Class And Object In Python
In this chapter, you will learn about class and object in Python. Python is pure object Oriented programming language since they exist and very simple to implemented OOPS feature
Creating of class
In Python, class is created, using the class keyword.
In Python, class is created, using the class keyword.
Syntax-
class class_name:
class_funtion
Example-
- class student:
- def _init_(self,name,course):
- self.name=name
- self.course=course
- def printf(self):
- print("name ",self.name)
- print("course ",self.course)
The class is successfully created.
def _init_(self,name,course)- This method is a class constructor and it is called automatically, when we create an instance(object) of this class.
def printf(self)- This method is user defined function. This method is defined to print the values of data members.
Creating instance(object)
Create an instance, which is just like a declaration of the variable.
Syntax-
object_name=class_name(data_member)
Example-
- stu=student(“Ajay malik”,”B.tech”)
- stu1=Student(“Rohit tomar”,”MCA”)
Accessing Attributes
In Python, to access the member function and data member, use the syntax, given below-
Syntax-
stu.prinf()
In Python, to access the member function and data member, use the syntax, given below-
Syntax-
stu.prinf()
stu1.pritf()
Now, we learnt creating a class, creating of an object and accessing of an attribute of class, so take an example for better understanding.
Example-
Now, we learnt creating a class, creating of an object and accessing of an attribute of class, so take an example for better understanding.
Example-
- class student:
- def __init__(self,name,course):
- self.name=name
- self.course=course
- def printf(self):
- print("name ",self.name)
- print("course ",self.course)
- stu1=student("Ajay Malik","B.tech")
- stu2=student("Rohit Tomar","MCA")
- stu1.printf()
Output-
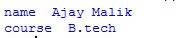
Built in function for accessing attribute of class
Thre are listed below
- getattr(obj, name[, default]) is used to access all the attributes of the class.
- hasattr(obj,name) checks whether an attribute exits or not.
- setattr(obj,name,value) changes the attribute value.
- delattr(obj, name) is required to delete an attribute.
- class student:
- def __init__(self,name,course):
- self.name=name
- self.course=course
- def printf(self):
- print("name ",self.name)
- print("course ",self.course)
- stu1=student("Ajay Malik","B.tech")
- stu2=student("Rohit Tomar","MCA")
- stu1.printf()
- stu2.printf()
- print(hasattr(stu1, 'name'))
- print(getattr(stu2, 'name'))
- setattr(stu2, 'course', "b.tech")
- delattr(stu2, 'course')
Output-
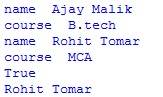
Built-In Class Attributes
In Python programming language, class has all the attribute and they can be used dot(.) with class_name
- __dict__: Dictionary contains the class namespace.
- __doc__: Class documentation string or none if it is not defined.
- __name__: Class name.
- __module__: Module name in which the class is defined.
- __bases__: Used to return base class list.
- class student:
- def __init__(self,name,course):
- self.name=name
- self.course=course
- def printf(self):
- print("name ",self.name)
- print("course ",self.course)
- stu1=student("Ajay Malik","B.tech")
- stu2=student("Rohit Tomar","MCA")
- stu1.printf()
- stu2.printf()
- print ("student.__doc__:", student.__doc__)
- print ("student.__name__:", student.__name__)
- print ("student.__module__:", student.__module__)
- print ("student.__bases__:", student.__bases__)
- print ("student.__dict__:", student.__dict__ )
Output-
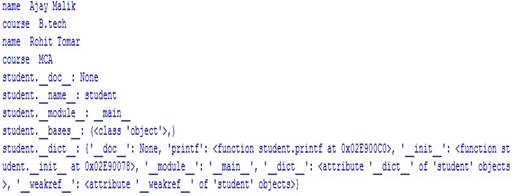
Class Inheritance
Inheritance transfers the property of parent class to base class.
A child class inherits all the attribute of parent class.
Syntax-
class child_class_name(parent_class_name):
class_members
Example-
Example-
- class base:
- def __init__(self):
- print("base class constructor call")
- def b_prinf(self):
- print("base class printf method call")
- class drived(base):
- def __init__(self):
- print("drived class constructor call")
- def d_prinf(self):
- print("drived class printf method call")
- obj=drived()
- obj.b_prinf()
- obj.d_prinf()
Output-
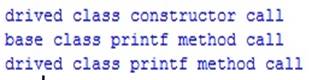
Overriding Methods
In Python, we can also override the function of base class into derived class, in order to change the functionality in derived class.
Example-
- class base:
- def __init__(self):
- print("base class constructor call")
- def prinf(self):
- print("base class printf method call")
- class drived(base):
- def __init__(self):
- print("drived class constructor call")
- def prinf(self):
- print("drived class printf method call")
- obj=drived()
- obj.prinf()
Output
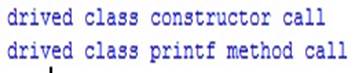
Base Overloading Methods
They are listed below-
- __init__ ( self [,args...] ) Constructor (with any optional arguments) Call : obj = className(args)
- __del__( self ) Destructor, deletes an object Call : del obj
- __repr__( self ) Evaluates the string representation Call : repr(obj)
- _str__( self ) Printable string representation Call : str(obj)
- __cmp__ ( self, x ) Object comparison , Call : cmp(obj, x)
In this chapter, you learnt OOPS programming concepts, how to create a class in Python and how to use a function of a class.