Brief Description
Programming C# is a new self-taught series of articles, in which I will teach you C# language in a tutorial format. This article concentrates on arrays in .NET and how you can work with arrays using C# language. Article also covers the Arrays class and its method, which can be used to sort, search, get, and set an array items.
Introduction
In C#, an array index starts at zero. That means first item of an array will be stored at 0th position. The position of the last item on an array will total number of items - 1.
In C#, arrays can be declared as fixed length or dynamic. Fixed length array can stores a predefined number of items, while size of dynamic arrays increases as you add new items to the array. You can declare an array of fixed length or dynamic. You can even change a dynamic array to static after it is defined. For example, the following like declares a dynamic array of integers.
int
[] intArray;
The following code declares an array, which can store 5 items starting from index 0 to 4.
int
[] intArray;
intArray = new int[5];
The following code declares an array that can store 100 items starting from index 0 to 99.
int
[] intArray;
intArray = new int[100];
Single Dimension Arrays
Arrays can be divided into four categories. These categories are single-dimensional arrays, multidimensional arrays or rectangular arrays, jagged arrays, and mixed arrays.
Single-dimensional arrays are the simplest form of arrays. These types of arrays are used to store number of items of a predefined type. All items in a single dimension array are stored in a row starting from 0 to the size of array - 1.
In C# arrays are objects. That means declaring an array doesn't create an array. After declaring an array, you need to instantiate an array by using the "new" operator.
The following code declares a integer array, which can store 3 items. As you can see from the code, first I declare the array using [] bracket and after that I instantiate the array by calling new operator.
int
[] intArray;
intArray = new int[3];
Array declarations in C# are pretty simple. You put array items in curly braces ({}). If an array is not initialized, its items are automatically initialized to the default initial value for the array type if the array is not initialized at the time it is declared.
The following code declares and initializes an array of three items of integer type.
int
[] intArray;
intArray = new int[3] {0, 1, 2};
The following code declares and initializes an array of 5 string items.
string
[] strArray = new string[5] {"Ronnie", "Jack", "Lori", "Max", "Tricky"};
You can even direct assign these values without using the new operator.
string
[] strArray = {"Ronnie", "Jack", "Lori", "Max", "Tricky"};
Multi Dimension Arrays
A multidimensional array is an array with more than one dimension. A multi dimension array is declared as following:
string
[,] strArray;
After declaring an array, you can specify the size of array dimensions if you want a fixed size array or dynamic arrays. For example, the following code two examples create two multi dimension arrays with a matrix of 3x2 and 2x2. The first array can store 6 items and second array can store 4 items respectively.
int
[,] numbers = new int[3, 2] { {1, 2}, {3, 4}, {5, 6} };
string[,] names = new string[2, 2] { {"Rosy","Amy"}, {"Peter","Albert"} };
If you don't want to specify the size of arrays, just don't define a number when you call new operator. For example,
int
[,] numbers = new int[,] { {1, 2}, {3, 4}, {5, 6} };
string[,] names = new string[,] { {"Rosy","Amy"}, {"Peter","Albert"} };
You can also omit the new operator as we did in single dimension arrays. You can assign these values directly without using the new operator. For example:
int
[,] numbers = { {1, 2}, {3, 4}, {5, 6} };
string[,] siblings = { {"Rosy", "Amy"}, {"Peter", "Albert"} };
Jagged Arrays
Jagged arrays are often called array of arrays. An element of a jagged array itself is an array. For example, you can define an array of names of students of a class where a name itself can be an array of three strings - first name, middle name and last name. Another example of jagged arrays is an array of integers containing another array of integers. For example,
int
[][] numArray = new int[][] { new int[] {1,3,5}, new int[] {2,4,6,8,10} };
Again, you can specify the size when you call the new operator.
Mixed Arrays
Mixed arrays are a combination of multi-dimension arrays and jagged arrays. Multi-dimension arrays are also called as rectangular arrays.
Accessing Arrays using foreach Loop
The foreach control statement (loop) of C# is a new to C++ or other developers. This control statement is used to iterate through the elements of a collection such as an array. For example, the following code uses foreach loop to read all items of numArray.
int
[] numArray = {1, 3, 5, 7, 9, 11, 13};
foreach (int num in numArray)
{
System.Console.WriteLine(num.ToString());
}
A Simple Example
This sample code listed in Listing 1 shows you how to use arrays. You can access an array items by using for loop but using foreach loop is easy to use and better.
Listing 1. Using arrays in C#.
using
System;
namespace ArraysSamp
{
class Class1
{
static void Main(string[] args)
{
int[] intArray = new int[3];
intArray[0] = 3;
intArray[1] = 6;
intArray[2] = 9;
Console.WriteLine("================");
foreach (int i in intArray)
{
Console.WriteLine(i.ToString() );
}
string[] strArray = new string[5]
{"Ronnie", "Jack", "Lori", "Max", "Tricky"};
Console.WriteLine("================");
foreach( string str in strArray)
{
Console.WriteLine(str);
}
Console.WriteLine("================");
string[,] names = new string[,]
{
{"Rosy","Amy"},
{"Peter","Albert"}
};
foreach( string str in names)
{
Console.WriteLine(str);
}
Console.ReadLine();
}
}
}
The output of Listing 1 looks like Figure 1.
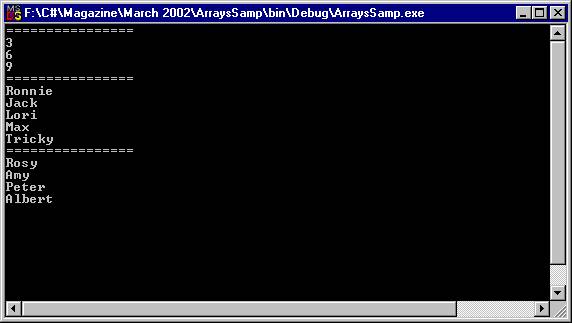
Figure 1.
Understanding the Array Class
The Array class, defined in the System namespace, is the base class for arrays in C#.Array class is an abstract base class but it provides CreateInstance method to construct an array.The Array class provides methods for creating, manipulating, searching, and sorting arrays.
Table 1 describes Array class properties.
Table 1. The System.Array Class Properties.
IsFixedSize |
Return a value indicating if an array has a fixed size or not. |
IsReadOnly |
Returns a value indicating if an array is read-only or not. |
IsSynchronized |
Returns a value indicating if access to an array is thread-safe or not. |
Length |
Returns the total number of items in all the dimensions of an array. |
Rank |
Returns the number of dimensions of an array. |
SyncRoot |
Returns an object that can be used to synchronize access to the array. |
Table 2 describes some of the Array class methods.
Table 2. The System.Array Class Methods.
BinarySearch |
This method searches a one-dimensional sorted Array for a value, using a binary search algorithm. |
Clear |
This method removes all items of an array and sets a range of items in the array to 0. |
Clone |
This method creates a shallow copy of the Array. |
Copy |
This method copies a section of one Array to another Array and performs type casting and boxing as required. |
CopyTo |
This method copies all the elements of the current one-dimensional Array to the specified one-dimensional Array starting at the specified destination Array index. |
CreateInstance |
This method initializes a new instance of the Array class. |
GetEnumerator |
This method returns an IEnumerator for the Array. |
GetLength |
This method returns the number of items in an Array. |
GetLowerBound |
This method returns the lower bound of an Array. |
GetUpperBound |
This method returns the upper bound of an Array. |
GetValue |
This method returns the value of the specified item in an Array. |
IndexOf |
This method returns the index of the first occurrence of a value in a one-dimensional Array or in a portion of the Array. |
Initialize |
This method initializes every item of the value-type Array by calling the default constructor of the value type. |
LastIndexOf |
This method returns the index of the last occurrence of a value in a one-dimensional Array or in a portion of the Array. |
Reverse |
This method reverses the order of the items in a one-dimensional Array or in a portion of the Array. |
SetValue |
This method sets the specified items in the current Array to the specified value. |
Sort |
This method sorts the items in one-dimensional Array objects. |
The Array Class
Array class is an abstract base class but it provides CreateInstance method to construct an array.
Array names = Array.CreateInstance( typeof(String), 2, 4 );
After creating an array using the CreateInstance method, you can use SetValue method to add items to an array. I will discuss SetValue method later in this article.
The Array class provides methods for creating, manipulating, searching, and sorting arrays. Array class provides three boolean properties IsFixedSize, IsReadOnly, and IsSynchronized to see if an array has fixed size, read only or synchronized or not respectively. The Length property of Array class returns the number of items in an array and the Rank property returns number of dimensions in a multi-dimension array.
Listing 1 creates two arrays with a fixed and variable lengths and sends the output to the system console.
Listing 1.
int
[] intArray;
// fixed array with 3 items
intArray = new int[3] {0, 1, 2};
// 2x2 varialbe length array
string[,] names = new string[,] { {"Rosy","Amy"}, {"Peter","Albert"} };
if(intArray.IsFixedSize)
{
Console.WriteLine("Array is fixed size");
Console.WriteLine("Size :" + intArray.Length.ToString());
}
if(names.IsFixedSize)
{
Console.WriteLine("Array is varialbe.");
Console.WriteLine("Size :" + names.Length.ToString());
Console.WriteLine("Rank :" + names.Rank.ToString());
}
Besides these properties, the Array class provides methods to add, insert, delete, copy, binary search, reverse, reverse and so on.
Searching an Item in an Array
The BinarySearch static method of Array class can be used to search for an item in a array. This method uses binary search algorithm to search for an item.The method takes at least two parameters-an array and an object (the item you are looking for). If an item found in an array, the method returns the index of the item (based on first item as 0th item), else method returns a negative value. Listing 2 uses BinarySearch method to search two arrays.
Listing 2. Searching an item in a array.
int
[] intArray = new int[3] {0, 1, 2};
string[] names = new string[] {"Rosy","Amy", "Peter","Albert"};
object obj1 = "Peter";
object obj2 = 1;
int retVal = Array.BinarySearch(names, obj1);
if(retVal >=0)
Console.WriteLine("Item index " +retVal.ToString() );
else
Console.WriteLine("Item not found");
retVal = Array.BinarySearch(intArray, obj2);
if(retVal >=0)
Console.WriteLine("Item index " +retVal.ToString() );
else
Console.WriteLine("Item not found");
Sorting Item in an Array
The Sort static method of the Array class can be used to sort an array items.This method has many overloaded forms. The simplest form takes a parameter of the array, you want to sort to. Listing 3 uses Sort method to sort an array items.
Listing 3. Sorting an array items.
string
[] names = new string[] {"Rosy","Amy", "Peter","Albert"};
Console.WriteLine("Original Array:");
foreach (string str in names)
{
Console.WriteLine(str);
}
Console.WriteLine("Sorted Array:");
Array.Sort(names);
foreach (string str in names)
{
Console.WriteLine(str);
}
Getting and Setting Values
The GetValue and SetValue methods of the Array class can be used to return a value from an index of an array and set values of an array item at a specified index respectively. The code listed in Listing 4 creates a 2-dimension array instance using the CreateInstance method. After that I use SetValue method to add values to the array.
In the end I find number of items in both dimensions and use GetValue method to read values and display on the console.
Listing 4. Using GetValue and SetValue methods.
Array names = Array.CreateInstance( typeof(String), 2, 4 );
names.SetValue( "Rosy", 0, 0 );
names.SetValue( "Amy", 0, 1 );
names.SetValue( "Peter", 0, 2 );
names.SetValue( "Albert", 0, 3 );
names.SetValue( "Mel", 1, 0 );
names.SetValue( "Mongee", 1, 1 );
names.SetValue( "Luma", 1, 2 );
names.SetValue( "Lara", 1, 3 );
int items1 = names.GetLength(0);
int items2 = names.GetLength(1);
for ( int i =0; i < items1; i++ )
for ( int j = 0; j < items2; j++ )
Console.WriteLine(i.ToString() +","+ j.ToString() +": " +names.GetValue( i, j ) );
Other Methods of Array Class
The Reverse static method of the Array class reverses the order of items in a array. Similar to the sort method, you can just pass an array as a parameter of the Reverse method.
Array.Reverse(names);
The Clear static method of the Array class removes all items of an array and sets its length to zero. This method takes three parameters - first an array object, second starting index of the array and third is number of elements. The following code removes two elements from the array starting at index 1 (means second element of the array).
Array.Clear(names, 1, 2);
The GetLength method returns the number of items in an array. The GetLowerBound and GetUppperBound methods return the lower and upper bounds of an array respectively. All these three methods take at least a parameter, which is the index of the dimension of an array. The following code snippet uses all three methods.
string
[] names = new string[] {"Rosy","Amy", "Peter","Albert"};
Console.WriteLine(names.GetLength(0).ToString());
Console.WriteLine(names.GetLowerBound(0).ToString());
Console.WriteLine(names.GetUpperBound(0).ToString());
The Copy static method of the Array class copies a section of an array to another array. The CopyTo method copies all the elements of an array to another one-dimension array. The code listed in Listing 5 copies contents of an integer array to an array of object types.
Listing 5. Copying an array.
// Creates and initializes a new Array of type Int32.
Array oddArray = Array.CreateInstance( Type.GetType("System.Int32"), 5 );
oddArray.SetValue(1, 0);
oddArray.SetValue(3, 1);
oddArray.SetValue(5, 2);
oddArray.SetValue(7, 3);
oddArray.SetValue(9, 4);
// Creates and initializes a new Array of type Object.
Array objArray = Array.CreateInstance( Type.GetType("System.Object"), 5 );
Array.Copy(oddArray, oddArray.GetLowerBound(0), objArray,
bjArray.GetLowerBound(0), 4 );
int items1 = objArray.GetUpperBound(0);
for ( int i =0; i < items1; i++ )
Console.WriteLine(objArray.GetValue(i).ToString());
You can even copy a part of an array to another array by passing number of items and starting item in the Copy method. The following format copies a range of items from an Array starting at the specified source index and pastes them to another Array starting at the specified destination index.
public
static void Copy(Array, int, Array, int, int);
Summary
In this article, you learned basics of arrays and how arrays are handled and used in C#. In the beginning of this article, we discussed different types of arrays such as single dimension, multi dimension, and jagged arrays. After that we discussed the Array class. In the end of this article, we saw how to work with arrays using different methods and properties of Array class.