As we know Web pages are based on the HTTP Protocol which is a stateless protocol, means that there is no information about the request, such as where they are coming from i.e. whether the request is from the same client or from a new client. Since the page is created each time, the page is requested then destroyed. So we can say that the state is not maintained between client requests by default.
ASP.NET developers use various available technologies for State Management. We can classify them as client side state management or server side state management.
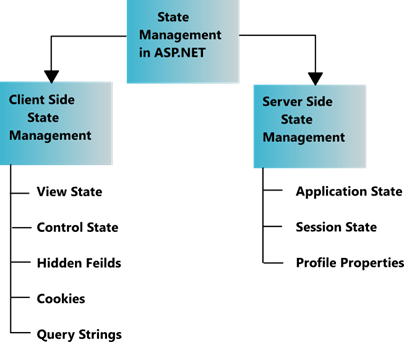
CLIENT SIDE STATE MANAGEMENT:
View State:
ViewState is an approach to saving data for the user. Because of the stateless nature of web pages, regular page member variables will not maintain their values across postbacks. ViewState is the mechanism that allows state values to be preserved across page postbacks and by default, EnableViewState property will be set to true. In a nutsheell, ViewState:
- Stores values per control by key name, like a Hashtable.
- Tracks changes to a View State value's initial state.
- Serializes and Deserializes saved data into a hidden form field on the client.
- Automatically restores View State data on postbacks.
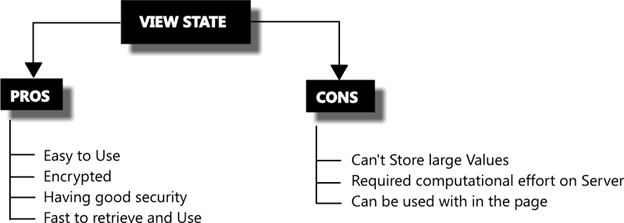
Sample:
//To Save Information in View State
ViewState.Add ("NickName", "Dolly");
//Retrieving View state
String strNickName = ViewState ["NickName"];
Here is a simple example of using the "ViewState" property to carry values between postbacks.
Code Example:
public int SomeInteger {
get {
object o = ViewState["SomeInteger"];
if (o != null) return (int)o;
return 0;
//a default
}
set { ViewState["SomeInteger"] = value; }
}
Control State:
The purpose of the control state repository is to cache data necessary for a control to properly function. ControlState is essentially a private ViewState for your control only, and it is not affected when ViewState is turned off. ControlState is used to store small amounts of critical information. Heavy usage of ControlState can impact the performance of application because it involves serialization and deserialization for its functioning.
There are two methods you have to implement in your custom control.
-
Load Control State
-
Save Control State
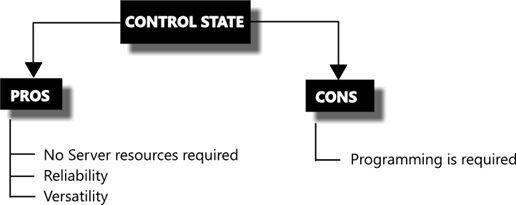
Code Example:
public class ControlStateWebControl : Control
{
#region Members
private string _strStateToSave;
#endregion
#region Methods
protected override void OnInit(EventArgs e)
{
Page.RegisterRequiresControlState(this);
base.OnInit(e);
}
protected override object SaveControlState()
{
return _strStateToSave;
}
protected override void LoadControlState(object state)
{
if (state != null)
{
_strStateToSave = state.ToString();
}
}
#endregion
}
Hidden Fields:
A Hidden control is the control which does not render anything on the web page at client browser but can be used to store some information on the web page which can be used on the page. Hidden fields are used to store data at the page level. These fields are not rendered by the browser, rather it's just like a standard control for which you can set its properties. If you use hidden fields, it is best to store only small amounts of frequently changed data on the client.
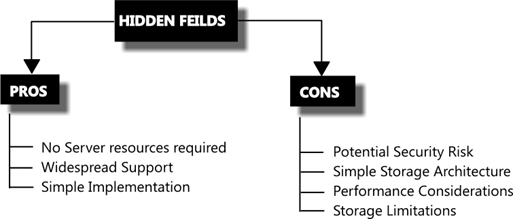
Sample:
//Declaring a hidden variable
protected HtmlInputHidden hidNickName;
//Populating hidden variable
hidNickName.Value = "Page No 1";
//Retrieving value stored in hidden field.
string str = hidNickName.Value;
Code Example:
protected void Page_Load(object sender, EventArgs e)
{
if(!IsPostBack)|
Label1.Text = string.Format("Clicked {0} times", HiddenField1.Value);
}
protected void Button1_Click(object sender, EventArgs e)
{
HiddenField1.Value = (Convert.ToInt32(HiddenField1.Value) + 1).ToString();
Label1.Text = string.Format("Clicked {0} times", HiddenField1.Value);
}
Cookies:
A cookie is a small amount of data which is either stored at client side in text file or in memory of the client browser session. Cookies are always sent with the request to the web server and information can be retrieved from the cookies at the web server. Every time a user visits a website, cookies are retrieved from the user machine and help identify the user.
Cookies are useful for storing small amounts of frequently changed information on the client. The information is sent with the request to the server.
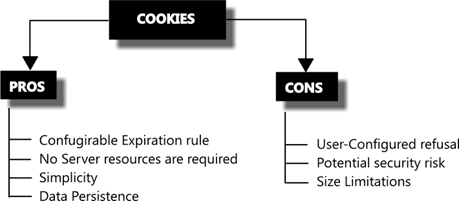
Sample:
// Creating a cookie
myCookie.Values.Add("muffin", "chocolate");
myCookie.Values.Add("babka", "cinnamon");
// Adding Cookie to Collection
Response.Cookies.Add(myCookie);
// Getting Values stored in a cookie
Response.Write(myCookie["babka"].ToString());
// Setting cookie path
myCookie.Path = "/forums";
// Setting domain for a cookie
myCookie.Domain = "forums.geekpedia.com";
// Deleting a cookie
myCookie.Expires = DateTime.Now.AddDays(-1);
Code Example:
//Storing value in cookie
HttpCookie cookie = new HttpCookie("NickName");
cookie.Value = "David";
Request.Cookies.Add(cookie);
//Retrieving value in cookie
if (Request.Cookies.Count > 0 && Request.Cookies["NickName"] != null)
lblNickName.Text = "Welcome" + Request.Cookies["NickName"].ToString();
else
lblNickName.Text = "Welcome Guest";
Query String:
A Query string is used to pass the values or information form one page to another page. They are passed along with URL in clear text. Query strings provide a simple but limited way of maintaining some state information. When surfing the internet you should have seen weird internet addresses such as:
http://www.localhost.com/Webform2.aspx?name=ABC&lastName=XYZ
This HTML address uses a QueryString property to pass values between pages.
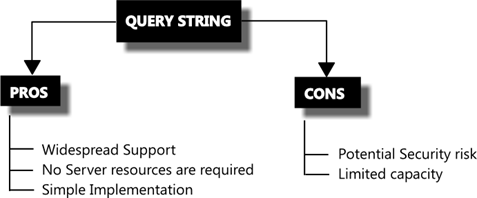
Syntax:
Request.QueryString(variable)[(index)|.Count]
Code Example:
using System;
using System.Web.UI;
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
string v = Request.QueryString["param"];
if (v != null)
{
Response.Write("param is ");
Response.Write(v);
}
string x = Request.QueryString["id"];
if (x != null)
{
Response.Write(" id detected");
}
}
}
SERVER SIDE STATE MANAGEMENT:
Application State:
Application State is used to store information which is shared among users of the ASP.Net web application. Application state is stored in the memory of the windows process which is processing user requests on the web server. Application state is useful in storing a small amount of often-used data. If application state is used for such data instead of frequent trips to the database, then it increases the response time/performance of the web application.
In classic ASP, an application object is used to store connection strings. It's a great place to store data that changes infrequently.
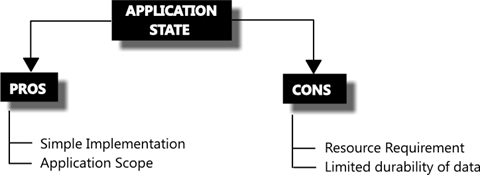
Code Example 1:
//Stroing information in application state
lock (this)
{
Application["NickName"] = "Nipun";
}
//Retrieving value from application state
lock (this)
{
string str = Application["NickName"].ToString();
}
Code Example 2:
protected void Page_Load(object sender, EventArgs e)
{
// Code that runs on page load
Application["LoginID"] = "Nipun";
Application["DomainName"] = "www.nipun.com";
}
Session State:
ASP.NET Session state provides a place to store values that will persist across page requests. Values stored in Session are stored on the server and will remain in memory until they are explicitly removed or until the Session expires. It is defined as the period of time that a unique user interacts with a Web application. Session state is a collection of objects, tied to a session stored on a server.
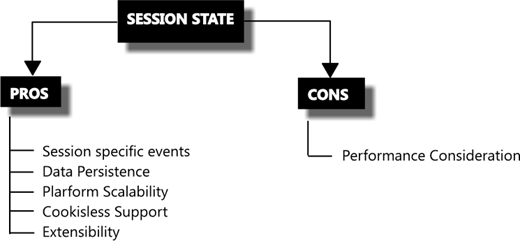
Sample:
//Storing informaton in session state
Session["NickName"] = "ABC";
//Retrieving information from session state
string str = Session["NickName"];
Code Example:
object sessionObject = Session["someObject"];
if (sessionObject != null)
{
myLabel.Text = sessionObject.ToString();
}
Profile Properties:
ASP.NET provides a feature called profile properties, which allows you to store user-specific data. It is similar to session state, except that unlike session state, the profile data is not lost when a user's session expires. The profile properties feature uses an ASP.NET profile, which is stored in a persistent format and associated with an individual user. In this each user has its own profile object.
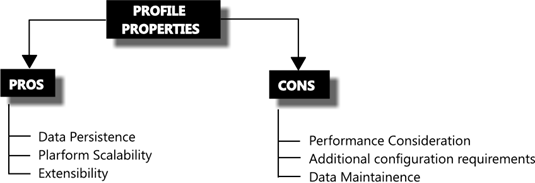
Sample:
<profile>
<properties>
<add item="item name" />
</properties>
</profile>
Code Example:
<authentication mode="Windows" />
<profile>
<properties>
<add name="FirstName"/>
<add name="LastName"/>
<add name="Age"/>
<add name="City"/>
</properties>
</profile>