This article has been excerpted from book "The Complete Visual C# Programmer's Guide from the Authors of C# Corner".
You can search for the occurrence of a value in an array with the IndexOf and LastIndexOf member functions. IndexOf starts the search from a lower subscript and moves forward, and LastIndexOf starts the search from an upper subscript and moves backwards. Both functions achieve a linear search, visiting each element sequentially until they find the match forward or backward.
Listing 20.13 illustrates a linear search through an array using the IndexOf and LastIndexOf methods.
Listing 20.13: Array Linear Search
// Linear Search
using System;
public class LinearSearcher
{
public static void Main()
{
String[] myArray = new String[7] { "kama", "dama", "lama", "yama", "pama", "rama", "lama" };
String myString = "lama";
Int32 myIndex;
// Search for the first occurrence of the duplicated value in a section of the
myIndex = Array.IndexOf(myArray, myString, 0, 6);
Console.WriteLine("The first occurrence of \"{0}\" between index 0 and index 6 is at index {1}.", myString, myIndex);
// Search for the last occurrence of the duplicated value in a section of the
myIndex = Array.LastIndexOf(myArray, myString, 6, 7);
Console.WriteLine("The last occurrence of \"{0}\" between index 0 and index 6 is at index {1}.", myString, myIndex);
Console.ReadLine();
}
}
The program in Listing 20.13, has this output:
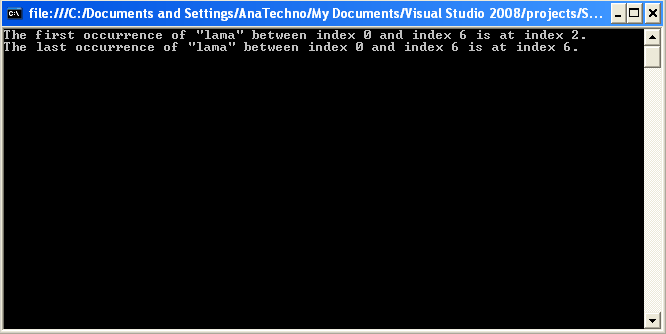
The String class provides methods for sorting, searching, and reversing that are easy to use. Note that Sort, BinarySearch, and Reverse are all static functions and are used for single-dimensional arrays.
Listing 20.14 illustrates usage of the Sort, BinarySearch, and Reverse functions.
Listing 20.14: Array Sort, Binarysearch, and Reverse Examples
// Binary Search
// Note an EXCEPTION occurs if the search element is not in the list
// We leave adding this functionality as homework!
using System;
class linSearch
{
public static void Main()
{
int[] a = new int[3];
Console.WriteLine("Enter number of elements you want to hold in the array (max3)?");
string s = Console.ReadLine();
int x = Int32.Parse(s);
Console.WriteLine("--------------------------------------------------");
Console.WriteLine("\n Enter array elements \n");
Console.WriteLine("--------------------------------------------------");
for (int i = 0; i < x; i++)
{
string s1 = Console.ReadLine();
a[i] = Int32.Parse(s1);
}
Console.WriteLine("Enter Search element\n");
Console.WriteLine("--------------------------------------------------");
string s3 = Console.ReadLine();
int x2 = Int32.Parse(s3);
// Sort the values of the Array.
Array.Sort(a);
for (int i = 0; i < x; i++)
{
Console.WriteLine("--------------Sorted-------------------------");
Console.WriteLine("Element{0} is {1}", i + 1, a[i]);
}
// BinarySearch the values of the Array.
int x3 = Array.BinarySearch(a, (Object)x2);
Console.WriteLine("--------------------------------------------------");
Console.WriteLine("BinarySearch: " + x3);
Console.WriteLine("Element{0} is {1}", x3, a[x3]);
Console.WriteLine("--------------------------------------------------");
// Reverse the values of the Array.
Array.Reverse(a);
Console.WriteLine("-----------Reversed-------------------------------");
for (int i = 0; i < x; i++)
{
Console.WriteLine("----------------------------------------------");
Console.WriteLine("Element{0} is {1}", i + 1, a[i]);
}
}
}
Listing 20.15 is a more sophisticated example of using the IComparer interface and Sort function together. The IComparer interface allows you to define a Compare method in order to do a comparison between two elements of your array. This Compare method is called repeatedly by the Sort function in order to sort the array. Listing 20.15 defines a Compare method that does a comparison between two strings.
Listing 20.15: Array Sorting
// sort an array according to the Nth element
using System;
using System.Collections;
public class CompareX : IComparer
{
int compareFrom = 0;
public CompareX(int i)
{
compareFrom = i;
}
public int Compare(object a, object b)
{
return String.Compare(a.ToString().Substring(compareFrom),
b.ToString().Substring(compareFrom));
}
}
public class ArrListEx
{
ArrayList arr = new ArrayList();
public ArrListEx()
{
arr.Add("aaaa9999");
arr.Add("bbbb8888");
arr.Add("cccc7777");
arr.Add("dddd6666");
arr.Sort(new CompareX(4));
IEnumerator arrList = arr.GetEnumerator();
while (arrList.MoveNext())
{
Console.WriteLine("Item: {0}", arrList.Current);
}
}
public static void Main(string[] args)
{
new ArrListEx();
}
}
Conclusion
Hope this article would have helped you in understanding Sorting, Reversing, and Searching in Arrays in C#. See other articles on the website on .NET and C#.
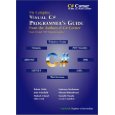 |
The Complete Visual C# Programmer's Guide covers most of the major components that make up C# and the .net environment. The book is geared toward the intermediate programmer, but contains enough material to satisfy the advanced developer. |