In this article I will show you how you can create a Photo Viewer from images of the Flicker Service.
Flicker Service
Flicker exposes a REST based feed to access images. You can find information about Flicker public feeds at the following link.
http://www.flickr.com/services/feeds/docs/photos_public/
Public images feed can be found at:
http://api.flickr.com/services/feeds/photos_public.gne
Expected Output
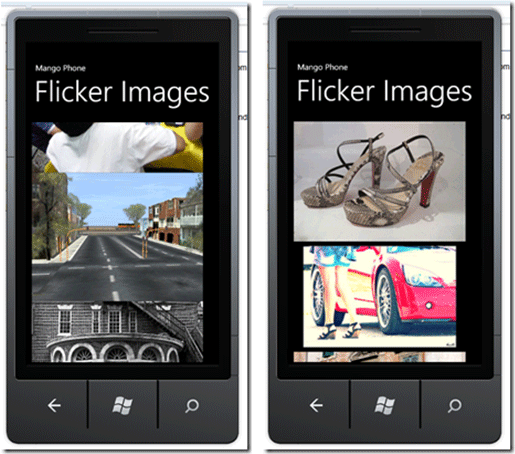
Approach
- Call Flicker Service
- Parse XML feed
- Bind it to List Box.
Create Windows Phone 7.1 Application
Create a Windows 7 Phone Application and select Windows Phone 7.1 as the target Windows Phone version.
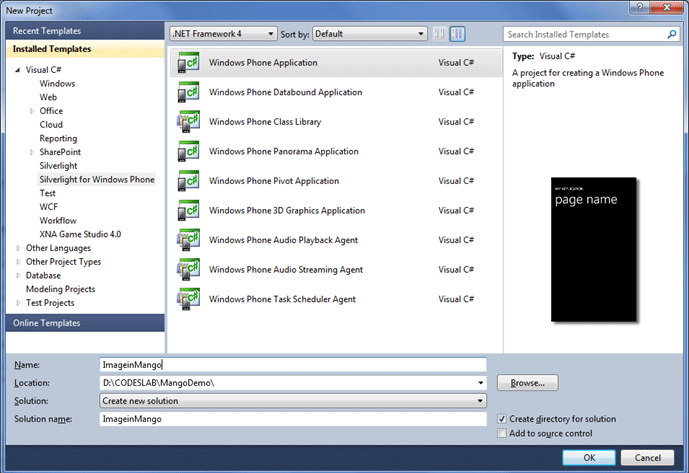
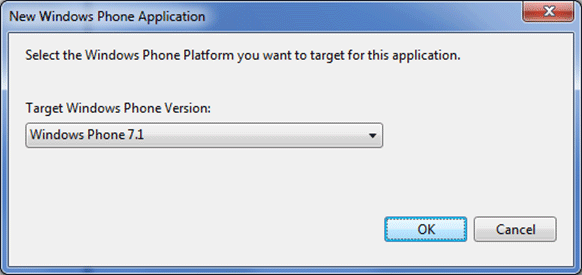
Create Photo class
To bind images to the ListBox, let us create a Photo class. Right-click and add a class called Photo to the project. When we parse Flicker Image feeds, we will bind a public Image URL here.
Photo.cs
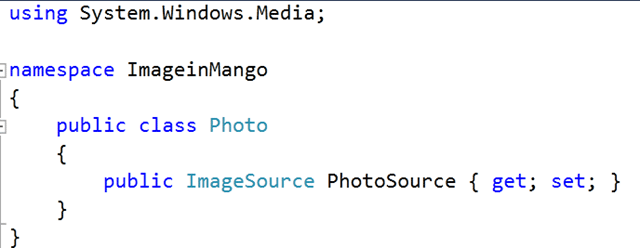
Parsing Image Feed of Flicker Service
When you open a public feed URL of Flicker in browser, you can view the feeds in the browser itself.

We are going to give value of link element from the feed in Image as source value.
We will have to make asynchronous calls as below:

Then in DonwnloadStringCompleted event, we will have to perform the following tasks:
- Create an observable collection of photos
- Parse the feed
- Iterate through all the public image url in feed and add to collection.
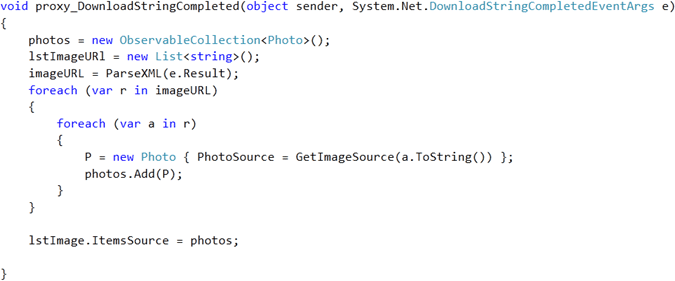
Parsing of feeds can be done as below:
- We are parsing Link URL.
- Fetching the value of href attribute in Link URL.
- There are two link elements and we are fetching the value of a link element having rel attribute value as enclosure
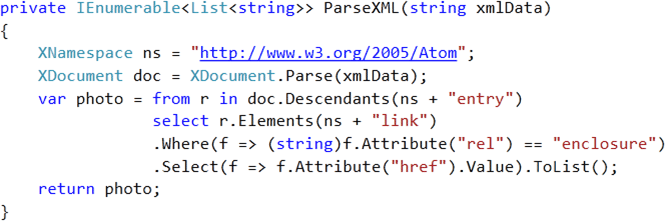
Design the Page
We are going to put a ListBox in content grid and inside ItemTemplate of ListBox , we will put Image control.
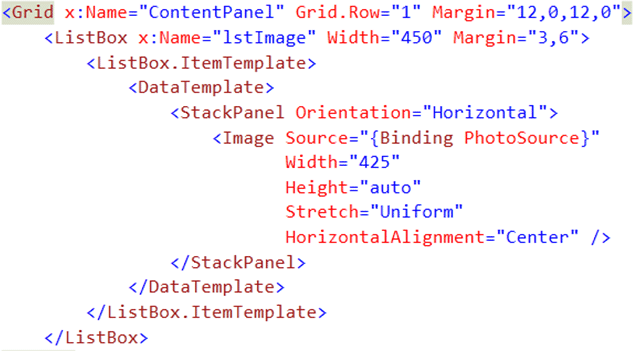
As the source of the Image you need to bind PhotoSource property of Photo class.
For Reference the full source codes are given below. Feel free to use for your purpose.
MainPage.xaml
<phone:PhoneApplicationPage
x:Class="FlickerImageFeed.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:phone="clr-namespace:Microsoft.Phone.Controls;assembly=Microsoft.Phone"
xmlns:shell="clr-namespace:Microsoft.Phone.Shell;assembly=Microsoft.Phone"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d" d:DesignWidth="480" d:DesignHeight="768"
FontFamily="{StaticResource PhoneFontFamilyNormal}"
FontSize="{StaticResource PhoneFontSizeNormal}"
Foreground="{StaticResource PhoneForegroundBrush}"
SupportedOrientations="Portrait" Orientation="Portrait"
shell:SystemTray.IsVisible="True">
<!--LayoutRoot is the root grid where all page content is placed-->
<Grid x:Name="LayoutRoot" Background="Transparent">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!--TitlePanel contains the name of the application and page title-->
<StackPanel x:Name="TitlePanel" Grid.Row="0" Margin="12,17,0,28">
<TextBlock x:Name="ApplicationTitle" Text="Mango Phone" Style="{StaticResource PhoneTextNormalStyle}"/>
<TextBlock x:Name="PageTitle" Text="Flicker Images" Margin="9,-7,0,0" Style="{StaticResource PhoneTextTitle1Style}"/>
</StackPanel>
<!--ContentPanel - place additional content here-->
<Grid x:Name="ContentPanel" Grid.Row="1" Margin="12,0,12,0">
<ListBox x:Name="lstImage" Width="450" Margin="3,6">
<ListBox.ItemTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<Image Source="{Binding PhotoSource}"
Width="425"
Height="auto"
Stretch="Uniform"
HorizontalAlignment="Center" />
</StackPanel>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
</Grid>
</phone:PhoneApplicationPage>>
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows.Media;
using Microsoft.Phone.Controls;
using System.Collections.ObjectModel;
using System.Windows.Media.Imaging;
using System.Xml.Linq;
namespace FlickerImageFeed
{
public partial class MainPage : PhoneApplicationPage
{
IEnumerable<List<string>> imageURL;
List<string> lstImageURl;
Photo P;
ObservableCollection<Photo> photos;
public MainPage()
{
InitializeComponent();
GetAllPhotos();
}
private ImageSource GetImageSource(string fileName)
{
return new BitmapImage(new Uri(fileName, UriKind.Absolute));
}
private void GetAllPhotos()
{
WebClient proxy = new WebClient();
proxy.DownloadStringAsync(new Uri("http://api.flickr.com/services/feeds/photos_public.gne"));
proxy.DownloadStringCompleted += new System.Net.DownloadStringCompletedEventHandler
(proxy_DownloadStringCompleted);
}
void proxy_DownloadStringCompleted(object sender, System.Net.DownloadStringCompletedEventArgs e)
{
photos = new ObservableCollection<Photo>();
lstImageURl = new List<string>();
imageURL = ParseXML(e.Result);
foreach (var r in imageURL)
{
foreach (var a in r)
{
P = new Photo { PhotoSource = GetImageSource(a.ToString()) };
photos.Add(P);
}
}
lstImage.ItemsSource = photos;
}
private IEnumerable<List<string>> ParseXML(string xmlData)
{
XNamespace ns = "http://www.w3.org/2005/Atom";
XDocument doc = XDocument.Parse(xmlData);
var photo = from r in doc.Descendants(ns + "entry")
select r.Elements(ns + "link")
.Where(f => (string)f.Attribute("rel") == "enclosure")
.Select(f => f.Attribute("href").Value).ToList();
return photo;
}
}
}
Run the Application
Press F5 to run Photo viewer.
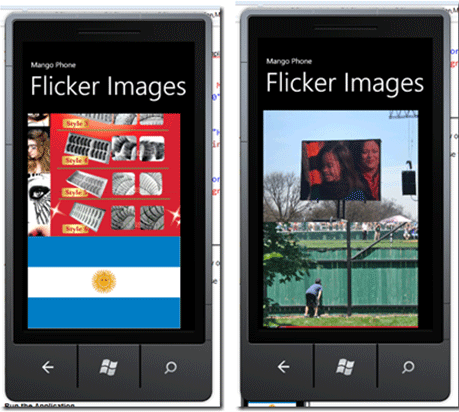
I hope this post was useful. Thanks for reading. In future articles, I will show you how to fetch Images from a server instead of attaching them as resources.