This article has been excerpted from book "Graphics Programming with GDI+".
A region describes the interior of a closed graphics shape, or form. A form has two areas: a nonclient area and a client area. The nonclient area (which does not allow for user-drawn graphics objects) includes the title bar- and depending on the application, horizontal and vertical scroll bars. This area cannot be used to draw graphics objects. The client area is used to draw controls and graphics objects.
In the .NET Framework library, the Region class object represents a region. If you have ever developed a complex .NET graphics application that requires a lot of rendering, you may have used this object a lot.
Constructing a Region Object
The Region class provides five overloaded forms. Using these forms, you can construct a Region object from a Rectangle, RectangleF, GraphicsPath, or RegionData object, or will no parameters. The following code snippet creates Region objects in different ways using different arguments.
//Create two rectangles
Rectangle rect1 = new Rectangle(20, 20, 60, 80);
RectangleF rect2 = new RectangleF(100, 20, 60, 100);
//Create a graphics path
GraphicsPath path = new GraphicsPath();
//Add a rectangle to the graphics path
path.AddRectangle(rect1);
//Create a region from rect1
Region rectRgn1 = new Region(rect1);
//Create a region from rect2
Region rectRgn2 = new Region(rect2);
//Create a region from GraphicsPath
Region pathRgn = new Region(path);
The Region class has no properties. After constructing a region, an application can use the Graphics class's FillRegion method to fill the region.
Table 6.1 describes the methods of the Region class briefly.
Table 6.1: Region method
Method |
Description |
Clone |
Creates an exact copy of a region. |
Complement |
Updates a region to the portion of a rectangle that does not intersect with the region. |
Exclude |
Updates a region to the portion of its interior that does not intersect with a rectangle. |
FormHrgn |
Creates a new Region object from a handle to the specified existing GDI region. |
GetBounds |
Returns a RectangleF structure that represents a rectangle that bounds a region. |
GetHrgn |
Returns a window handle for a region. |
GetRegionData |
Returns a RegionData object for a region. RegionData contains information describing a region. |
GetRegionScans |
Returns an array of RectangleF structures that approximate a region. |
Intersect |
Updates a region to the intersection of itself with another region. |
IsEmpty |
Returns true if a region is empty; otherwise return false. |
IfInfinite |
Returns true if a region has an infinite interior; otherwise returns false. |
IsVisible |
Returns true if the specified rectangle is contained within a region. |
MakeEmpty |
Mark a region as empty. |
MakeInfinite |
Marks a region as infinite. |
Transform |
Applies the transformation matrix to the region. |
Translate |
Offsets the coordinates of a region by the specified amount. |
Union |
Updates a region to the union of itself and the given graphics path. |
Xor |
Updates a region to the union minus the intersection of itself with the given graphics path. |
Conclusion
Hope the article would have helped you in understanding the Region Class in GDI+. Read other articles on GDI+ on the website.
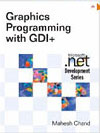 |
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |