Tkinter Widgets-Menu
In Python, menu is generally used to provide a more than one option. We can create a menu form of popup and dropdown etc.
Syntax-
w = Menu ( main, option)
w = Menu ( main, option)
Parameter-
Main- parent Window.
Option- attribute of button widget.
Example-
Output
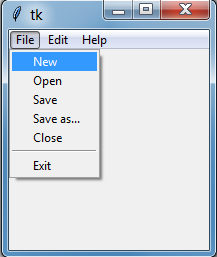
Example-
- from tkinter import *
- def none():
- filewin = Toplevel(main)
- button = Button(filewin, text="none")
- button.pack()
- main = Tk()
- menubar = Menu(main)
- filemenu = Menu(menubar, tearoff=0)
- filemenu.add_command(label="New", command=none)
- filemenu.add_command(label="Open", command=none)
- filemenu.add_command(label="Save", command=none)
- filemenu.add_command(label="Save as...", command=none)
- filemenu.add_command(label="Close", command=none)
- filemenu.add_separator()
- filemenu.add_command(label="Exit", command=main.quit)
- menubar.add_cascade(label="File", menu=filemenu)
- editmenu = Menu(menubar, tearoff=0)
- editmenu.add_command(label="Undo", command=none)
- editmenu.add_separator()
- editmenu.add_command(label="Cut", command=none)
- editmenu.add_command(label="Copy", command=none)
- editmenu.add_command(label="Paste", command=none)
- editmenu.add_command(label="Delete", command=none)
- editmenu.add_command(label="Select All", command=none)
- menubar.add_cascade(label="Edit", menu=editmenu)
- helpmenu = Menu(menubar, tearoff=0)
- helpmenu.add_command(label="Help Index", command=none)
- helpmenu.add_command(label="About...", command=none)
- menubar.add_cascade(label="Help", menu=helpmenu)
- main.config(menu=menubar)
- main.mainloop()
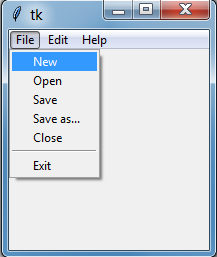
Option | Description |
activebackground | Sets background color of widgets, when the button is under the cursor. |
activeborderwidth | It is used to define the border width. |
activeforeground | It sets foreground color of widgets, when the button is under the cursor. |
bg | Normal background color. |
bd | It sets border width in pixels. Default is 1. |
cursor | Cursor is used to create a cursor in canvas like arrow, circle, dot etc. |
disabledforeground | It is used to disable foreground color of the text. |
font | It is used to set the font of the text. |
fg | It Setus normal foreground (text) color of widgets. |
postcommand | It is used to set the postcommand of widgets. |
relief | Relief is special type of border. The values are SUNKEN, RAISED, GROOVE, and RIDGE. |
image | It is used to set an image on widgets. |
selectcolor | It is used to set color of the selected item. |
Summary
In this chapter, you learnt what menu widget is and how to use the property of the widget.