Introduction:
This article describes an approach used to determine the time of the last system boot up and to display the time elapsed since boot up. The application uses element of the System.Management library to run a select query against Win32_OperatingSystem; the application also display the amount of elapsed time since system boot up by comparing the boot up time against the current time; the elapsed time is updated every 1000 milliseconds whilst the application is running. A TimeSpan object is used to calculate the difference between the current time and the boot up time.
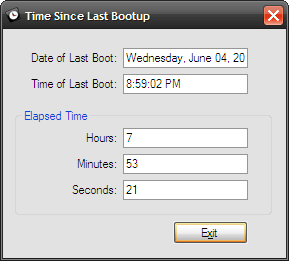
Figure 1: The Application
Getting Started:
In order to get started, unzip the included project and open the solution in the Visual Studio 2008 environment. In the solution explorer, you should note these files (Figure 2):
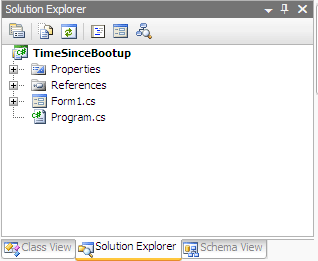
Figure 2: Solution Explorer
As you can see from Figure 2; there is a single Win Forms project containing a single form. All code required of this application is included in this form's code.
The Main Form (Form1.cs).
The main form of the application, Form1, contains all of the code necessary.
If you'd care to open the code view up in the IDE you will see that the code file begins with the following library imports:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Management;
Note that the defaults have been altered and now include the reference to the System.Management library.
Following the imports, the namespace, class, and constructor are defined:
namespace TimeSinceBootup
{
public partial class Form1 : Form
{
Next up, a local variable is defined:
// local member variable
private DateTime dtBootTime = new DateTime();
This variable is used to store the last boot up time; this value is set when the application starts and is used in each subsequent evaluation the elapsed time (in response to a timer tick event).
The next section of code is the form's constructor; the only addition to the default was to enable the timer used to update the display of the elapsed time since the last system boot up.
/// <summary>
/// Enable the timer in the
/// constructor
/// </summary>
public Form1()
{
InitializeComponent();
timer1.Enabled = true;
}
Next up is form load event handler; in this section of code, a SelectQuery from System.Management is created and set to obtain the last boot up time from the operating system. The query is executed and a ManagementDateTimeConverter is used convert the recovered value to a System.DateTime value. That value is then used to set the boot time variable and to then display the start date and start time for the last system boot up.
/// <summary>
/// On form load, use a Management.Select query
/// to discover the last boot time and set
/// the boot time datetime variable to maintain
/// that value
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void Form1_Load(object sender, EventArgs e)
{
// define a select query
SelectQuery query =
new SelectQuery("SELECT LastBootUpTime FROM Win32_OperatingSystem
WHERE Primary='true'");
// create a new management object searcher and pass it
// the select query
ManagementObjectSearcher searcher =
new ManagementObjectSearcher(query);
// get the datetime value and set the local boot
// time variable to contain that value
foreach(ManagementObject mo in searcher.Get())
{
dtBootTime =
ManagementDateTimeConverter.ToDateTime(
mo.Properties["LastBootUpTime"].Value.ToString());
// display the start time and date
txtDate.Text = dtBootTime.ToLongDateString();
txtTime.Text = dtBootTime.ToLongTimeString();
}
}
Next up is the timer tick event handler; the timer is set to an interval of 1000 milliseconds and every time one second passes, the elapsed time is recalculated by setting the difference between the boot up time and the current time into a TimeSpan object. The time span is then used to set the display of the hours, minutes, and seconds that have passed since the last system boot up.
/// <summary>
/// Update the elapsed time display
/// every second
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void timer1_Tick(object sender, EventArgs e)
{
// get the current difference betwee
// the last boot time and now using
// a timespace
TimeSpan ts = DateTime.Now - dtBootTime;
// display the hours, minutes, and seconds
// since the last boot time
txtLapsedHours.Text = (ts.Hours).ToString();
txtLapsedMinutes.Text = (ts.Minutes).ToString();
txtLapsedSeconds.Text = (ts.Seconds).ToString();
}