Tkinter Widget-Scale
Scale widget is used to create a graphical slider object that can be used to select the values from a specific scale.
Syntax-
w = Scale ( main, option, ... )
Parameter-
Main- parent Window.
Option- attribute of scale widget.
Example-
- from tkinter import *
- def sel():
- selection = "Value = " + str(var.get())
- label.config(text = selection)
- main = Tk()
- var = DoubleVar()
- scale = Scale( main, variable = var )
- scale.pack(anchor=CENTER)
- button = Button(main, text="Get Value", command=sel)
- button.pack(anchor=CENTER)
- label = Label(main)
- label.pack()
- main.mainloop()
Output-
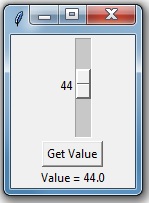
Option | Description |
activebackground | It sets background color of widget when widget is under the cursor. |
Bg | Normal background color. |
Bd | It sets the border width in pixels. Default is 2. |
command | It is used to call function or method |
cursor | Cursor is used to create a cursor in widget like arrow, circle, dot etc. |
font | It is used to change the font of text |
fg | It sets the normal foreground (text) color of widgets. |
from_ | It is used to define the range of scale in int or float. |
highlightbackground | It is used to change the highlight background color of widgets. |
highlightcolor | It changes the color of widgets on focus. |
label | It is used to set the label. |
length | It is used to set the length of scale |
orient | It is used to set the orient of scale like vertical or horizontal. |
relief | Relief is special type of border. The values are SUNKEN, RAISED, GROOVE and RIDGE. |
repeatdelay | This option controls how long button 1 has to be held down in the trough before the slider starts moving in that direction repeatedly. Default is repeatdelay=300 and the units are milliseconds. |
resolution | Used to set the resolution of scale. |
showvalue | Used to set the value on label of scale. |
sliderlength | It is used to define the slider length. |
state | It is used to define the state of widgets. |
takefocus | It is used to set the focus on a particular value. |
tickinterval | It is used to define interval on scale. |
to | It is used to define a range of scale. |
troughcolor | It is used to set color of the trough. |
variable | It is used to define the value of scale. |
width | It is used to define the width of widgets. |
Summary
In this chapter, you learnt what Scale widget is and how to use the property of the widget.