How To Use Conditional Statement In Oracle
Description
There are three types of conditional statement, which are-
- IF-THEN-ELSE statement
- IF-THEN-ELSIF statement
- CASE
IF-THEN-ELSE Statement
If then else statement is used, when a condition is true, the first statement is executed and second statement is skipped. When the condition is false the first statement is skipped and second statement is executed.
Syntax
- If condition then
- statement 1;
- statement 2;
- else
- statement 3;
- statement 4;
- End if;
Example
- SET SERVEROUTPUT ON;
- DECLARE
- V_NUM NUMBER := &ENTER_A_NUMBER;
- BEGIN
- IF MOD(V_NUM, 2)=0
- THEN
- DBMS_OUTPUT.PUT_LINE(V_NUM||'This is even Number');
- ELSE
- DBMS_OUTPUT.PUT_LINE(V_NUM||'This is odd number');
- END IF;
- END;
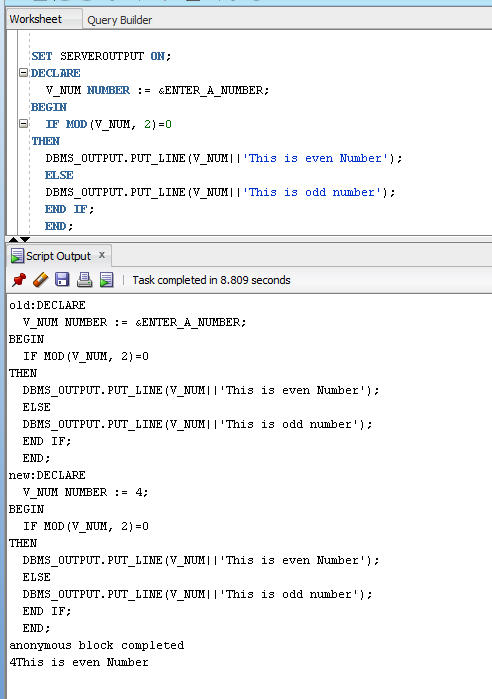
For odd number
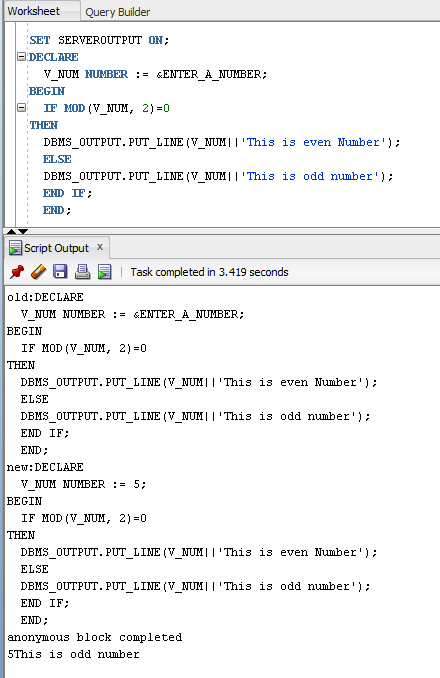
IF-THEN-ELSIF Statement
If a condition for statement is true, it will run, else it goes to next, else if ….if all fail, it executes else statement.
Syntax
- IF condition 1 THEN
- statement 1;
- statement 2;
- ELSIF condition 2 THEN
- statement 3;
- statement 4;
- ELSIF condition 3 then
- statement 5;
- statement 6;
- ………
- ELSE
- statement N;
- END IF;
Example
- SET SERVEROUTPUT ON;
- DECLARE
- V_color varchar2(20) := '&ENTER_A_color_name';
- BEGIN
- IF v_color ='red'
- THEN
- DBMS_OUTPUT.PUT_LINE('the sun is red');
- ELSIF
- v_color='blue'
- THEN
- DBMS_OUTPUT.PUT_LINE('the ocean is blue');
- ELSIF
- v_color='green'
- THEN
- DBMS_OUTPUT.PUT_LINE('the leaf is green');
- ELSE
- DBMS_OUTPUT.PUT_LINE('this is natural color');
- END IF;
- DBMS_OUTPUT.PUT_LINE('the world is very beautiful');
- END;
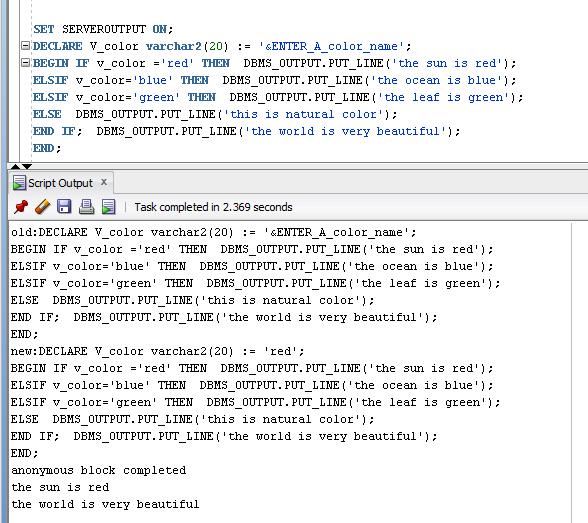
Enter a Blue color
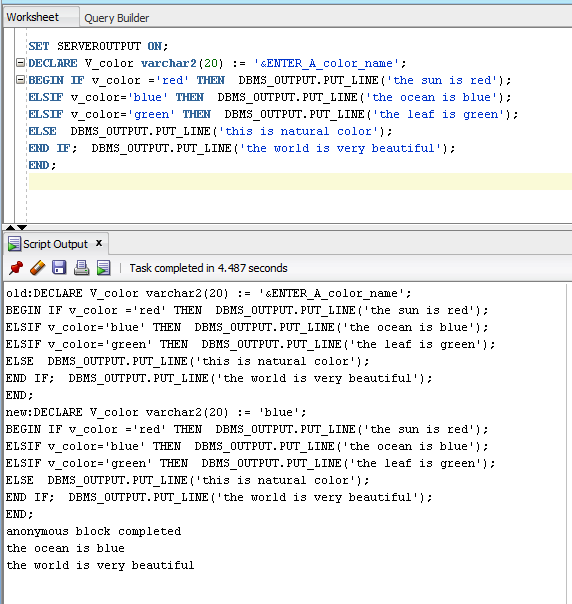
CASE Statement
There are two types of case expression, which are-
- Simple case expression
- Searched case expression
Syntax
- CASE [Expression]
- When condition 1 THEN Result 1
- When condition 2 THEN Result 2
- ……..
- When condition n THEN Result n
- ELSE Result
- END
Example
Simple case expression
- SELECT EMP_ID, EMP_NAME,
- (
- CASE EMP_ID
- WHEN 7001 THEN 'PRESIDENT'
- WHEN 7002 THEN 'CLERK'
- WHEN 7003 THEN 'PRESIDENT'
- WHEN 7004 THEN 'SALESMAN'
- WHEN 7005 THEN 'SALESMAN'
- WHEN 7006 THEN 'CLERK'
- WHEN 7007 THEN 'CLERK'
- ELSE 'SORRY'
- END
- )
- FROM EMPLOYEE;
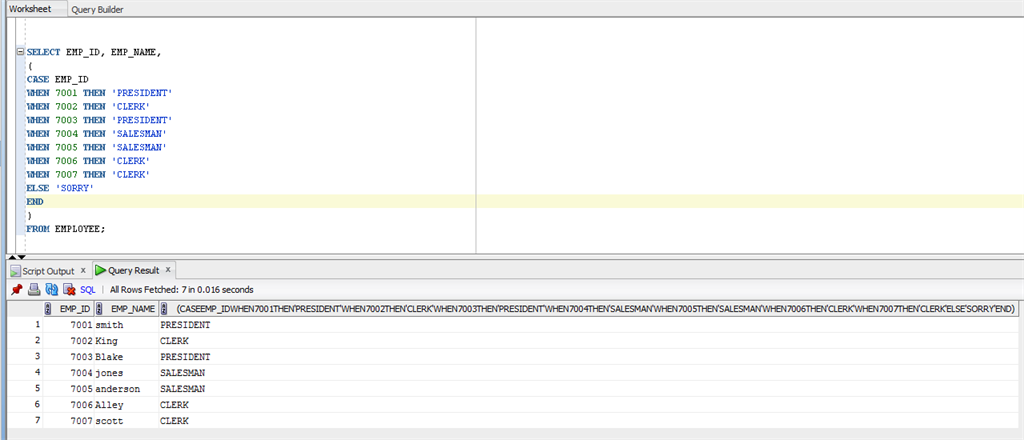
Searched case expression
- SELECT
- (
- CASE 'SMITH'
- WHEN 'KING' THEN 'FALSE'
- WHEN 'SMITH' THEN '2 TRUE'
- WHEN 'ALLEY' THEN '3 FALSE'
- WHEN 'ANDERSON'THEN '4 TRUE'
- ELSE 'SORRY'
- END
- )
- FROM DUAL;
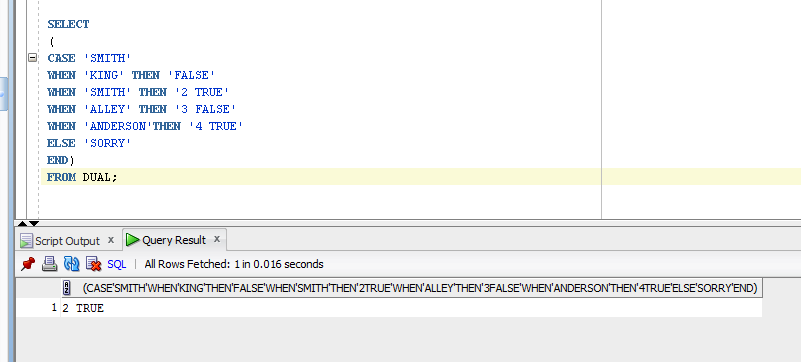
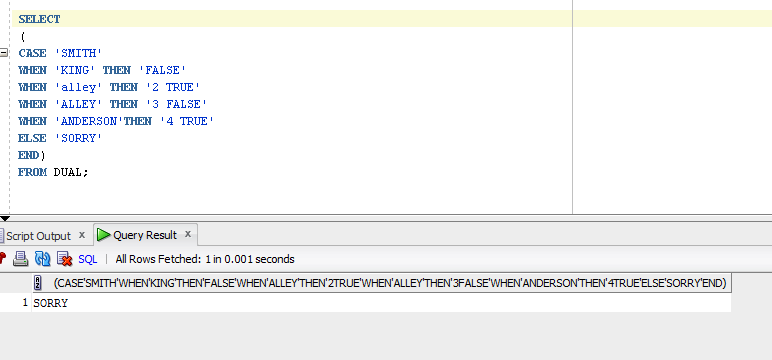
Summary