFile(@"C:\Images\Dock.jpg");
// Align the image and text on the CheckBox.
dynamicCheckBox.ImageAlign = ContentAlignment.MiddleRight;
// Give the CheckBox a flat appearance.
dynamicCheckBox.FlatStyle = FlatStyle.Flat;
CheckBox States
A typical CheckBox control has two possible states – Checked and Unchecked. Checked state is when the CheckBox has check mark on and Unchecked is when the CheckBox is not checked. Typically, we use a mouse to check or uncheck a CheckBox.
Checked property is true when a CheckBox is in checked state.
dynamicCheckBox.Checked = true;
CheckState property represents the state of a CheckBox. It can be checked or unchecked. Usually, we check if a CheckBox is checked or not and decide to take an action on that state something like following code snippet.
if (dynamicCheckBox.Checked)
{
// Do something when CheckBox is checked
}
else
{
// Do something here when CheckBox is not checked
}
ThreeState is a new property added to the CheckBox in latest versions of Windows Forms. When this property is true, the CheckBox has three states - Checked, Unchecked, and Indeterminate. The following code snippet sets and checks CheckState.
dynamicCheckBox.CheckState = CheckState.Indeterminate;
if (dynamicCheckBox.CheckState == CheckState.Checked)
{
}
else if (dynamicCheckBox.CheckState == CheckState.Indeterminate)
{
}
else
{
}
AutoCheck property represents whether the Checked or CheckState values and the CheckBox's appearance are automatically changed when the CheckBox is clicked. By default this property is true but if set to false.
dynamicCheckBox.AutoCheck = false;
CheckBox Checked Event Hander
CheckedChanged and CheckStateChanged are two important events for a CheckBox control. The CheckedChanged event occurs when the value of the Checked property changes. The CheckStateChanged event occurs when the value of the CheckState property changes.
To add these event handlers, you go to Events window and double click on CheckedChanged and CheckedStateChanged events as you can see in Figure 4.
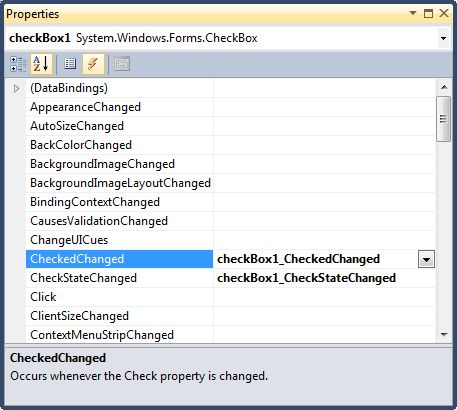
Figure 4
The following code snippet defines and implements these events and their respective event handlers.
dynamicCheckBox.CheckedChanged += new System.EventHandler(CheckBoxCheckedChanged);
dynamicCheckBox.CheckStateChanged += new System.EventHandler(CheckBoxCheckedChanged);
private void CheckBoxCheckedChanged(object sender, EventArgs e)
{
}
private void CheckBoxCheckedChanged(object sender, EventArgs e)
{
}
Summary
In this article, we discussed discuss how to create a CheckBox control in Windows Forms at design-time as well as run-time. After that, we saw how to use various properties and methods.