How To Use The Loop Statement In Oracle
Description
There are some types of loop statement in Oracle, which are-
- Simple - loop
- While - loop
- For – loop
- Cursor – For – loop
A simple loop statement executes a sequence of statements multiple times. The loop and End loop keywords enclose the statement.
Syntax
- Loop
- statement 1;
- statement 2;
- statement 3;
- ……
- End loop;
- set serveroutput on;
- Declare
- v_counter number : = 0;
- v_result number;
- Begin
- loop
- v_counter : = v_counter + 1;
- v_result : = 15 * v_counter;
- DBMS_output.put_line(‘15’ || ‘*’ ||v_counter||’=’||v_result);
- if v_counter >=10 then
- Exit;
- End if;
- End loop;
- End;
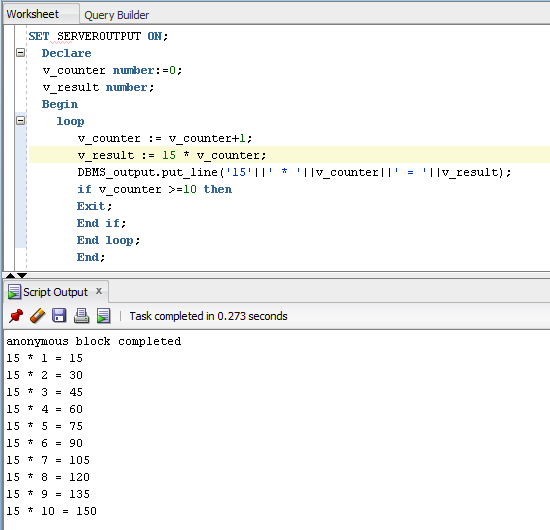
WHILE LOOP
It executes block of the statements several times. It is best used, when number of iteration to be performed are unknown.
Syntax
- While condition Loop
- statement 1;
- statement 2;
- statement 3;
- ……
- End loop;
In the example, given below, print multiplication table of 15, using while loop.
- Set ServerOutPut ON;
- Declare
- v_counter number :=1;
- v_result number;
- Begin
- While
- v_counter <=10 loop
- v_result :=15 * v_counter;
- DBMS_output.put_line(‘15’||’ * ’||v_counter||’ = ’||v_result);
- v_counter := v_counter+1
- End loop;
- DBMS_output.put_line(‘outside the loop’);
- End;
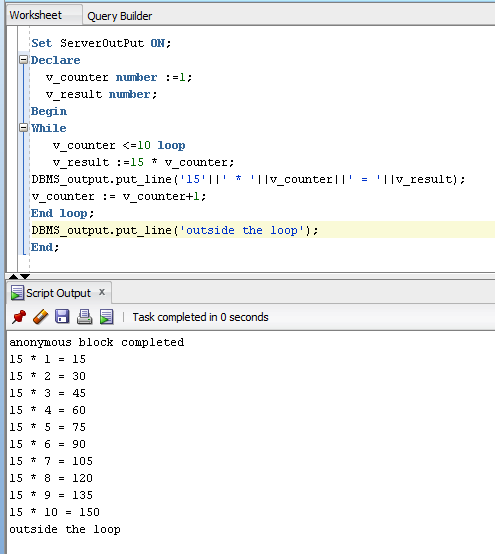
While loop works with Boolean variable as a Test condition.
Example
- Set serveroutput ON;
- Declare
- v_Test nBoolean := TRUE;
- v_counter number := 0;
- Begin
- while v_Test loop
- v_counter :=v_counter +1;
- DBMS_output.put_line(v_counter);
- //Loop termination code//
- if v_counter = 10
- then
- v_Test := false
- End if;
- End loop;
- DBMS_output.put_line(‘outside the loop’);
- End;
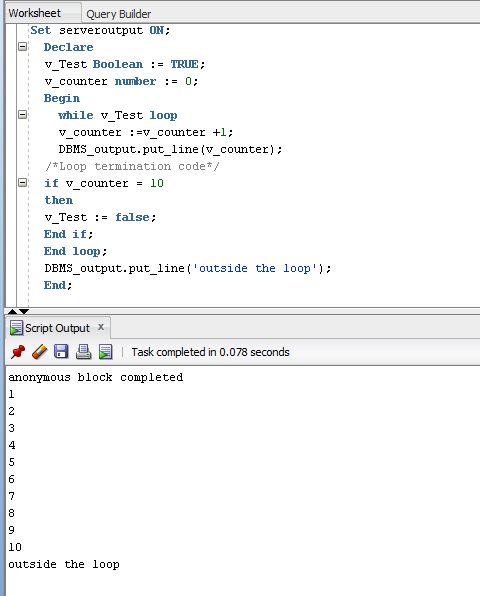
FOR LOOP
For loop allows you to execute the block of statements repeatedly for fixed number of times.
Syntax
- For Loop_counter IN [Reverse]
- Lower_Limit . . Upper_Limit Loop
- Statement 1;
- statement 2;
- statement 3;
- …
- END Loop;
- Set Serveroutput ON;
- Begin
- For v_counter IN 1 . . 10 Loop
- DBMS_OUTPUT.PUT_LINE(v_counter);
- END Loop;
- End;
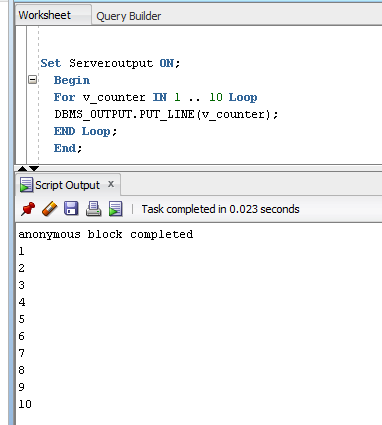
For Loop in Reverse Order
- Set serveroutput ON;
- Begin
- for v_counter in reverse 1 .. 10 loop
- DBMS_output.put_line(v_counter);
- End Loop;
- End;
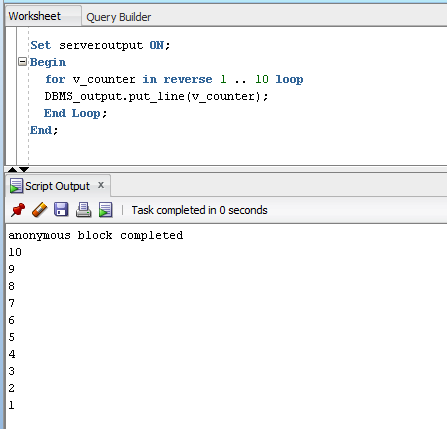
Cursor For Loop
A cursor for loop is used, when you want to fetch the process and all the records in a cursor.
Syntax
- For Loop_Index IN Cursor_name
- Loop
- Statements
- End Loop;
- SET SERVEROUTPUT ON;
- DECLARE
- CURSOR employee_cur IS SELECT emp_id, salary FROM employee;
- BEGIN
- FOR employee IN employee_cur
- LOOP
- DBMS_OUTPUT.PUT_LINE('EMP_Id'||' = '||' salary');
- END LOOP;
- END;
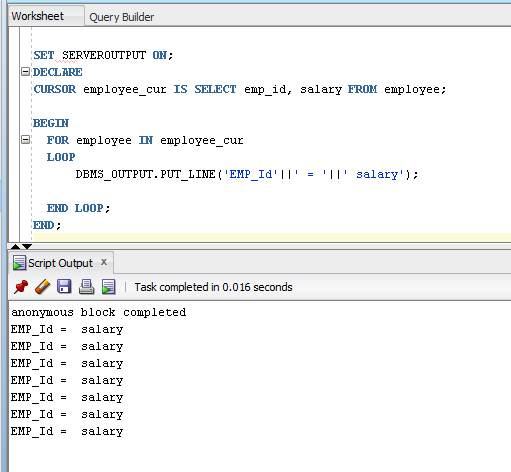
Summary
Thus, we learnt, there are some types of loop in Oracle such as simple loop, while-loop, for-loop, cursor-for-loop. We learnt, how to use these loop statements in Oracle with the examples.