Fundamentals Of Python
Introduction
In this article, we will learn some fundaments of Python like identifiers, reserved words, comments, multiline statement, quotation in Python, multiple statements in single line and suites etc.
Python identifiers
Python identifiers are the smaller elements of Python program. We can say, the identifiers are used to identify a variable, module, class, function and other object.
Rules for identifiers are.
- Python identifiers are stared with the alphabet (a-z) or (A -Z) or underscore ( _ ).
- Python identifiers are followed by any alphabet (a-z) or (A-Z), number (0-9) and underscore(_).
- Python identifiers does not start with the digit (number (0-9)) and any special characters (@, % and $ etc.)
- Python identifiers does not have any special characters (@, % and $ etc.)
Example for identifiers are.
Identifiers name | Correct or incorrect |
_name | Correct |
name_ | Correct |
_name1 | Correct |
2name | Incorrect |
name_lastname_1 | Correct |
@name | Incorrect |
name@ | Incorrect |
Name | Correct |
Name1 | Correct |
Python is a case sensitive language. For example, in Python, CSHAPCORNER and csharpcorner; both are different identifiers, shown in the image, given below.
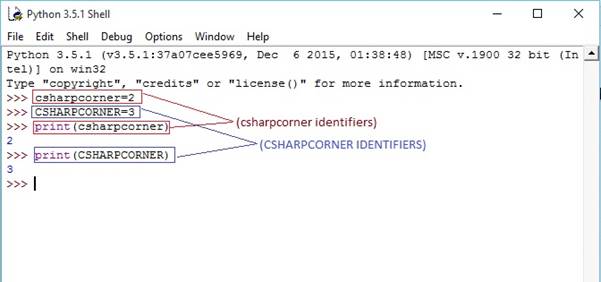
Names for identifiers in Python are.
In Python, the class name should start with the upper letter.
- Starting with underscore means identifiers are private.
Python reserved word (key word)
Python keywords (reserved words) are the words, which are reserved by Python and we cannot use as a variable and a constraint.
Python keywords are listed below.
Python reserved words | |||
False | True | from | if |
None | def | global | import |
And | del | raise | in |
As | elif | return | is |
Assert | else | try | lambda |
Break | except | while | nonlocal |
Class | finally | with | not |
Continue | for | yield |
or
|
Pass |
Comments in Python
Python also supports the comments like other programming languages. All the characters and strings should come after hash (#) sign and before finishing physical line. Python does not support multiline comment.
Example
- # This is the Python comment.
- print("this is c#corner Python tutorial by ajay malik").
- # This is Python Workspace.
Output
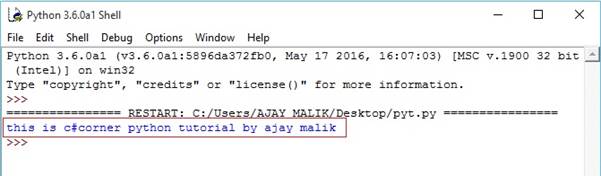
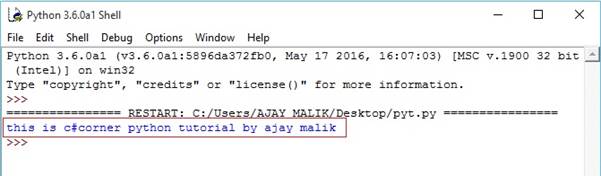
In the example, shown above, comment is ignored by Python.
Line and Indentation
In Python programming language, no braces are used to indicate the block of the function, class and code. It is indicated by line indentation.
For example.
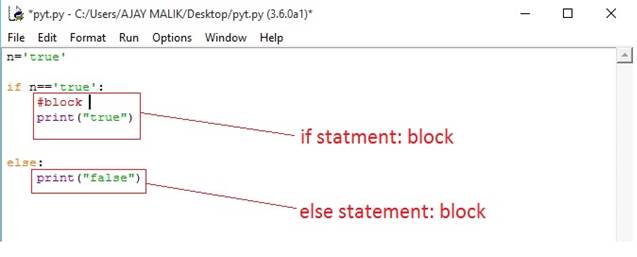
Quotation in Python
Python supports single (‘), double (“) and triple (‘’’ or “””) quotes to denote a string.
Triple (‘’’ or “””) is used to span the string in the multiple lines.
For example
- name='Python'
- type="programming language"
- content="""Python is open source programming language
- free to download and easy to use"""
Summary
In this article, you learnt about the fundaments of Python like keywords, identifiers and comments etc.