Functions In Python
Introduction
In this article, you will learn that Python supports the user defined function, which increases the code reusability and provides the modularity.
Function is a block of code, used to perform the specific function.
Defining a function
In Python, the user defined function is declared, using ‘ def ’ keyword.
Syntax
def function_name(parameter):
Statements
Parameter is optional.
Example-
- #defining function
- def helloprint():
- print("hello i m inside function")
- #calling
- helloprint()
Output-
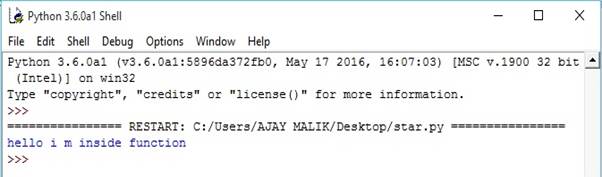
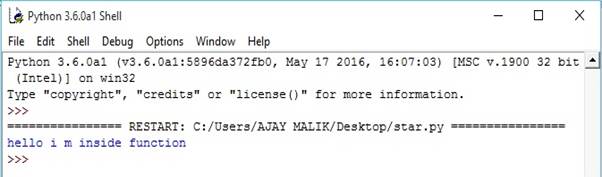
Calling of a function-
Calling is just a use code of our function and it is an important part of our function because our source code executes after calling.
Example-
- def sum(a,b):
- return a+b
- r=sum(5,6) #calling
- print("sum of 5,6 is:",r)
Output-
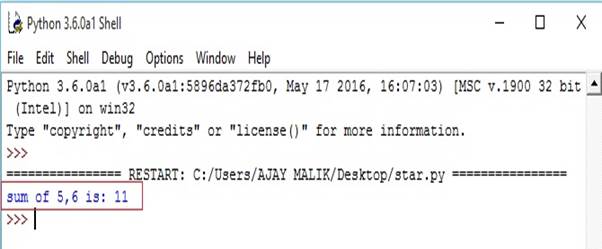
Types of functions are-
- Parameterized function
Parameterized function refers to the function, which is called with an argument or a parameter.
Example-
- def sum(a,b):
- return a+b
- r=sum(5,6) #calling
- print("sum of 5,6 is:",r)
Output-
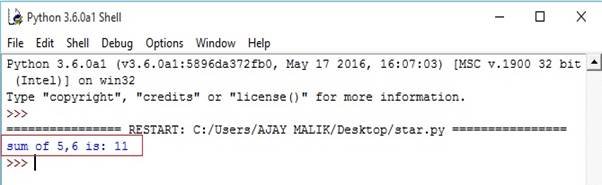
- Parameter less function
Parameter less function refers to the functions to call an argument or parameter not required
Example-
- def sayhello():
- print("hello how are you :)")# calling
- sayhello()
Output-
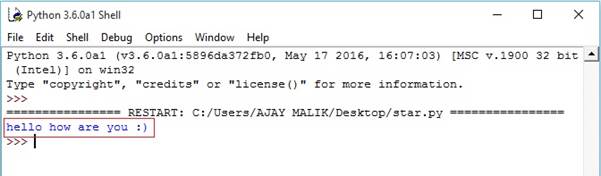
Call by reference
In Python, function argument is by default passed by reference, which means, if you change a parameter in the function and an actual value of the parameter is also changed.
Example-
- list1 = [1, 2, 3, 4, 5, 6]
- def mypop(list2):
- list2.pop()
- print("before calling mypop list1 is:", list1)
- mypop(list1)
- print("after calling mypop list1 is:", list1)
Output-
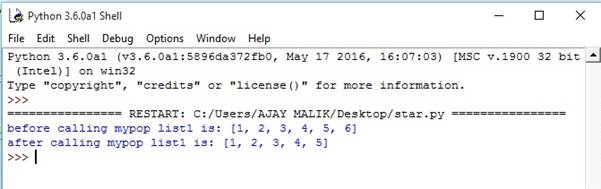
Anonymous function
Anonymous function is defined, using ‘lambda’ keyword. Anonymous functions are like ternary operator in ‘C programming language’. Anonymous function takes any number of an argument but returns only value.
Syntax-
Function _name=lambda arg1,arg2,...,argn:expression
Example-
- add=lambda a,b:a+b # Anonymous function
- #calling
- print("sum of 5 amd 6 is:",add(5,6))
Output-
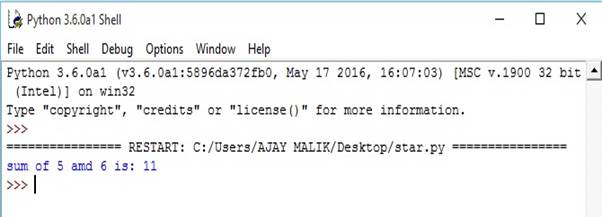
Return statement-
Return statement is used in the function to return the result or an output of the function code.
Syntax-
Return: value
Example-
- def sum(a,b):
- return a+b
- r=sum(5,6) #calling
- print("sum of 5,6 is:",r)
Output-
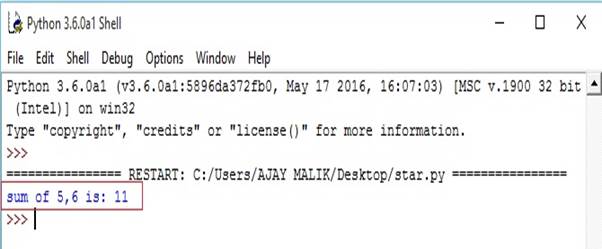
Scope of variable-
Variable is accessible at all the locations in the program. It depends on the declaration location of the variable.
There are two types of scope of variable, which are-
- Global variable-
Access of the global variable is in the entire program, which means the variable is accessed at all the locations in the program
Local variable-
The scope of a local variable is within the block.
Example-
- a=5
- def scope():
- a=4
- print("local variable:",a)# print locall variable
- scope() #calling
- print("global variable:",a) #print global variable
Output-
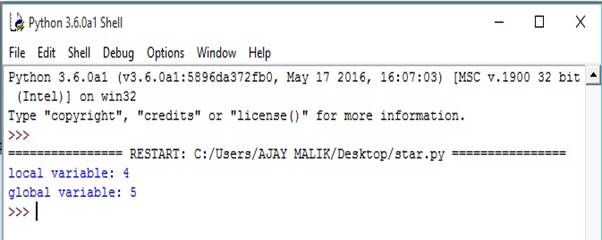
Summary
In this article, you learnt how to create a user defined function and how to call them using call by value and call by reference method.