The GetThumbnailImage method of the Bitmap class in GDI+ and C# is used to create a small thumbnail image of a lange image. The following code snippet/page shows how to create an image thumbnail using Bitmap.GetThumbnailImage method.
using System;
using System.Data;
using System.Configuration;
using System.Collections;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Drawing;
using System.IO;
using System.Text;
using System.Net.Mail;
using System.Text.RegularExpressions;
using System.Threading;
public partial class _Default : System.Web.UI.Page
{
private string Finalimagename = "";
protected void Page_Load(object sender, EventArgs e)
{
}
protected void btnUpload1_Click(object sender, EventArgs e)
{
ImageUpload();
}
private void ImageUpload()
{
/* this code used to make directory name RealImage */
Directory.CreateDirectory(Server.MapPath("RealImage\\"));
HtmlInputFile htmlFile = (HtmlInputFile)BrowseImage0;
if (htmlFile.PostedFile.ContentLength > 0)
{
string sFormat = String.Format("{0:#.##}", (float)htmlFile.PostedFile.ContentLength / 2048);
if (float.Parse(sFormat) < float.Parse("2048"))
{
if (htmlFile.PostedFile != null)
{
ViewState["ImageName"] = htmlFile.PostedFile.FileName.Substring(htmlFile.PostedFile.FileName.LastIndexOf("\\") + 1);//browseImagePath[0];
}
else
{
ViewState["ImageName"] = "";
}
}
else
{
lblError1.Visible = true;
lblError1.Text = "Image Size is Large, please resize it !!";
}
}
else
{
ViewState["ImageName"] = "";
if (ViewState["ImageName"].ToString() == "")
{
lblError1.Visible = true;
lblError1.Text = "Attach an image to upload";
}
return;
}
//This function is used to make image thumbnail
MakeThumbnail();
}
//Return thumbnail callback
public bool ThumbnailCallback()
{
return true;
}
//For image thumbnial
private void MakeThumbnail()
{
System.Drawing.Image myThumbnail150;
object obj = new object();
obj = BrowseImage0;
System.Drawing.Image.GetThumbnailImageAbort myCallback = new System.Drawing.Image.GetThumbnailImageAbort(ThumbnailCallback);
HtmlInputFile hFile = (HtmlInputFile)obj;
if (hFile.PostedFile != null && hFile.PostedFile.ContentLength > 0)
{
//this code used to remove some symbols between image name and replace with space
string imgname1 = hFile.PostedFile.FileName.Replace('%', ' ').Substring(hFile.PostedFile.FileName.LastIndexOf("\\") + 1);
string imgname2 = imgname1.Replace('#', ' ').Substring(imgname1.LastIndexOf("\\") + 1);
string imgname3 = imgname2.Replace('@', ' ').Substring(imgname1.LastIndexOf("\\") + 1);
string imgname4 = imgname3.Replace(',', ' ').Substring(imgname1.LastIndexOf("\\") + 1);
string imgname5 = imgname4.Replace('&', ' ').Substring(imgname1.LastIndexOf("\\") + 1);
Finalimagename = imgname5.ToString();
string imgname = hFile.PostedFile.FileName.Substring(hFile.PostedFile.FileName.LastIndexOf("\\") + 1);
string sExtension = imgname.Substring(imgname.LastIndexOf(".") + 1);
//this code is used to check image extension
if (sExtension.ToLower() == "jpg" || sExtension.ToLower() == "gif" || sExtension.ToLower() == "bmp" || sExtension.ToLower() == "jpeg")
{
if (!File.Exists(MapPath("RealImage\\" + Finalimagename)))
{
hFile.PostedFile.SaveAs(ResolveUrl(Server.MapPath("RealImage\\" + Finalimagename)));
System.Drawing.Image imagesize = System.Drawing.Image.FromFile(ResolveUrl(Server.MapPath("RealImage\\" + Finalimagename)));
Bitmap bitmapNew = new Bitmap(imagesize);
if (imagesize.Width < imagesize.Height)
{
myThumbnail150 = bitmapNew.GetThumbnailImage(150 * imagesize.Width / imagesize.Height, 150, myCallback, IntPtr.Zero);
}
else
{
myThumbnail150 = bitmapNew.GetThumbnailImage(150, imagesize.Height * 150 / imagesize.Width, myCallback, IntPtr.Zero);
}
//Create a new directory name ThumbnailImage
Directory.CreateDirectory(Server.MapPath("ThumbnailImage"));
//Save image in TumbnailImage folder
myThumbnail150.Save(ResolveUrl(Server.MapPath("ThumbnailImage\\")) + Finalimagename,
System.Drawing.Imaging.ImageFormat.Jpeg);
MessageLabel.Text = "Successfully uploaded"
}
}
else
{
lblError1.Visible = true;
lblError1.Text = "Check image extension";
}
}
}
}
Output :
First of all upload image.

Figure1.
This is real image.
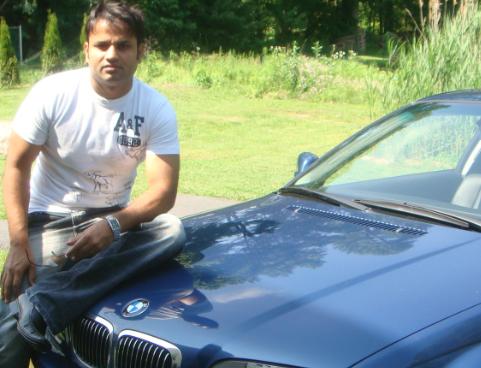
Figure2.
This is thumbnail image.
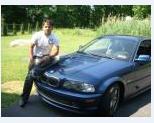
Figure3.
For more information download attached file.