Variable in Python
Variable
In this Chapter, you will learn what variable is? Variables in Python programming language are the reserved memory location to store value. Thus, you create a variable, which means you reserve the memory space for your variable.
Python interpreter allocates the memory according to the data type of the variable.
Assigning Value to Variable
In Python, assign value to a variable, using equal sign (=)
In Python, the variable is on the left side of equal sign(=) and value is on right side of equal sign(=).
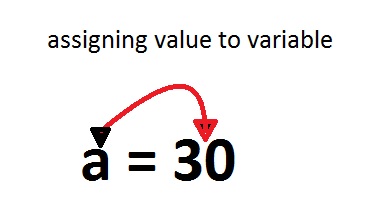
Multiple Assignment
Python allows single value, which is assigned to the multiple variables at same time and within the same line.
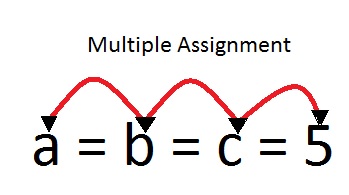
Different value to different variable.
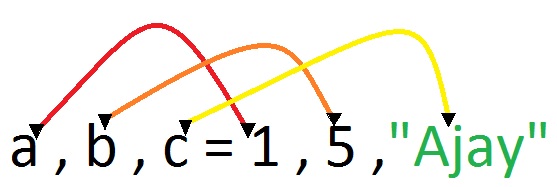
Data type
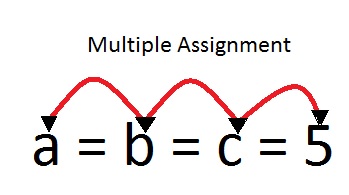
Different value to different variable.
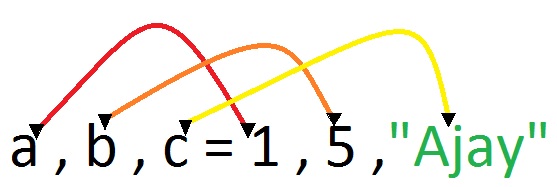
Data type
Python supports many type data type and these data type are used to define operations possible in them.
Following is a list of Python’s data type.
- Numbers
- String
- List
- Tuple
- Dictionary
We will discuss later in detail but here we will only review about the data type.
Python numbers
Numbers data type stores the numeric value.
Numerical type
- int ( signed integer)
- long (octal and hexadecimal)
- float
- complex
Example
- i=2 #integer
- l=999999999999999999999 #long
- f=10.5 #float
- c=3.14j #complex
Python string
Python strings are used to store the character, word and sentence.
In Python, string starts from 0 to (length -1), which means first character of Python string is at zero index.
In Python, string starts from 0 to (length -1), which means first character of Python string is at zero index.
Example
- str1= "hello Python! really you are open source "
- print(str1)
Python list
In Python, list is a versatile data type because list stores the multi type data value over a single list object.
Python list is similar to an array in C and Python’s list multi type value over a single variable but an array only stores a single type value.
- (+) sign is a list concatenation operator.
- (*) asterisk is the repetition operator.
Example
- #list in python
- list1=[1,2,'ajay',2.2]
In Python programming language, tuple is a sequence data type like list but tuple reads only list, which means element and size of tuple cannot be changed.
Example
Example
- #python tuple
- t=(1,2,3,4,'ajay')
Python dictionary
Python dictionary is the special type data type with the key value.
Dictionary is the key-value pair.
Example
- #dictionary in python
- tup={'name':'ajay','ph no.':'9999999999'}
Data type conversion (type casting)
Data type casting is a conversion of one data type to another data type.
In Python, several functions are available for type casting.
In Python, several functions are available for type casting.
They are listed below.
Function | Description |
int(n) | Convert n to an integer |
Long(n) | Converts n to a long |
float(n) | Converts n to a float |
complex(real[imag]) | Creates a complex number |
str(n) | Converts an object n to string |
repr(n) | Converts an object n to an expression string |
eval(str) | Evaluates a string and return an object |
tuple(n) | Converts n to tuple |
list(n) | Converts n to list |
set(n) | Converts s to a set |
dict(n) | Creates a dictionary n must be a sequence of (key, value) tuple |
frozenset(n) | Converts n to frozen set |
chr(n) | Converts an integer to a character |
unichar(n) | Converts n integer to Unicode character |
ord(n) | Converts a single character to its integer value |
hex(n) | Converts an integer to hexadecimal string |
oct(n) | Converts an integer to an octal string |