Decision Making
Introduction
In this article, you will learn that decision making is an expectation condition, which occurs at the time of execution of the program and decision making is used to control the flow to the program, which means decision making is used to execute the statement, according to the particular condition.
Decision making statement works on true or false.
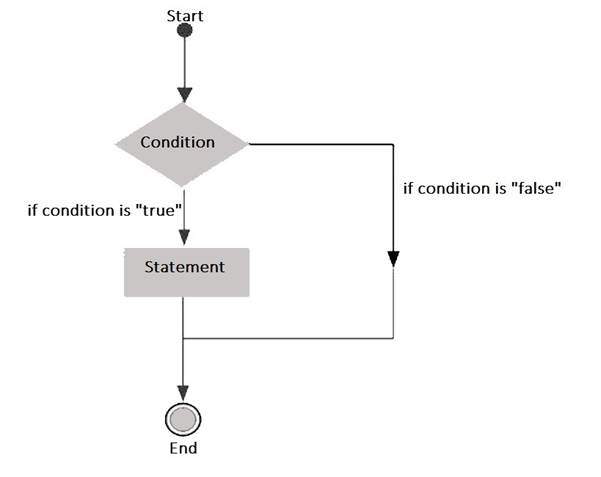
Decision making executes the statement, when the condition is true.
Python supports (decision making) statement, given below.
- if statement
- if else statement
- elif statement
- nested if statement
if statement
If statement contains a logical expression and a logical expression is compared for the execution of statement inside the “if statement” block.
Flow chart of “if statement” is given below.
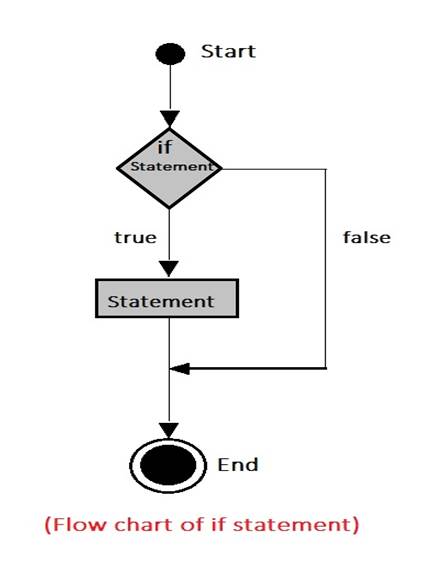
Syntax of “if statement”
if condition(c):
statement(s)
Only statement is executed, when the condition statement (c) is “true”.
Otherwise statement (s) is skipped.
Example
- a= 100
- if a:
- print("true expression value")
- print(a)
- b= 0
- if b:
- print("true expression value")
- print(b)
Output
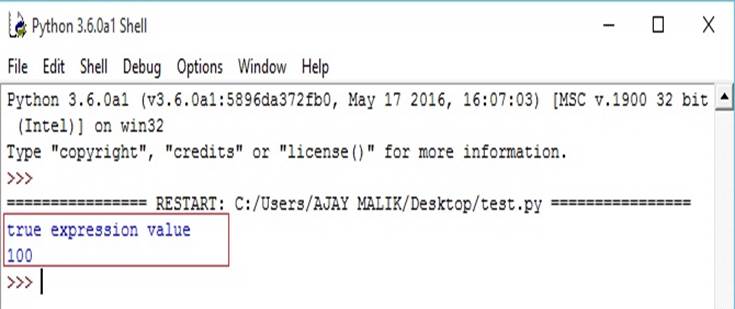
Explanation
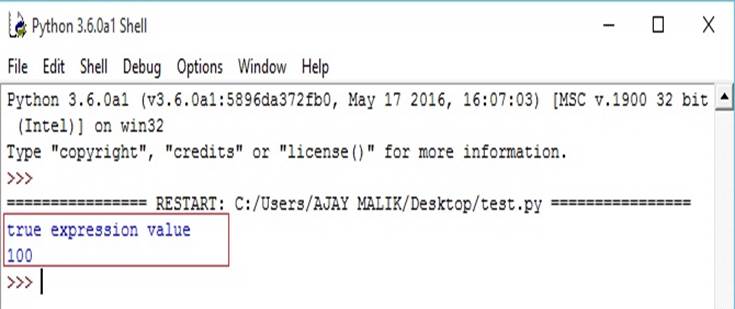
Explanation
a=100, condition is true because in Python, every non zero condition is true and it prints the statement.
b=0, condition is false because in Python, every zero condition is false and skips the statement.
if else statement
If else statement in Python is used to execute the statement, according to the condition. Else is executed, when the condition of “if statement” is false.
Flowchart
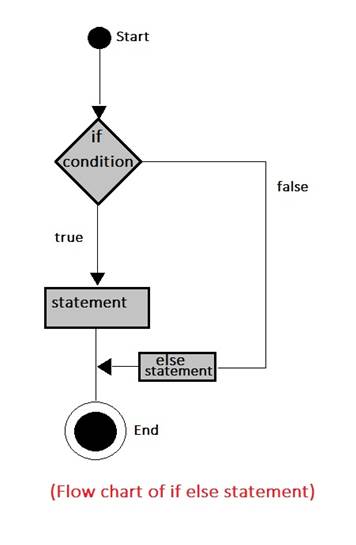
Syntax
If condition(c):
Statement(s1)
else:
statement(s2)
statement(s1) is executed, when the condition(c) is “true” and statement(s2) is executed, when the condition(c) is “false”.
Example
- n=10
- if n%2==0:
- print("n is even number")
- else:
- print("n is odd")
Output
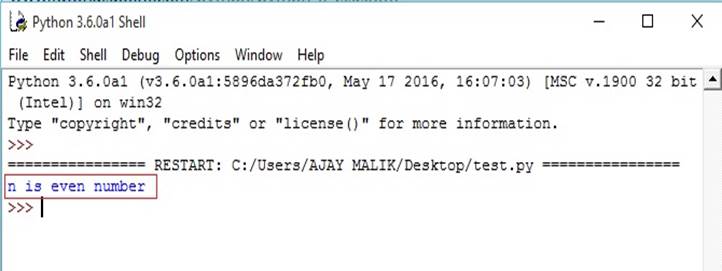
Explanation
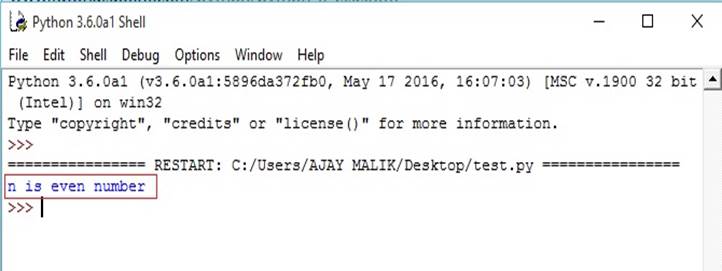
Explanation
In the example, shown above, n=10 checks the condition of if statement, its true and print “n is even” and if statement is false, else statement is executed.
elif statement
We can say “elif” statement as a ladder. It’s used to execute one statement among the many statements, according to the condition.
Flow chart
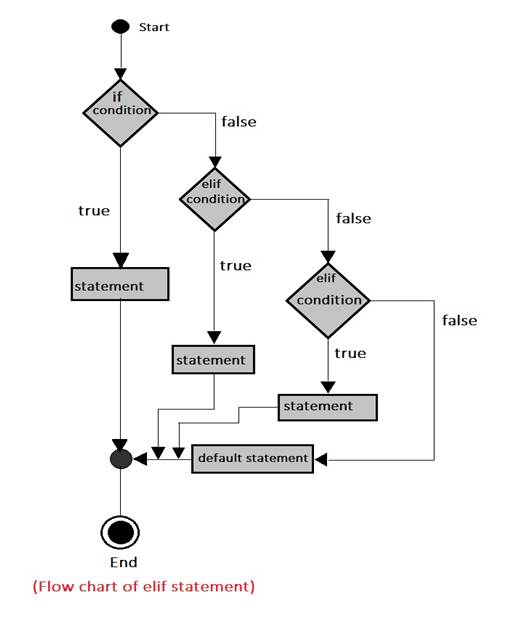
Syntax of elif statement
if condition(c1):
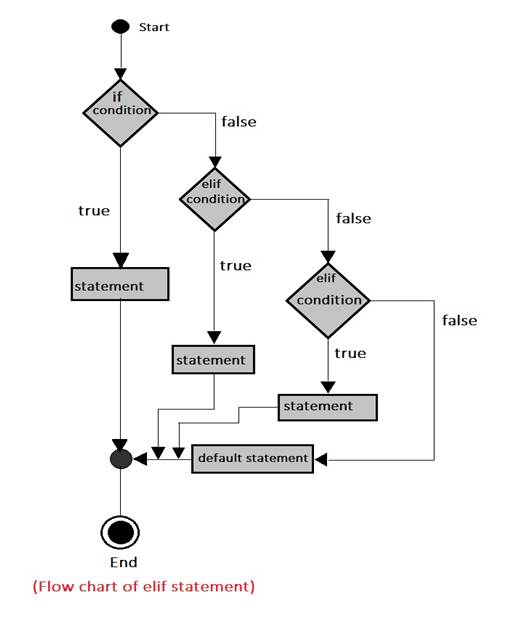
Syntax of elif statement
if condition(c1):
statement(s1)
elif condition(c2):
statement(s2)
elif condition(c3)
statement(s3)
else:
statement(s4)
Example
- n = int(input("enter a month number"))
- if n == 1:
- print("jan")
- elif n == 2:
- print("feb")
- elif n == 3:
- print("march")
- elif n == 4:
- print("april")
- elif n == 5:
- print("may")
- elif n == 6:
- print("june")
- elif n == 7:
- print("july")
- elif n == 8:
- print("august")
- elif n == 9:
- print("september")
- elif n == 10:
- print("october")
- elif n == 11:
- print("november")
- elif n == 12:
- print("december")
- else :
- print("wrong input try again!")
Output
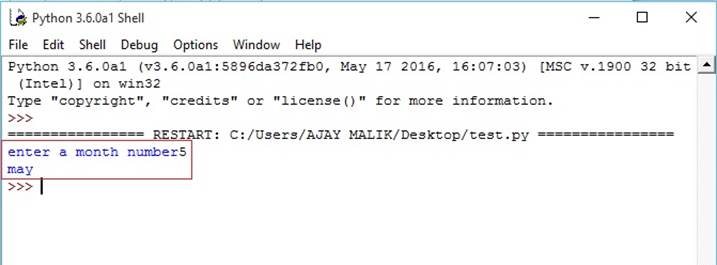
Explanation
The program, given above, prints the month, according to an input.
elif checks the condition, until condition is not satisfied and when the condition is satisfied, it terminates whole “elif” statement
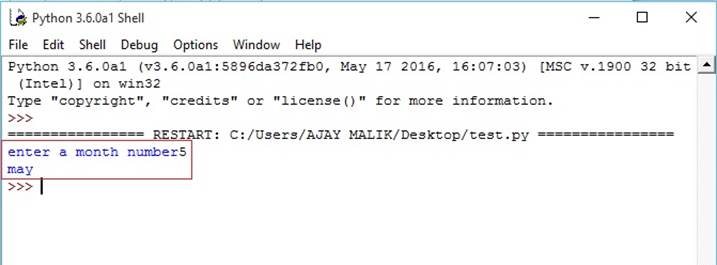
Explanation
The program, given above, prints the month, according to an input.
elif checks the condition, until condition is not satisfied and when the condition is satisfied, it terminates whole “elif” statement
Nested if statement
Nested if statement is “if statement inside the if statement”. It checks the condition’s upper condition and if it is true, then inside, if condition is checked.
Flowchart
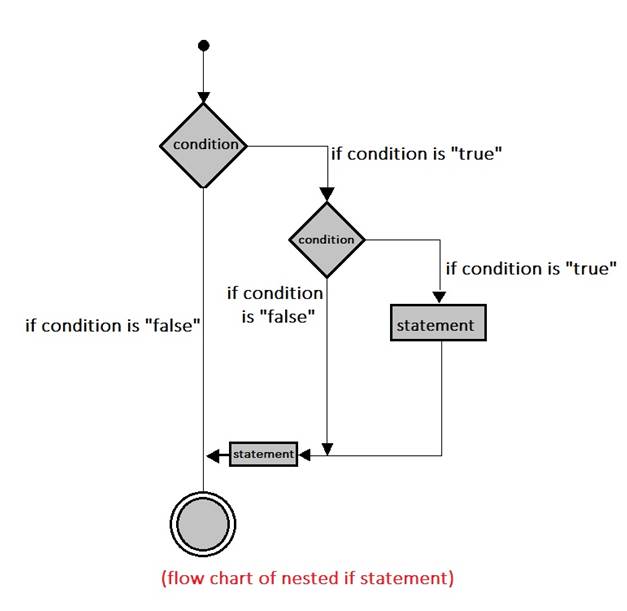
Syntax
if condition:
statement
if condition:
statement
else:
statement
Example
- n = int(input("enter a number"))
- if n % 2 == 0:
- print("even number")
- if n > 0:
- print("\npositive number")
- else :
- print("\nnegative number")
- else :
- print("odd number")
Output
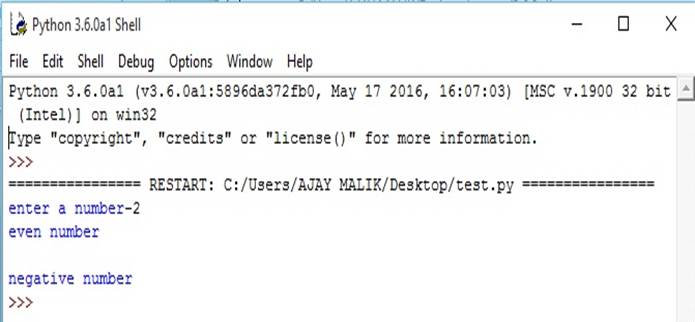
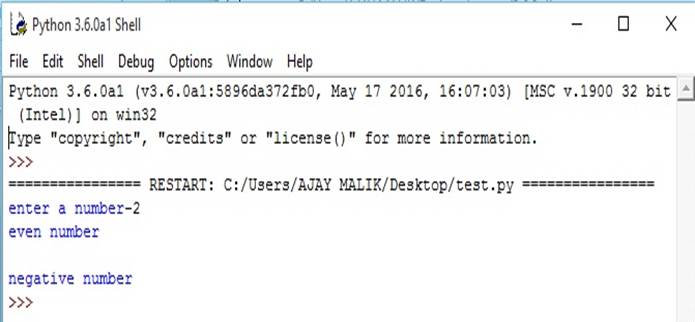
Explanation
The program, given above, first checks the condition for even or odd, if the number is even then “inner if” statement checks whether the number is negative or positive.
Summary
In this article, you learnt how to use decision making statement in Python.