{
AddGradientMode();
textBox1.BackColor = startColor;
textBox2.BackColor = endColor;
}
private void AddGradientMode()
{
//Adds linear gradient mode styles to the combo box
comboBox1.Items.Add(LinearGradientMode.BackwardDiagonal);
comboBox1.Items.Add(LinearGradientMode.ForwardDiagonal);
comboBox1.Items.Add(LinearGradientMode.Horizontal);
comboBox1.Items.Add(LinearGradientMode.Vertical);
comboBox1.Text = LinearGradientMode.BackwardDiagonal.ToString();
}
Next we add code for the Pick... buttons, which allow the user to provide color selections for the starting and ending colors. We also set the color of relative text boxes, as shown in Listing 4.12.
LISTING 4.12: The pick button click event handler
private void StartClrBtn_Click(object sender, System.EventArgs e)
{
//Use ColorDialog to select a color
ColorDialog clrDlg = new ColorDialog();
if (clrDlg.ShowDialog() == DialogResult.OK)
{
//Save color as foregorumd color,
//and fill text box with this color
startColor = clrDlg.Color;
textBox1.BackColor = startColor;
}
}
private void EndClrBtn_Click(object sender, System.EventArgs e)
{
//Use ColorDialog to select a color
ColorDialog clrDlg = new ColorDialog();
if (clrDlg.ShowDialog() == DialogResult.OK)
{
//Save color as background color,
//and fill text box with this color
endColor = clrDlg.Color;
textBox2.BackColor = endColor;
}
}
The last step is to write code for the Apply Setting button. This button reads various settings, including the selected gradient mode in the combo box, the starting and ending colors, another rectangle, and gamma correction. As Listing 4.13 shows, the code creates a linear gradient brush using a rectangle, two colors and the gradient mode selection. After creating the brush, it calls the FillRectangle method.
LISTING 4.13: The Apply Settings button click event handler
private void ApplyBtn_Click(object sender, System.EventArgs e)
{
Graphics g = this.CreateGraphics();
g.Clear(this.BackColor);
//Read current style from combo box
string str = comboBox1.Text;
//find out the mode and set is as the current mode
switch (str)
{
case "BackwardDiagonal":
mode = LinearGradientMode.BackwardDiagonal;
break;
case "ForwardDiagonal":
mode = LinearGradientMode.ForwardDiagonal;
break;
case "Horizontal":
mode = LinearGradientMode.Horizontal;
break;
case "Vertical":
mode = LinearGradientMode.Vertical;
break;
default:
break;
}
//Create rectangle
Rectangle rect = new Rectangle(70, 200, 200, 220);
//Create linear gradient brush and mode
if (checkBox1.Checked)
{
Rectangle rect1 = new Rectangle(20, 20, 50, 50);
lgBrush = new LinearGradientBrush
(rect1, startColor, endColor, mode);
}
else
{
lgBrush = new LinearGradientBrush
(rect, startColor, endColor, mode);
}
//Gamma correction check box is checked
if (checkBox1.Checked)
{
lgBrush.GammaCorrection = true;
}
//Fill Rectangle
g.FillRectangle(lgBrush, rect);
//Dispose of objects
if (lgBrush != null)
lgBrush.Dispose();
g.Dispose();
}
When you run the application, the result looks like Figure 4.15.
FIGURE 4.15: The default linear gradient brush output
To generate a different output, let's change the linear gradient mode to Vertical. We'll also change the colors, with the results shown in Figure 4.16.
Let's' change the colors and gradient mode again, this time selecting the Other Rectangle check box. The sets a range of the gradient. If the output is out of range, the gradient repeats itself. The new output looks like Figure 4.17.
Conclusion
Hope the article would have helped you in understanding Gradient Brushes in GDI+. Read other articles on GDI+ on the website.
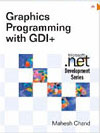 |
This book teaches .NET developers how to work with GDI+ as they develop applications that include graphics, or that interact with monitors or printers. It begins by explaining the difference between GDI and GDI+, and covering the basic concepts of graphics programming in Windows. |