This article has been excerpted from book "A Programmer's Guide to ADO.NET in C#".
I'll show you how to develop database applications using ADO.NET and ASP.NET. To start creating your first ADO.NET application, you'll create a Web Application project as you did in the previous section. In this example you're adding only a List Box control to the page and you're going to read data from a database and display the data in the list box.
After dragging a list Box control from the Web Forms control toolbox and dropping it on the page, write the code in Listing 7-2 on the Page_Load event. You can add a Page_Load event either double clicking on the page or using the Properties window.
Note: If you're using an access 2000 database and OleDb data providers, don't forget to add reference to the System.Data.OleDb namespace to your project.
Listing 7-2. Filling data from a database to a ListBox control
using System;
using System.Collections;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.HtmlControls;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Xml.Linq;
using System.Data.OleDb;
namespace firstADO
{
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
// Put user code to initialize the page here
// Create a connection object
string ConnectionString = @"Provider = Microsoft.Jet.OLEDB.4.0;" +
" Data source =c:/Northwind.mdb";
OleDbConnection conn = new OleDbConnection(ConnectionString);
// open the connection
if (conn.State != ConnectionState.Open)
conn.Open();
// create a data adapter
OleDbDataAdapter da = new OleDbDataAdapter
("Select customerID From customers", conn);
//Create and fill a dataset
DataSet ds = new DataSet();
da.Fill(ds);
// Bind dataset to the control
// Set DataSource property of ListBox as DataSet's DefaultView
ListBox1.DataSource = ds;
ListBox1.SelectedIndex = 0;
// Set Field Name You want to get data from
ListBox1.DataTextField = "customerID";
DataBind();
// Close the connection
if (conn.State == ConnectionState.Open)
conn.Close();
}
}
}
As you can see, this code looks familiar. First you create a connection object with the Northwind.mdb database. After that you create a data adapter and select FirstName, LastName and Title from the Employees table. Then you create a dataset object and fill it using the data adapter's Fill method. Once you have a dataset, you set the dataset as the ListBox's DataSource property and set SelectIndex as 0. The SelectIndex property represents the index of the column from a dataset you want to display in the control. The field name of your column is FirstName. At the end you call the DataBind method of the ListBox. This method binds the data to the list box.
The output like figure 7-17. As you can see the ListBox control displays data from FirstName column of the Employees table.
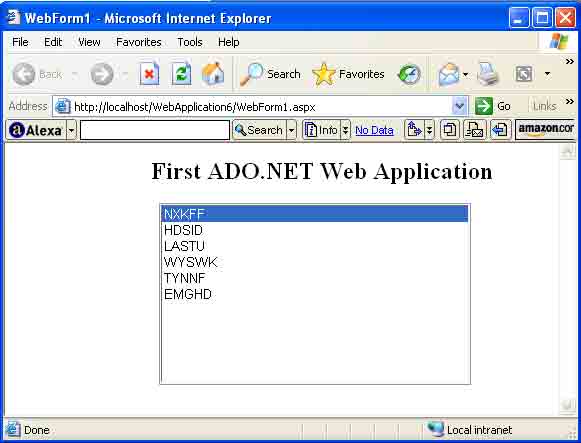
Figure 7-17. Your first ADO.NET Web application
Conclusion
Hope this article would have helped you in understanding Creating Your First ADO.NET Web Application. See other articles on the website also for further reference.
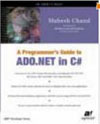 |
This essential guide to Microsoft's ADO.NET overviews C#, then leads you toward deeper understanding of ADO.NET. |