String In Python
Introduction
String is the most useable data type in Python, because the string stores every type value like number, character and also stores the mathematical expression.
String data type stores every type value as a string. For example, we store a number value but we want to perform a mathematical operation. Thus, we do type conversion (type casting) and we are able to perform the operations.
Example
- str1=input("enter a number")
- str2=input("again enter a number")
- a=int(str1)
- b=int(str2)
- c=a+b
- print("sum of",str1,"&",str2,"is",c)
Output
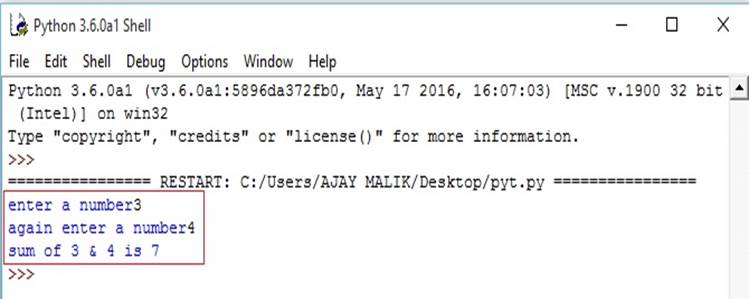
Explanation
In this program, we enter a number used in the string format but we perform a mathematical operation on the string, so we type cast string into int type variable and then perform an addition operation on an integer variable and finally print the result.
In this program, we convert our string type into an integer type, using int class.
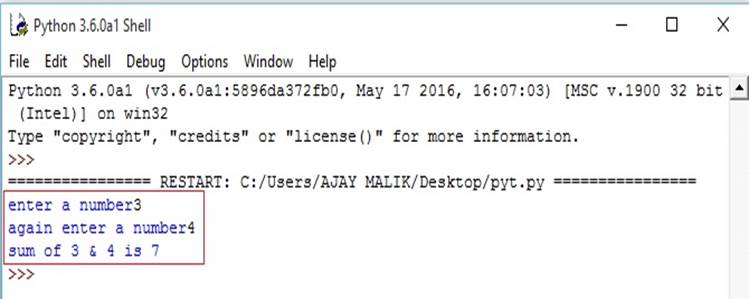
Explanation
In this program, we enter a number used in the string format but we perform a mathematical operation on the string, so we type cast string into int type variable and then perform an addition operation on an integer variable and finally print the result.
In this program, we convert our string type into an integer type, using int class.
Structure of string in Python
Python does not have a character type. In Python, a single character is also stored in the string type.
In Python, the string is stored in the sequence, which means, if we want to store a string “c#corner”, first character is stored in an index 0 and last character is stored in n-1 index (n is the length of the string).
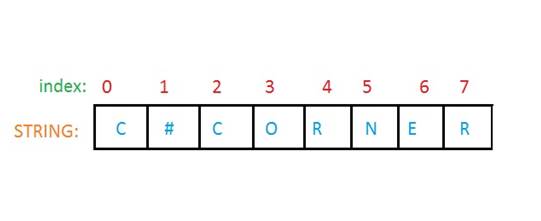
Example
- str=input("enter a string")
- print("element on index[0] is",str[0]) # print first element
- print("string is ",str) # print whole string
- print("element index 1 to 4",str[1:5]) #print element is range
Output
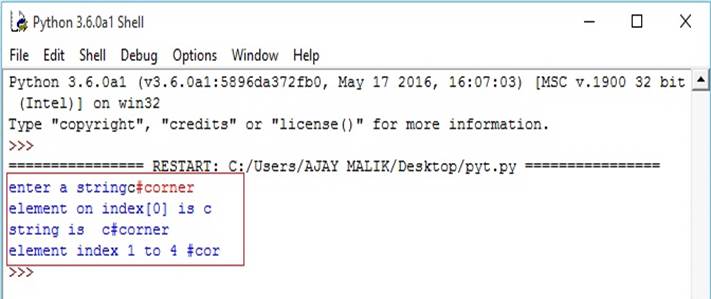
Updating strings
In Python, we can update the string or we can say change a particular word with another word.
Example
- str1=input("enter a string")
- print("\nstring=",str1)
- str2=input("\nenter string to update")
- str1=str1[:6]+str2
- print("\nafter update=",str1)
Output
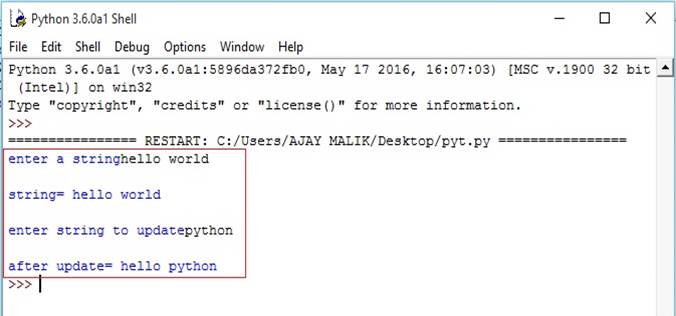
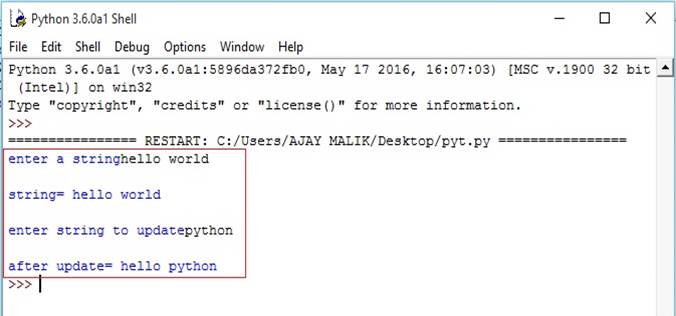
String operators
String operators are used to perform an operation on the string. Some string operators are listed below.
String operators are used to perform an operation on the string. Some string operators are listed below.
Let string str1=”c#” and str2=”corner”
Operator | Description | Example |
+ | Concatenation-join strings into one string | Str3=str1+str2 Str3 is “c#corner” |
* | Repetition-multiple same string into new string | Str3=str1*2 Str3 is “c#c# |
[] | Slice is used to find the character according to an index | Str2[0]-> gives ‘c’ |
[:] | Range Slice-finds the character in the range | Str2[1:4]->gives ‘orn’ |
In | Membership-it is used to find the given character, which exists in the string and returns ‘true’, | if the character is found in the string In string str2, n is present and gives 1 |
not in | Membership-it is used to find the given character, which does not exist in the string and returns ‘true’, if the character is not found in the string | In string, str2 j is not present and gives 1 |
For example, Concatenation operator ( + )
- str1="c#"
- str2="corner"
- str3=str1+str2
- print("str1 is: ",str1)
- print("str2 is : ",str2)
- print("After Concatenation string is:",str3)
Output
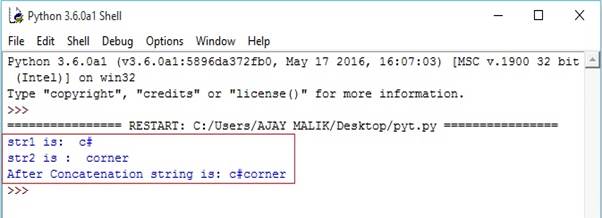
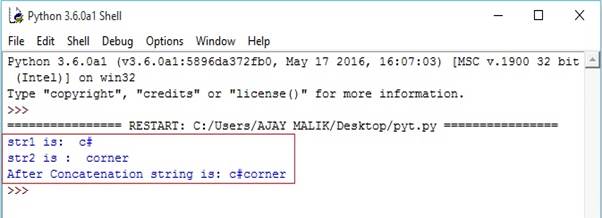
Example of Repetition operator
- str1="c#"
- str2=str1*2
- print("str1 is : ",str1)
- print("After Repetition:",str2)
Output
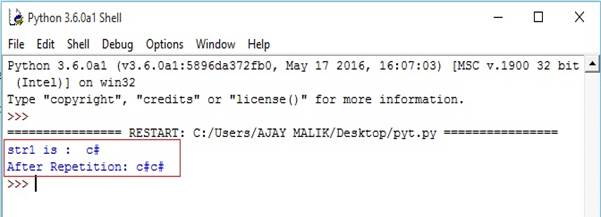
Example of slice operator
- str1="c#corner"
- print("chatacter at index 0 :",str1[0])
- print("character at index 3: ",str1[3])
- print("character at index 6: ",str1[6])
Output
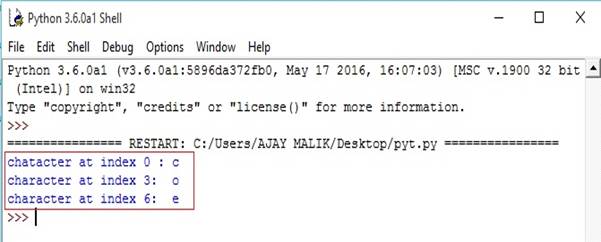
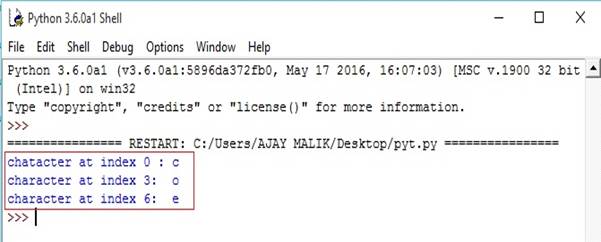
Example of range slice operator
- str1="c#corner"
- print("string is: ",str1)
- print("string in range[1:4] is : ",str1[1:4])
- print("string in range[3:5] is :",str1[3:5])
Output
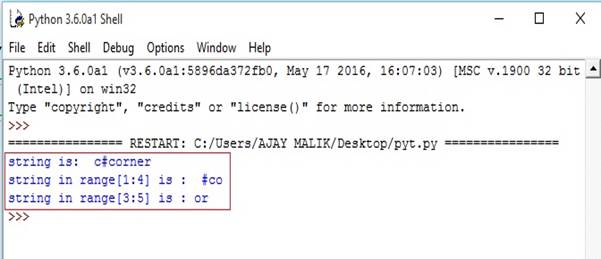
Example of in operator
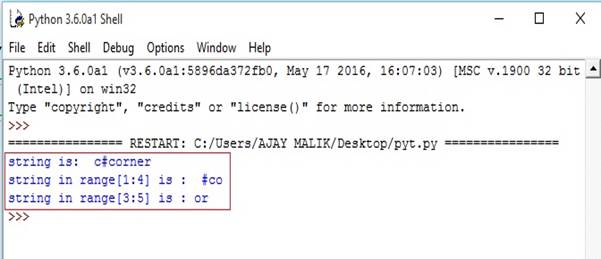
Example of in operator
- str1="c#corner"
- n1= 'r' in str1
- print("r in found in string:",n1)
- n2='j' in str1
- print("j is found in string:",n2)
- n3='corner' in str1
- print("corner is found in string:",n3)
Output
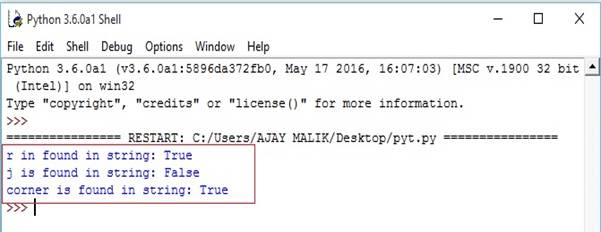
Example of “for not in operator”
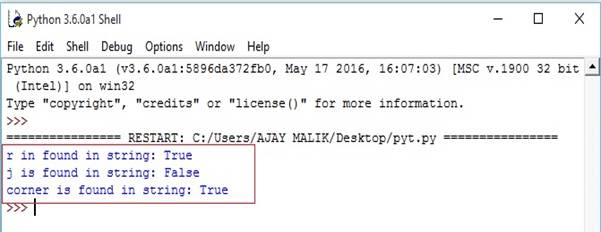
Example of “for not in operator”
- str1="c#corner"
- n1= 'r' not in str1
- print("r in found in string:",n1)
- n2='j' not in str1
- print("j is found in string:",n2)
- n3='corner'not in str1
- print("corner is found in string:",n3)
Output
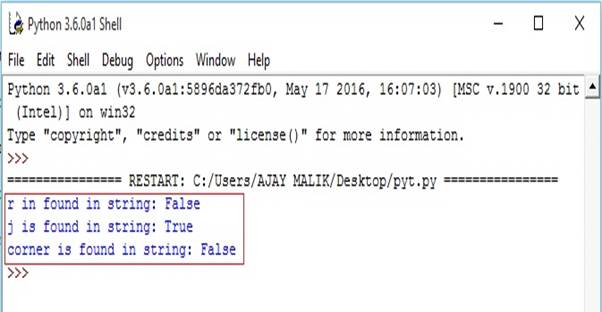
Escape character
Escape character is the non printable character, which means it is not print on screen while we write in print() function but they having a special means that can be make a special character and used for different purpose.
They are listed below.
Backslash notation | Description | Hexadecimal character |
\b | Backspace | 0x08 |
\a | Alert or Bell | 0x07 |
\e | Escape | 0x1b |
\f | Formfeed | 0x0c |
\cx | Control-x | |
\C-x | Control-x | |
\M-\C-x | Meta-Control-x | |
\n | Newline | 0x0a |
\nnn | Octal notation, where n is in the range 0.7 | |
\r | Carriage return | 0x0d |
\s | Space | 0x20 |
\t | Tab | 0x09 |
\v | Vertical tab | 0x0b |
\x | Character x | |
\xnn | Hexadecimal notation, where n is in the range 0.9, a.f, or A.F |
Escape character is interpreted in single (‘ ‘) or double quoted (“ “) strings
Example
- print(“hello guys ? \awhats up!”)
String formatting operator
String formatting is one of the most important feature of Python programming language, which is using string format operator %.
Using this operator, Python’s print() function performs like printf() function of C programming language.
Example
- str1="c# corner"
- n=400
- print("%s has %d article of Angular js"%(str1,n))
Output
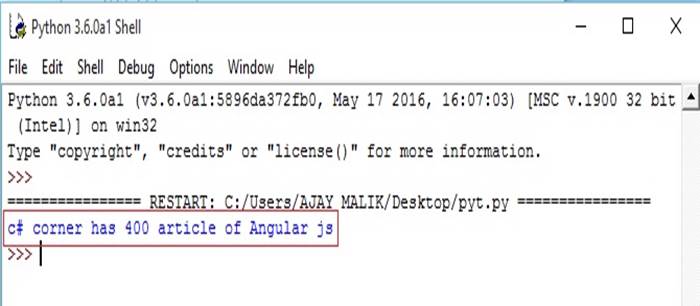
String formatting operators are listed below
Format Symbol | Conversion |
%c | character |
%s | string conversion via str() prior to formatting |
%i | signed decimal integer |
%d | signed decimal integer |
%u | unsigned decimal integer |
%o | octal integer |
%x | hexadecimal integer (lowercase letters) |
%X | hexadecimal integer (UPPERcase letters) |
%e | exponential notation (with lowercase 'e') |
%E | exponential notation (with UPPERcase 'E') |
%f | floating point real number |
%g | the shorter of %f and %e |
%G | the shorter of %f and %E |
Built-in string method
Python built-in method or function is used to perform an operation on the string or we can say to manipulate the string.
There are following built-in functions
Sr.no | |
1 | capitalize() change string first letter lower to upper |
2 | center(width, fillchar) - Returns a space-padded string with the original string centered to a total of width columns. |
3 | count(str, beg= 0,end=len(string)) Counts how many times str occurs in string or in a substring of string if starting index beg and ending index end are given. |
4 | decode(encoding='UTF-8',errors='strict') Decodes the string using the codec registered for encoding. encoding defaults to the default string encoding. |
5 | encode(encoding='UTF-8',errors='strict') Returns encoded string version of string; on error, default is to raise a ValueError unless errors is given with 'ignore' or 'replace'. |
6 | endswith(suffix, beg=0, end=len(string)) Determines if string or a substring of string (if starting index beg and ending index end are given) ends with suffix; returns true if so and false otherwise. |
7 |
expandtabs(tabsize=8) Expands tabs in string to multiple spaces; defaults to 8 spaces per tab if tabsize not provided.
|
8 | find(str, beg=0 end=len(string)) Determine if str occurs in string or in a substring of string if starting index beg and ending index end are given returns index if found and -1 otherwise. |
9 | index(str, beg=0, end=len(string)) Same as find(), but raises an exception if str not found. |
10 | isalnum() Returns true if string contains numerical and alphabet. |
11 |
isalpha() Returns true if string contains all alphabet otherwise false.
Whitespace also consider a non alphabet.
|
12 |
isdigit() Returns true if string contains all numerical type otherwise return false.
|
13 | islower() Returns true, if string contains all lowercase characters |
14 | isnumeric() Returns true, if string contains all numerical type otherwise otherwise false. |
15 | isspace() Returns true, if the string contains only whitespace otherwise return false. |
16 | istitle() Returns true, if the string contains first letter of ever word’s is uppercase otherwise false. |
17 | isupper() Returns true, if the string contains all uppercase alphabets. |
18 | join(seq) merges (concatenates) the string representations of elements in sequence seq into a string, with separator string. |
19 | len(string) returns the length of the string. |
20 | ljust(width[, fillchar]) Returns a space-padded string with the original string left-justified to a total of width columns. |
21 | lower() Converts all uppercase letters to lowercase of the string |
22 |
lstrip() Removes all leading whitespace from the string.
|
23 | maketrans() Returns a translation table to be used in translate function. |
24 | max(str) Returns the max alphabetical character from the string str. |
25 | min(str) Returns the min alphabetical character from the string str. |
26 | replace(old, new [, max]) replaces all the occurrences of old in the string with new or at most max occurrences, if max is given. |
27 | rfind(str, beg=0,end=len(string)) Same as find(), but searches backwards in the string. |
28 | rindex( str, beg=0, end=len(string)) Same as index(), but searches backwards in the string. |
29 | rjust(width,[, fillchar]) Returns a space-padded string with the original string right-justified to a total of the width columns. |
30 | rstrip() Removes all the trailing whitespace of the string. |
31 | split(str="", num=string.count(str)) splits the string according to delimiter str (space if not provided) and returns the list of the substrings; split into at most num substrings if given. |
32 | splitlines( num=string.count('\n')) splits the string at all (or num) NEWLINEs and returns a list of each line with NEWLINEs removed. |
33 | startswith(str, beg=0,end=len(string)) Determines if the string or a substring of string (if starting index beg and ending index end are given) starts with substring str; returns true if so and false otherwise. |
34 | strip([chars]) performs both lstrip() and rstrip() on string. |
35 | swapcase() inverts case for all letters in string. |
36 | title() returns "titlecased" version of the string, that is, all the words begin with an uppercase and the rest are lowercase |
37 | translate(table, deletechars="") translates the string according to translation table str(256 chars), removing those in the del string. |
38 |
upper() converts lowercase letters in the string to uppercase.
|
39 | zfill (width) returns original string leftpadded with zeros to a total of width characters intended for numbers, zfill() retains any sign given (less one zero). |
40 | isdecimal() returns true, if a unicode string contains only decimal characters and false otherwise. |
Examples of some built-in functions.
capitalize()
- str=”hello python”
- print(“str. capitalize():”str.capitalize)
isdecimal()
- str=”9999999 99999”
- print(“only digit:”,str.isdecimal())
All in built-in functions execute, using syntax, given below.
stringname.function()
Summary
In this chapter, you learnt Python string in detail like how to create Python string and you also learnt about the predefined string function.