This article has been excerpted from book "A Programmer's Guide to ADO.NET in C#".
Now you'll create your first sample using data adapters. In this sample example, I'll show you how to create data adapters using Sql and OleDb data providers and fill data from data from data adapter to a DataGrid control.
First, create a Windows application using Visual C# projects and add two buttons and a DataGrid control to the form by dragging the controls form the toolbox to the form. Second, set both button's Name property; use OleDbDataAdapter and SqlDataAdapter. Next set the properties to OleDbData Adapter and SQL DataAdapter. After setting these properties, the form will look like figure 5-40. As you can see, there are two buttons, OleDbDataAdapter and SQL DataAdapter.
Now add button-click event handlers for both the OleDbDataAdapter and SQL DataAdapter buttons. You can add a button-click event handler either by double-clicking on the button or by using the Events tab of the properties window of a button control. On the OleDb DataAdapter button-click event handler, you'll write code to read data from an OleDb data source and fill data to the data grid. On the SQL DataAdapter button-click event handler, you'll write code to read data from a SQL Server datasource and fill data to the data grid.
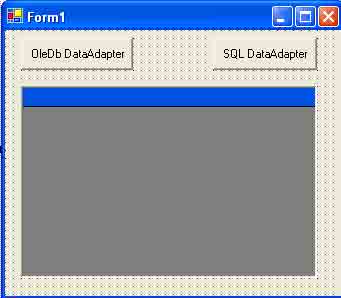
Figure 5-40. Creating a Windows Forms application and adding controls to the form
Listing 5-44 shows the source code for the OleDb DataAdapter button click, and Listing 5-44 shows the source code for the SQL DataAdapter button click, and Listing 5-45 shows the source code for the SQL DataAdatper button click. As you can see, you follow the same steps as before. Open a connection, create a data adapter object with a SELECT string, create a dataset object, call data adapter's FILL method to fill the dataset, and bind the dataset to the DataGrid.DataSource property as DataSet.DefaultViewManager, which represents the default view of a DataSet object.
Listing 5-44. Displaying the Order table data in a Data Grid
using OleDbDataAdapter;
private void OleDbDataAdapter_Click(object sender, System.Event Args e)
{
//Create a connection object
string ConnectionString = @"provider=Microsoft.Jet.OLEDB.4.0;" +
"Data Source= C:/northwind.mdb";
string SQL = "SELECT * FROM Orders";
OleDbConnection conn = new OleDbConnection(ConnectionString);
// open the connection
conn.Open( );
// Create an OleDbDataAdapter object
OleDbDataAdapter adapter = new OleDbDataAdapter();
adapter.SelectCommand = new OleDbCommand(SQL, conn);
// Create Data Set object
DataSet ds = new DataSet("orders");
// Call DataAdapter's Fill method to fill data from the
// DataAdapter to the DataSet
adapter.Fill(ds);
// Bind dataset to a DataGrid control
dataGrid1.DataSource = ds.DefaultViewManager;
}
The output of Listing 5-44 looks like figure 5-41.
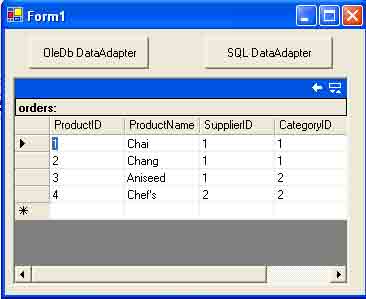
Figure 5-41. Filling data from an Access database to a DataGrid control using OleDbDataAdapter
The output of listing looks like figure 5-42.
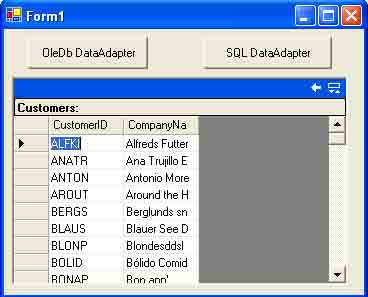
Figure 5-42. Filling data from a SQL server database to a DataGrid control using SqlDataAdapter
Listing 5-45. Displaying the Customers tables data in a DataGrid
using SqlDataAdapter;
private void SqlDataAdapter_Click(object sender, System.EventArgs e)
{
string ConnectionString = "Integrated Security = SSPI;" +
"Initial catalog = Northwind;" + " Data Source =MAIN-SERVER; ";
string SQL = "SELECT CustomerID, CompanyName FROM Customers";
SqlConnection conn = new SqlConnection(ConnectionString);
// open the connection
conn.Open( );
//Create a SqlDataAdapter object
SqlDataAdapter adapter = new SqlDataAdapter(SQL, conn);
// Call DataAdapter's Fill method to fill data from the
// Data Adapter to the DataSet
DataSet ds = new DataSet("Customers");
adapter.Fill(ds);
// Bind data set to a DataGrid control
dataGrid1.DataSource = ds.DefaultViewManager;
}
Conclusion
Hope this article would have helped you in understanding DataAdapter Example in ADO.NET. See my other articles on the website on ADO.NET.
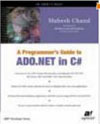 |
This essential guide to Microsoft's ADO.NET overviews C#, then leads you toward deeper understanding of ADO.NET. |