In my previous article we discussed SOAP Message Versions. Now let us move ahead and create a message.

You can create a Message using the CreateMessage() method of the Message class. This method is overloaded with 11 different sets of input parameters.
The easiest way to create a message is by just providing the MessageVersion and an action:

When the above code snippet is run you will get a message as output and if you notice there is no body in the message since we have not added that.
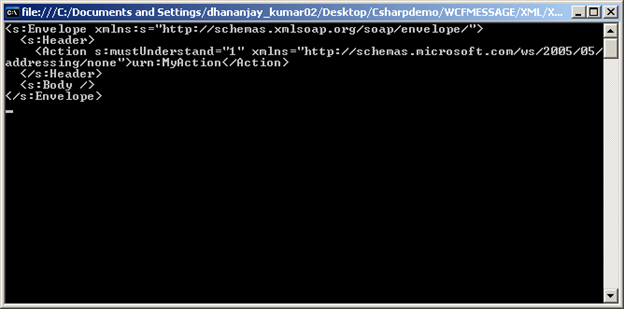
If you want to add a body to the message you can do that but the restriction is that you can only add a serialized object as a message body.
Let us add a string to the message body. Since strings and other built-in types are by default serializable. So we do not need to explicitly serialize it.

In the above function we have added a string message body with the value "HI I am Body". When running the above code snippet you will get output as below:
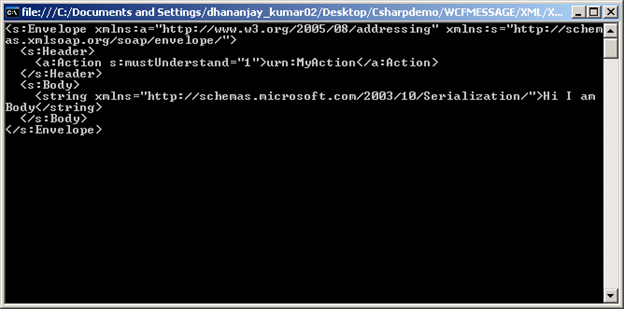
Let us create a message as an object of a custom class as the message body. Assume you have a custom class called Product.
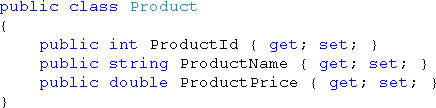
And you want to create a message having an instance of this class as the message body. You can very much do that as below:
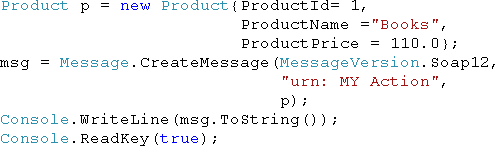
On running the above code you will get output as below:
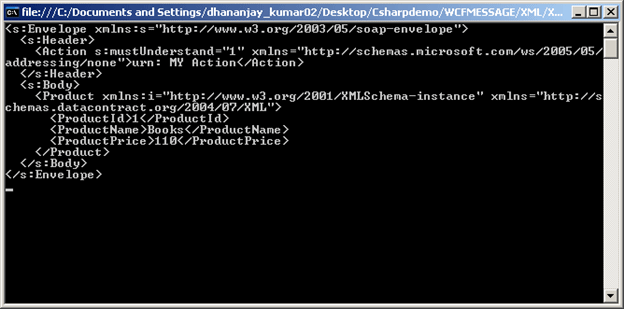
And you can see the custom object is part of the message.
For your reference the code for you to copy is given below:
Program.cs
using System;
using System.ServiceModel.Channels;
using System.ServiceModel;
using System.Xml;
using System.Collections.Generic;
namespace XML
{
class Program
{
static void Main(string[] args)
{
Message msg = Message.CreateMessage(MessageVersion.Soap11,
"urn:MyAction");
Console.WriteLine(msg.ToString());
Console.ReadKey(true);
msg = Message.CreateMessage(MessageVersion.Soap11WSAddressing10,
"urn:MyAction",
"Hi I am Body");
Console.WriteLine(msg.ToString());
Console.ReadKey(true);
Product p = new Product
{
ProductId = 1,
ProductName = "Books",
ProductPrice = 110.0
};
msg = Message.CreateMessage(MessageVersion.Soap12,
"urn: MY Action",
p);
Console.WriteLine(msg.ToString());
Console.ReadKey(true);
}
}
public class Product
{
public int ProductId { get; set; }
public string ProductName { get; set; }
public double ProductPrice { get; set; }
}
}
Now you know how you can create a message in WCF. I hope this article was useful. Thanks for reading.