Python Dictionary
Introduction
In this article, we will learn about one of the most important data type, which is dictionary. The dictionary contains the two values first ‘key’ and another is value, separated by colon (:) and a key-value pair is written in { }. Key is unique and a value can be repeated. Dictionary data type is easy to traverse and easy to find the value.
Syntax-
Dictionary_name={‘key’:’value’,’key’:’value’}
Example-
- dict1={'name':'ajay','skill':'python'}
Accessing value in the dictionary-
To access the value of the dictionary, we can use the key along with a square bracket.
Example-
- dict1={'name':'ajay','skill':'python'} #list definition
- print("name:",dict1['name']) #print name
- print("skill:",dict1['skill']) #print skill
Output-
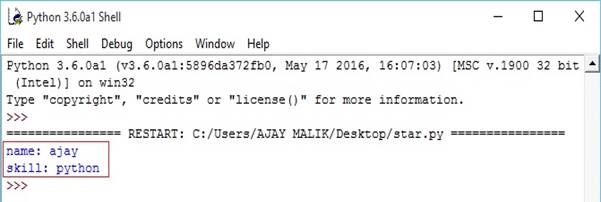
Updating dictionary-
We can update an existing value, using the key and we can also add a new pair of the key and value.
Example-
- dict1={'name':'ajay','skill':'python'}
- print("dictionary before change: ",dict1) #change exist item
- dict1['skill']='c#' #change existing element
- print("dictionary after change: ",dict1) #add new entry
- dict1['exp']=2 #add new key and value pair
- print("after add new element: ",dict1)
Output-
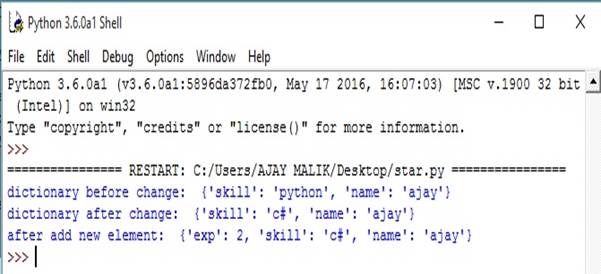
Deleting Dictionary element-
We can delete an individual element or whole dictionary and empty dictionary.
Example-
- dict1={'name':'ajay','skill':'python'}
- print("dictionary before delete: ",dict1) #delete individual element
- del dict1['skill']
- print("after delete individual element:",dict1) #clear dict1
- dict1.clear()
- print("after clear:",dict1) #delete whole dict1
- del dict1
- print("after deleting whole dict1:",dict1)
Output-
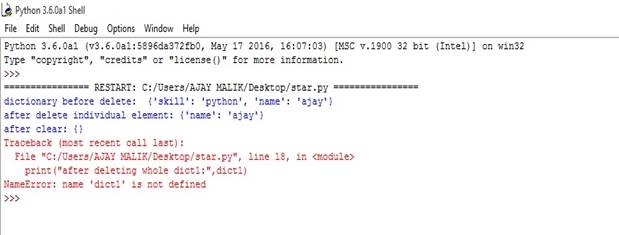
Built-in Dictionary functions are listed below-
len(dict)
Returns the total number of items, existing in the dictionary.
Syntax-
len(dict)
return value- number (length of dictionary)
Example-
- dict1={'name':'ajay','skill':'python'}
- print("length of dict1 is:",len(dict1)) # calculating length
Output-
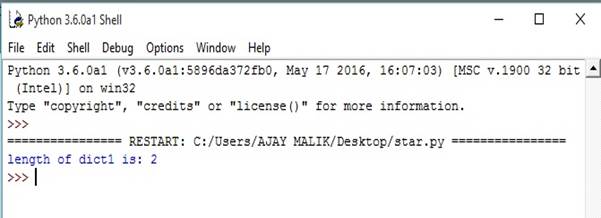
str(dict)
It converts dictionary to string
Syntax-
str(dict)
return value- return string
Example-
- dict1={'name':'ajay','skill':'python'}
- str1=str(dict1) #convert dict1 to string
- print("string",str1)
Output-
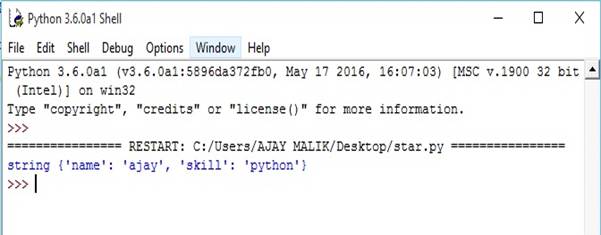
type()
Type function returns the variable data type.
Syntax-
type(dict)
return value- It returns the datatype of passed variable.
Example-
- dict1={'name':'ajay','skill':'python'}
- print("datatype of dict is:",type(dict1))
Output-
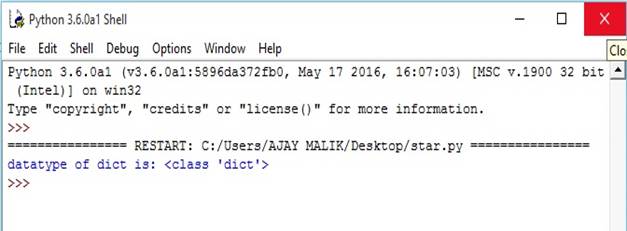
Other dictionary functions are-
dict.clear()
It removes all the elements of dictionary.
Syntax-
dict_name.clear()
return- no return
Example-
- dict1={'name':'ajay','ph':9990600495}
- print("dict1 before execute dict1.clear: ",dict1)
- dict1.clear()
- print("dict1 after execute dict1.clear: ",dict1)
Output-
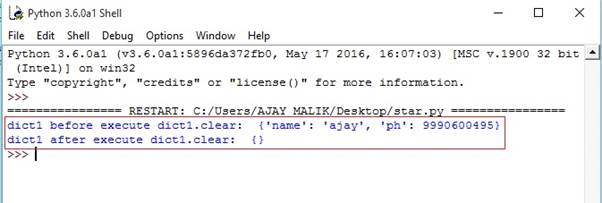
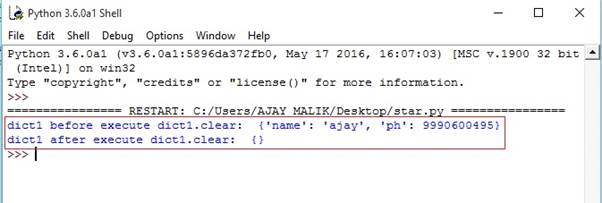
dict.copy()
It copies dictionary and returns a new copy of dictionary.
Syntax-
dict2=dict1.copy()
return- return dictionary
Example-
- dict1={'name':'ajay','ph':9990600495} #copy dict1 in dict2
- dict2=dict1.copy()
- print("dict1: ",dict1)
- print("dict2: ",dict2)
Output-
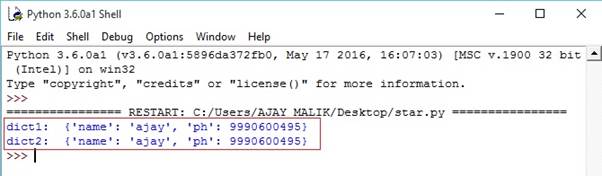
dict.fromkeys()
It creates a new dictionary with the keys and default value set to zero ‘0’.
Syntax-
dict_name=dict.fromkey(seq,values)
return- dictionary
Example-
- seq1=['name','age','sex']
- dict1=dict.fromkeys(seq1)
- print("dict1 :",dict1)
Output-
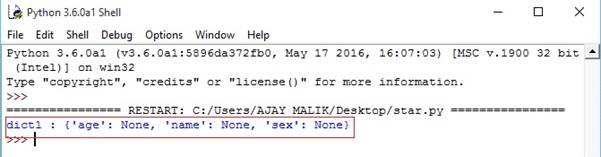
dict.key(key)
It returns the value of the given key and the key is not passed. It returns none.
Syntax-
Variable_name=dict_name.get(key)
Return- It returns the value of the given key.
Example-
- dict1={'name':'ajay malik','sex':'male'}
- print("value at name key are:",dict1.get('name'))
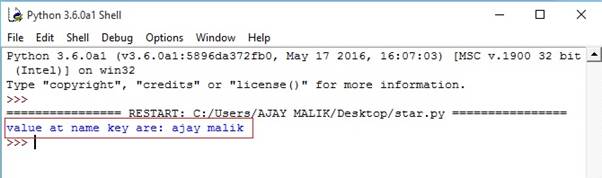
dict.keys()
This method returns the keys of dictionary.
Syntax-
Dict_name.keys()
Return- Dictionary key.
Example-
- dict1={'name':'Ajay','Age':23}
- print("keys in dict1: ",dict1.keys())
Output-
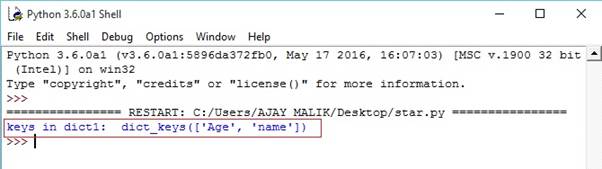
dict1.values()
It returns the list of all the values of dictionary.
Syntax-
dict.values()
Return- return values
Example-
- dict1={'name':'Ajay','Age':23}
- print("values in dict1: ",dict1.values())
Output-
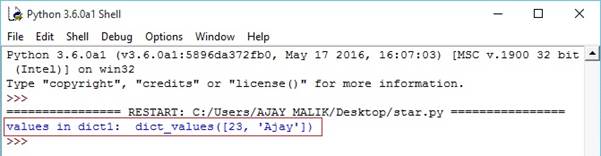
Summary
In this article, you learnt how to create dictionary, adding key value pair into dictionary and you also friendly about predefine function of dictionary.