Objective:
In this tutorial we will fetch data from SQL Server database using LINQ and display that data in Silver Light, while we are following ASP.Net MVC Framework.
Step 1:
Create a Silver Light application.
File
New
Project
Silver Light
Give a meaningful name. Here name is SilverLightinMVCFramework.
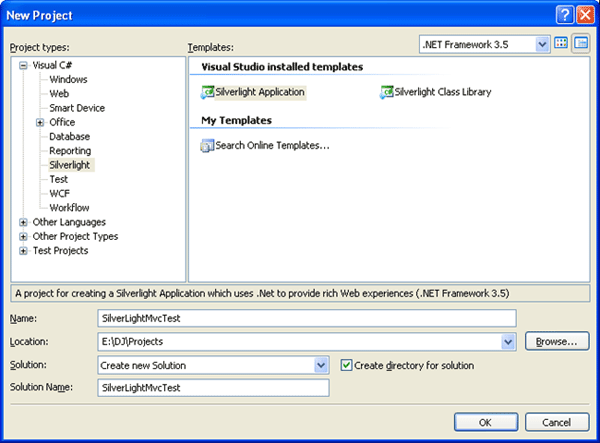
Step 2:
Hosting Silver Light application.
There are three options available for hosting a Silver Light application. Select ASP.Net MVC Web project. Then after ,Select option NO for creating UNIT Test Project.
Now a SilverLight application with ASP.Net MVC host web project.
Solution Explorer should look like below.
Step 3:
Right click on Controller. Click on Add-> New Item and select add LINQ to SQL classes. Give a name, here name is Test.dbml
Step 4:
Here, I have already created a TEST data base in my database. I am going to display data from this database.
-
Open Server Explorer
-
Right click on Data Connection
-
Click Add New Data Connection.
Below screen will get display.
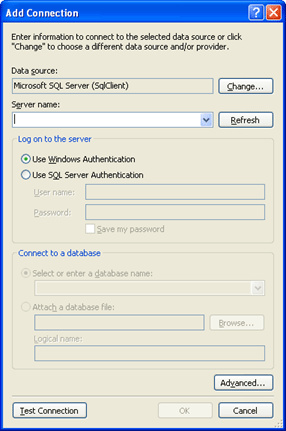
Now give a Server name and select database to connect. In my case name of database is Test.
Expand test.dbo. Here there are 2 tables in Test database. One is Test_Details and other is testsample. In this tutorial, I am going to display data from Test_Detatils table. So select this table from Server explorer and drag it on Test.dbml page.
Now if you click on Test.Dbml.CS file. You will find code has been created for you. Code is as follows. You don't need to write this code. It has been already created once you drag table from server explorer to Test.Dbml page.
Test.Dbml.CS
#pragma warning disable 1591
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated by a tool.
// Runtime Version:2.0.50727.3053
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace SilverLightMvcTest.Web.Models
{
using System.Data.Linq;
using System.Data.Linq.Mapping;
using System.Data;
using System.Collections.Generic;
using System.Reflection;
using System.Linq;
using System.Linq.Expressions;
using System.ComponentModel;
using System;
[System.Data.Linq.Mapping.DatabaseAttribute(Name="test")]
public partial class TestDataContext : System.Data.Linq.DataContext
{
private static System.Data.Linq.Mapping.MappingSource mappingSource = new AttributeMappingSource();
#region Extensibility Method Definitions
partial void OnCreated();
#endregion
public TestDataContext() :
base(global::System.Configuration.ConfigurationManager.ConnectionStrings["testConnectionString"].ConnectionString, mappingSource)
{
OnCreated();
}
public TestDataContext(string connection) :
base(connection, mappingSource)
{
OnCreated();
}
public TestDataContext(System.Data.IDbConnection connection) :
base(connection, mappingSource)
{
OnCreated();
}
public TestDataContext(string connection, System.Data.Linq.Mapping.MappingSource mappingSource) :
base(connection, mappingSource)
{
OnCreated();
}
public TestDataContext(System.Data.IDbConnection connection, System.Data.Linq.Mapping.MappingSource mappingSource) :
base(connection, mappingSource)
{
OnCreated();
}
public System.Data.Linq.Table<Test_Detail> Test_Details
{
get
{
return this.GetTable<Test_Detail>();
}
}
}
[Table(Name="dbo.Test_Details")]
public partial class Test_Detail
{
private string _testId;
private string _testName;
private int _testMaxMarks;
public Test_Detail()
{
}
[Column(Storage="_testId", DbType="NVarChar(50) NOT NULL", CanBeNull=false)]
public string testId
{
get
{
return this._testId;
}
set
{
if ((this._testId != value))
{
this._testId = value;
}
}
}
[Column(Storage="_testName", DbType="NVarChar(50) NOT NULL", CanBeNull=false)]
public string testName
{
get
{
return this._testName;
}
set
{
if ((this._testName != value))
{
this._testName = value;
}
}
}
[Column(Storage="_testMaxMarks", DbType="Int NOT NULL")]
public int testMaxMarks
{
get
{
return this._testMaxMarks;
}
set
{
if ((this._testMaxMarks != value))
{
this._testMaxMarks = value;
}
}
}
}
}
#pragma warning restore 1591
Up to this step, Linq class has been created.
Step 5:
Click on Controller and select HomeController. You will see code window for HomeController.
Right now this is containing two actions called Index and About.
Note : Methods inside a controller is called action. For details on the same , see other articles.
Step 6:
We will add here one more action called List inside Home Controller. Purpose of this action is to fetch out all records of Test_Details table and return List of records as JSON.
Note : For different return types from Action see other articles.
Add following code in Home Controller class. Don't forget to include at the top of HomeController.cs
using SilverLightMvcTest.Web.Models;
public ActionResult List()
{
TestDataContext _obj = new TestDataContext();
var _res = from r in _obj.Test_Details
select r;
return Json(_res);
}
The above action List is returning JSON of all the records fetch from Test_Dtails table of Test Database.
So now the complete code of HomeController.cs will look like ,
HomeController.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using SilverLightMvcTest.Web.Models;
namespace SilverLightMvcTest.Web.Controllers
{
[HandleError]
public class HomeController : Controller
{
public ActionResult Index()
{
ViewData["Message"] = "Welcome to ASP.NET MVC!";
return View();
}
public ActionResult About()
{
return View();
}
public ActionResult List()
{
TestDataContext _obj = new TestDataContext();
var _res = from r in _obj.Test_Details
select r;
return Json(_res);
}
}
}
Step 7:
Now click on
View
Home
Right Click
Add
View
Give name of the View exactly of action name. Here action name is List so name of view will be List. Leave other things as default and click on Add. It will create a List.aspx page.
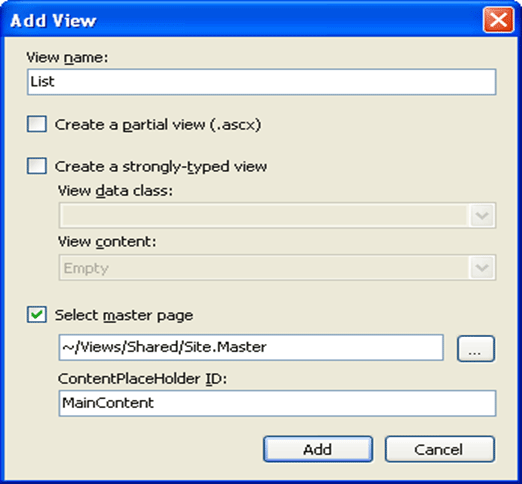
List.aspx will have following code.
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
<title>List</title>
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<h2>List</h2>
</asp:Content>
Remove
<h2>List</h2>
And add below code
<p>
<object data=""data:application/x-silverlight-2" type="application/x-silverlight-2" width="300px" height="300px">
<param name="source" value="/ClientBin/SilverLightMvcTest.xap" />
<param name="minRuntimeVersion" value="2.0.31005.0" />
<param name="windowless" value="true" />
<param name="Background" value="#00FFFFFF" />
</object>
</p>
Here at value property of source, name is SilverLightMvcTest.xap . Here make sure you are giving the name, which name you gave to your SilverLight Project at Step 1:
So now complete code for List.aspx would be
List.aspx
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
<asp:Content ID="Content1" ContentPlaceHolderID="head" runat="server">
<title>List</title>
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<p>
<object data=""data:application/x-silverlight-2" type="application/x-silverlight-2" width="300px" height="300px">
<param name="source" value="/ClientBin/SilverLightMvcTest.xap" />
<param name="minRuntimeVersion" value="2.0.31005.0" />
<param name="windowless" value="true" />
<param name="Background" value="#00FFFFFF" />
</object>
</p>
</asp:Content>
Step 8:
Copy paste the below code in Page.Xaml.CS file.
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button x:Name="b1" Height="100" Width="100" Click="b1_Click" Grid.Row="0" Grid.Column="1"/>
Now click on
View
ToolBox
Drag DataGrid and drop it after Button element of Xaml. After dragging complete code of Page.xaml.Cs file would be like below
Page.Xaml.cs
<UserControl x:Class="SilverLightMvcTest.Page"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:data="clr-namespace:System.Windows.Controls;assembly=System.Windows.Controls.Data"
Width="400" Height="300">
<Grid x:Name="LayoutRoot" Background="White">
<Grid.RowDefinitions>
<RowDefinition Height="Auto" />
<RowDefinition Height="Auto" />
</Grid.RowDefinitions>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="Auto" />
<ColumnDefinition Width="Auto" />
</Grid.ColumnDefinitions>
<Button x:Name="b1" Height="100" Width="100" Click="b1_Click" Grid.Row="0" Grid.Column="1"/>
<data:DataGrid x:Name="MyGrid" Grid.Row="1" Grid.Column="1"> </data:DataGrid>
</Grid>
</UserControl>
I have given name of DataGrid as MyGrid and for button as b1.
Step 9:
Right click on SilverLight Project and add new class . Give it name Test_Details.
Add following code in Test_Details.cs
Test_Details.cs
using System;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Ink;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace SilverLightMvcTest
{
public class Test_Details
{
public string testId
{
get;
set;
}
public string testName
{
set;
get;
}
public int testMaxMarks
{
set;
get;
}
}
}
Make sure here property name and return type is exactly same of the column name and types in table of database.
Step 10:
-
Run your application, by clicking F5.
-
Below screen will come
-
Click on address bar and type
http://localhost:2675/home/list
Copy this URL.
Step 11:
Page.Xaml.CS
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Runtime.Serialization.Json;
namespace SilverLightMvcTest
{
public partial class Page : UserControl
{
public Page()
{
InitializeComponent();
}
private void b1_Click(object sender, RoutedEventArgs e)
{
WebClient _client = new WebClient();
_client.OpenReadCompleted += new OpenReadCompletedEventHandler(_client_OpenReadCompleted);
_client.OpenReadAsync(new Uri("http://localhost:2675/home/list"));
}
void _client_OpenReadCompleted(object sender, OpenReadCompletedEventArgs e)
{
DataContractJsonSerializer json = new DataContractJsonSerializer(typeof(List<Test_Details>));
IList<Test_Details> res = (List<Test_Details>)(json.ReadObject(e.Result));
MyGrid.ItemsSource = res;
}
}
}
Point to note in above code is
In line
_client.OpenReadAsync(new Uri("http://localhost:2675/home/list"));
As the parameter of URI, paste that URI which we copied at step 10 C.
Now Run this by pressing F5, you will get the output, when you click on Button.
Output after clicking on Button
How to use attached Zip File?
Step 1: Download
Step 2: Unzip
Step 3: create a table in database called Test_Details with structure explained above
Step 4: see on which port number, Silverlight application is running.
Let , if it is running on port number 2689.
Change the code in Page.Xaml.Cs file
With
_client.OpenReadAsync(new Uri("http://localhost:2689/home/list"));
It will run..
Happy Coding!